mirror of
https://github.com/zitadel/zitadel.git
synced 2025-07-01 11:18:33 +00:00
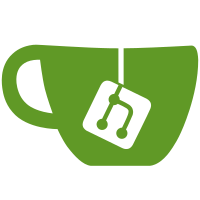
* commander * commander * selber! * move to packages * fix(errors): implement Is interface * test: command * test: commands * add init steps * setup tenant * add default step yaml * possibility to set password * merge v2 into v2-commander * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: search query builder can filter events in memory * fix: filters for add member * fix(setup): add `ExternalSecure` to config * chore: name iam to instance * fix: matching * remove unsued func * base url * base url * test(command): filter funcs * test: commands * fix: rename orgiampolicy to domain policy * start from init * commands * config * fix indexes and add constraints * fixes * fix: merge conflicts * fix: protos * fix: md files * setup * add deprecated org iam policy again * typo * fix search query * fix filter * Apply suggestions from code review * remove custom org from org setup * add todos for verification * change apps creation * simplify package structure * fix error * move preparation helper for tests * fix unique constraints * fix config mapping in setup * fix error handling in encryption_keys.go * fix projection config * fix query from old views to projection * fix setup of mgmt api * set iam project and fix instance projection * fix tokens view * fix steps.yaml and defaults.yaml * fix projections * change instance context to interface * instance interceptors and additional events in setup * cleanup * tests for interceptors * fix label policy * add todo * single api endpoint in environment.json Co-authored-by: adlerhurst <silvan.reusser@gmail.com> Co-authored-by: fabi <fabienne.gerschwiler@gmail.com>
60 lines
1.3 KiB
Go
60 lines
1.3 KiB
Go
package models
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/caos/zitadel/internal/api/authz"
|
|
)
|
|
|
|
type AggregateCreator struct {
|
|
serviceName string
|
|
}
|
|
|
|
func NewAggregateCreator(serviceName string) *AggregateCreator {
|
|
return &AggregateCreator{serviceName: serviceName}
|
|
}
|
|
|
|
type option func(*Aggregate)
|
|
|
|
func (c *AggregateCreator) NewAggregate(ctx context.Context, id string, typ AggregateType, version Version, previousSequence uint64, opts ...option) (*Aggregate, error) {
|
|
ctxData := authz.GetCtxData(ctx)
|
|
instance := authz.GetInstance(ctx)
|
|
editorUser := ctxData.UserID
|
|
resourceOwner := ctxData.OrgID
|
|
instanceID := instance.InstanceID()
|
|
|
|
aggregate := &Aggregate{
|
|
ID: id,
|
|
typ: typ,
|
|
PreviousSequence: previousSequence,
|
|
version: version,
|
|
Events: make([]*Event, 0, 2),
|
|
editorService: c.serviceName,
|
|
editorUser: editorUser,
|
|
resourceOwner: resourceOwner,
|
|
instanceID: instanceID,
|
|
}
|
|
|
|
for _, opt := range opts {
|
|
opt(aggregate)
|
|
}
|
|
|
|
if err := aggregate.Validate(); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return aggregate, nil
|
|
}
|
|
|
|
func OverwriteEditorUser(userID string) func(*Aggregate) {
|
|
return func(a *Aggregate) {
|
|
a.editorUser = userID
|
|
}
|
|
}
|
|
|
|
func OverwriteResourceOwner(resourceOwner string) func(*Aggregate) {
|
|
return func(a *Aggregate) {
|
|
a.resourceOwner = resourceOwner
|
|
}
|
|
}
|