mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
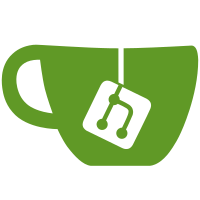
* implement notification providers * email provider * notification handler * notify users * implement code sent on user eventstore * send email implementation * send init code * handle codes * fix project member handler * add some logs for debug * send emails * text changes * send sms * notification process * send password code * format phone number * test format phone * remove fmts * remove unused code * rename files * add mocks * merge master * Update internal/notification/providers/email/message.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * Update internal/notification/repository/eventsourcing/handler/notification.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * Update internal/notification/repository/eventsourcing/handler/notification.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * Update internal/notification/providers/email/provider.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * requested changes of mr * move locker to eventstore pkg * Update internal/notification/providers/chat/message.go Co-authored-by: Livio Amstutz <livio.a@gmail.com> * move locker to eventstore pkg * linebreak * Update internal/notification/providers/email/provider.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * Update internal/notification/repository/eventsourcing/handler/notification.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * Update internal/notification/repository/eventsourcing/handler/notification.go Co-authored-by: Silvan <silvan.reusser@gmail.com> Co-authored-by: Silvan <silvan.reusser@gmail.com> Co-authored-by: Livio Amstutz <livio.a@gmail.com>
40 lines
1.2 KiB
Go
40 lines
1.2 KiB
Go
package twilio
|
|
|
|
import (
|
|
"github.com/caos/logging"
|
|
caos_errs "github.com/caos/zitadel/internal/errors"
|
|
"github.com/caos/zitadel/internal/notification/providers"
|
|
twilio "github.com/kevinburke/twilio-go"
|
|
)
|
|
|
|
type Twilio struct {
|
|
client *twilio.Client
|
|
}
|
|
|
|
func InitTwilioProvider(config TwilioConfig) *Twilio {
|
|
return &Twilio{
|
|
client: twilio.NewClient(config.SID, config.Token, nil),
|
|
}
|
|
}
|
|
|
|
func (t *Twilio) CanHandleMessage(message providers.Message) bool {
|
|
twilioMsg, ok := message.(*TwilioMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return twilioMsg.Content != "" && twilioMsg.RecipientPhoneNumber != "" && twilioMsg.SenderPhoneNumber != ""
|
|
}
|
|
|
|
func (t *Twilio) HandleMessage(message providers.Message) error {
|
|
twilioMsg, ok := message.(*TwilioMessage)
|
|
if !ok {
|
|
return caos_errs.ThrowInternal(nil, "TWILI-s0pLc", "message is not TwilioMessage")
|
|
}
|
|
m, err := t.client.Messages.SendMessage(twilioMsg.SenderPhoneNumber, twilioMsg.RecipientPhoneNumber, twilioMsg.GetContent(), nil)
|
|
if err != nil {
|
|
return caos_errs.ThrowInternal(err, "TWILI-osk3S", "could not send message")
|
|
}
|
|
logging.LogWithFields("SMS_-f335c523", "message_sid", m.Sid, "status", m.Status).Debug("sms sent")
|
|
return nil
|
|
}
|