mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 02:54:20 +00:00
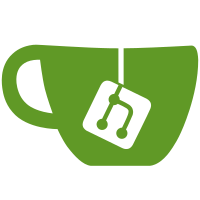
* chore: rename package errors to zerrors * rename package errors to gerrors * fix error related linting issues * fix zitadel error assertion * fix gosimple linting issues * fix deprecated linting issues * resolve gci linting issues * fix import structure --------- Co-authored-by: Elio Bischof <elio@zitadel.com>
61 lines
1.3 KiB
Go
61 lines
1.3 KiB
Go
package domain
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
es_models "github.com/zitadel/zitadel/internal/eventstore/v1/models"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
type Password struct {
|
|
es_models.ObjectRoot
|
|
|
|
SecretString string
|
|
EncodedSecret string
|
|
ChangeRequired bool
|
|
}
|
|
|
|
func NewPassword(password string) *Password {
|
|
return &Password{
|
|
SecretString: password,
|
|
}
|
|
}
|
|
|
|
type PasswordCode struct {
|
|
es_models.ObjectRoot
|
|
|
|
Code *crypto.CryptoValue
|
|
Expiry time.Duration
|
|
NotificationType NotificationType
|
|
}
|
|
|
|
func (p *Password) HashPasswordIfExisting(policy *PasswordComplexityPolicy, hasher *crypto.PasswordHasher) error {
|
|
if p.SecretString == "" {
|
|
return nil
|
|
}
|
|
if policy == nil {
|
|
return zerrors.ThrowPreconditionFailed(nil, "DOMAIN-s8ifS", "Errors.User.PasswordComplexityPolicy.NotFound")
|
|
}
|
|
if err := policy.Check(p.SecretString); err != nil {
|
|
return err
|
|
}
|
|
encoded, err := hasher.Hash(p.SecretString)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
p.EncodedSecret = encoded
|
|
return nil
|
|
}
|
|
|
|
func NewPasswordCode(passwordGenerator crypto.Generator) (*PasswordCode, error) {
|
|
passwordCodeCrypto, _, err := crypto.NewCode(passwordGenerator)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &PasswordCode{
|
|
Code: passwordCodeCrypto,
|
|
Expiry: passwordGenerator.Expiry(),
|
|
}, nil
|
|
}
|