mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
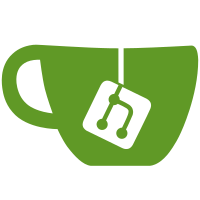
* fix: make user creation errors helpful * fix linting and unit testing errors * fix linting * make zitadel config reusable * fix human validations * translate ssr errors * make zitadel config reusable * cover more translations for ssr * handle email validation message centrally * fix unit tests * fix linting * align signatures * use more precise wording * handle phone validation message centrally * fix: return specific profile errors * docs: edit comments * fix unit tests --------- Co-authored-by: Silvan <silvan.reusser@gmail.com>
37 lines
822 B
Go
37 lines
822 B
Go
package idp
|
|
|
|
import (
|
|
"context"
|
|
|
|
"golang.org/x/text/language"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
)
|
|
|
|
// Provider is the minimal implementation for a 3rd party authentication provider
|
|
type Provider interface {
|
|
Name() string
|
|
BeginAuth(ctx context.Context, state string, params ...any) (Session, error)
|
|
IsLinkingAllowed() bool
|
|
IsCreationAllowed() bool
|
|
IsAutoCreation() bool
|
|
IsAutoUpdate() bool
|
|
}
|
|
|
|
// User contains the information of a federated user.
|
|
type User interface {
|
|
GetID() string
|
|
GetFirstName() string
|
|
GetLastName() string
|
|
GetDisplayName() string
|
|
GetNickname() string
|
|
GetPreferredUsername() string
|
|
GetEmail() domain.EmailAddress
|
|
IsEmailVerified() bool
|
|
GetPhone() domain.PhoneNumber
|
|
IsPhoneVerified() bool
|
|
GetPreferredLanguage() language.Tag
|
|
GetAvatarURL() string
|
|
GetProfile() string
|
|
}
|