mirror of
https://github.com/zitadel/zitadel.git
synced 2025-05-16 23:38:37 +00:00
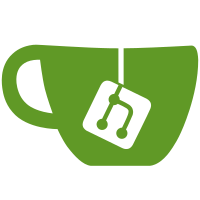
* new console * move npm ci to angular build * rel path for assets * local grpc copy * login policy, rm clear views, features rel path * login_hint param * edit default color values * remove features, zitadel-tier, contact styles * html formatter * rm feature restrictions, fix theming issues * instance naming * statehandler * rm class * rm class * update footer links * statehandler * stroked color Co-authored-by: Livio Amstutz <livio.a@gmail.com>
141 lines
4.3 KiB
TypeScript
141 lines
4.3 KiB
TypeScript
import { NgModule } from '@angular/core';
|
|
import { RouterModule, Routes } from '@angular/router';
|
|
import { AuthGuard } from 'src/app/guards/auth.guard';
|
|
import { RoleGuard } from 'src/app/guards/role.guard';
|
|
import { PolicyComponentServiceType, PolicyComponentType } from 'src/app/modules/policies/policy-component-types.enum';
|
|
|
|
import { IamComponent } from './iam.component';
|
|
|
|
const routes: Routes = [
|
|
{
|
|
path: '',
|
|
component: IamComponent,
|
|
canActivate: [AuthGuard, RoleGuard],
|
|
data: {
|
|
roles: ['iam.read'],
|
|
},
|
|
},
|
|
{
|
|
path: 'members',
|
|
loadChildren: () => import('./iam-members/iam-members.module').then((m) => m.IamMembersModule),
|
|
canActivate: [AuthGuard, RoleGuard],
|
|
data: {
|
|
roles: ['iam.member.read'],
|
|
},
|
|
},
|
|
{
|
|
path: 'idp',
|
|
children: [
|
|
{
|
|
path: 'create',
|
|
loadChildren: () => import('src/app/modules/idp-create/idp-create.module').then((m) => m.IdpCreateModule),
|
|
canActivate: [AuthGuard, RoleGuard],
|
|
data: {
|
|
roles: ['iam.idp.write'],
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
},
|
|
{
|
|
path: ':id',
|
|
loadChildren: () => import('src/app/modules/idp/idp.module').then((m) => m.IdpModule),
|
|
canActivate: [AuthGuard, RoleGuard],
|
|
data: {
|
|
roles: ['iam.idp.read'],
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
},
|
|
],
|
|
},
|
|
{
|
|
path: 'policy',
|
|
children: [
|
|
{
|
|
path: PolicyComponentType.AGE,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/password-age-policy/password-age-policy.module').then(
|
|
(m) => m.PasswordAgePolicyModule,
|
|
),
|
|
},
|
|
{
|
|
path: PolicyComponentType.LOCKOUT,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/password-lockout-policy/password-lockout-policy.module').then(
|
|
(m) => m.PasswordLockoutPolicyModule,
|
|
),
|
|
},
|
|
{
|
|
path: PolicyComponentType.PRIVATELABEL,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/private-labeling-policy/private-labeling-policy.module').then(
|
|
(m) => m.PrivateLabelingPolicyModule,
|
|
),
|
|
},
|
|
{
|
|
path: PolicyComponentType.COMPLEXITY,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/password-complexity-policy/password-complexity-policy.module').then(
|
|
(m) => m.PasswordComplexityPolicyModule,
|
|
),
|
|
},
|
|
{
|
|
path: PolicyComponentType.IAM,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/org-iam-policy/org-iam-policy.module').then((m) => m.OrgIamPolicyModule),
|
|
},
|
|
{
|
|
path: PolicyComponentType.LOGIN,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/login-policy/login-policy.module').then((m) => m.LoginPolicyModule),
|
|
},
|
|
{
|
|
path: PolicyComponentType.MESSAGETEXTS,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/message-texts/message-texts.module').then((m) => m.MessageTextsPolicyModule),
|
|
},
|
|
{
|
|
path: PolicyComponentType.LOGINTEXTS,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/login-texts/login-texts.module').then((m) => m.LoginTextsPolicyModule),
|
|
},
|
|
{
|
|
path: PolicyComponentType.PRIVACYPOLICY,
|
|
data: {
|
|
serviceType: PolicyComponentServiceType.ADMIN,
|
|
},
|
|
loadChildren: () =>
|
|
import('src/app/modules/policies/privacy-policy/privacy-policy.module').then((m) => m.PrivacyPolicyModule),
|
|
},
|
|
],
|
|
},
|
|
];
|
|
|
|
@NgModule({
|
|
imports: [RouterModule.forChild(routes)],
|
|
exports: [RouterModule],
|
|
})
|
|
export class IamRoutingModule {}
|