mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
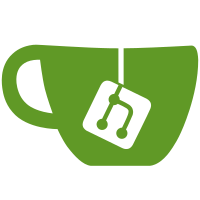
* take baseurl if saved on event * refactor: make es mocks reusable * Revert "refactor: make es mocks reusable" This reverts commit434ce12a6a
. * make messages testable * test asset url * fmt * fmt * simplify notification.Start * test url combinations * support init code added * support password changed * support reset pw * support user domain claimed * support add pwless login * support verify phone * Revert "support verify phone" This reverts commite40503303e
. * save trigger origin from ctx * add ready for review check * camel * test email otp * fix variable naming * fix DefaultOTPEmailURLV2 * Revert "fix DefaultOTPEmailURLV2" This reverts commitfa34d4d2a8
. * fix email otp challenged test * fix email otp challenged test * pass origin in login and gateway requests * take origin from header * take x-forwarded if present * Update internal/notification/handlers/queries.go Co-authored-by: Tim Möhlmann <tim+github@zitadel.com> * Update internal/notification/handlers/commands.go Co-authored-by: Tim Möhlmann <tim+github@zitadel.com> * move origin header to ctx if available * generate * cleanup * use forwarded header * support X-Forwarded-* headers * standardize context handling * fix linting --------- Co-authored-by: Tim Möhlmann <tim+github@zitadel.com>
68 lines
2.8 KiB
Go
68 lines
2.8 KiB
Go
package handlers
|
|
|
|
import (
|
|
"context"
|
|
"net/http"
|
|
|
|
"golang.org/x/text/language"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/query"
|
|
)
|
|
|
|
type Queries interface {
|
|
ActiveLabelPolicyByOrg(ctx context.Context, orgID string, withOwnerRemoved bool) (*query.LabelPolicy, error)
|
|
MailTemplateByOrg(ctx context.Context, orgID string, withOwnerRemoved bool) (*query.MailTemplate, error)
|
|
GetNotifyUserByID(ctx context.Context, shouldTriggered bool, userID string, withOwnerRemoved bool, queries ...query.SearchQuery) (*query.NotifyUser, error)
|
|
CustomTextListByTemplate(ctx context.Context, aggregateID, template string, withOwnerRemoved bool) (*query.CustomTexts, error)
|
|
SearchInstanceDomains(ctx context.Context, queries *query.InstanceDomainSearchQueries) (*query.InstanceDomains, error)
|
|
SessionByID(ctx context.Context, shouldTriggerBulk bool, id, sessionToken string) (*query.Session, error)
|
|
NotificationPolicyByOrg(ctx context.Context, shouldTriggerBulk bool, orgID string, withOwnerRemoved bool) (*query.NotificationPolicy, error)
|
|
SearchMilestones(ctx context.Context, instanceIDs []string, queries *query.MilestonesSearchQueries) (*query.Milestones, error)
|
|
NotificationProviderByIDAndType(ctx context.Context, aggID string, providerType domain.NotificationProviderType) (*query.DebugNotificationProvider, error)
|
|
SMSProviderConfig(ctx context.Context, queries ...query.SearchQuery) (*query.SMSConfig, error)
|
|
SMTPConfigByAggregateID(ctx context.Context, aggregateID string) (*query.SMTPConfig, error)
|
|
GetDefaultLanguage(ctx context.Context) language.Tag
|
|
}
|
|
|
|
type NotificationQueries struct {
|
|
Queries
|
|
es *eventstore.Eventstore
|
|
externalDomain string
|
|
externalPort uint16
|
|
externalSecure bool
|
|
fileSystemPath string
|
|
UserDataCrypto crypto.EncryptionAlgorithm
|
|
SMTPPasswordCrypto crypto.EncryptionAlgorithm
|
|
SMSTokenCrypto crypto.EncryptionAlgorithm
|
|
statikDir http.FileSystem
|
|
}
|
|
|
|
func NewNotificationQueries(
|
|
baseQueries Queries,
|
|
es *eventstore.Eventstore,
|
|
externalDomain string,
|
|
externalPort uint16,
|
|
externalSecure bool,
|
|
fileSystemPath string,
|
|
userDataCrypto crypto.EncryptionAlgorithm,
|
|
smtpPasswordCrypto crypto.EncryptionAlgorithm,
|
|
smsTokenCrypto crypto.EncryptionAlgorithm,
|
|
statikDir http.FileSystem,
|
|
) *NotificationQueries {
|
|
return &NotificationQueries{
|
|
Queries: baseQueries,
|
|
es: es,
|
|
externalDomain: externalDomain,
|
|
externalPort: externalPort,
|
|
externalSecure: externalSecure,
|
|
fileSystemPath: fileSystemPath,
|
|
UserDataCrypto: userDataCrypto,
|
|
SMTPPasswordCrypto: smtpPasswordCrypto,
|
|
SMSTokenCrypto: smsTokenCrypto,
|
|
statikDir: statikDir,
|
|
}
|
|
}
|