mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
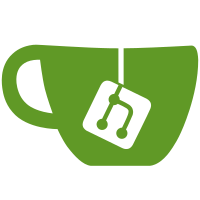
* refactor(domain): add user type * fix(projections): start with login names * fix(login_policy): correct handling of user domain claimed event * fix(projections): add members * refactor: simplify member projections * add migration for members * add metadata to member projections * refactor: login name projection * fix: set correct suffixes on login name projections * test(projections): login name reduces * fix: correct cols in reduce member * test(projections): org, iam, project members * member additional cols and conds as opt, add project grant members * fix(migration): members * fix(migration): correct database name * migration version * migs * better naming for member cond and col * split project and project grant members * prepare member columns * feat(queries): membership query * test(queries): membership prepare * fix(queries): multiple projections for latest sequence * fix(api): use query for membership queries in auth and management * feat: org member queries * fix(api): use query for iam member calls * fix(queries): org members * fix(queries): project members * fix(queries): project grant members * fix(query): member queries and user avatar column * member cols * fix(queries): membership stmt * fix user test * fix user test * fix(projections): add user grant projection * fix(user_grant): handle state changes * add state to migration * merge eventstore-naming into user-grant-projection * fix(migrations): version * fix(query): event mappers for usergrant aggregate * fix(projection): correct aggregate for user grants * cleanup reducers * add tests for projection Co-authored-by: Livio Amstutz <livio.a@gmail.com>
103 lines
3.3 KiB
Go
103 lines
3.3 KiB
Go
package query
|
|
|
|
import (
|
|
"context"
|
|
"database/sql"
|
|
"net/http"
|
|
"sync"
|
|
|
|
"github.com/caos/logging"
|
|
sd "github.com/caos/zitadel/internal/config/systemdefaults"
|
|
"github.com/caos/zitadel/internal/config/types"
|
|
"github.com/caos/zitadel/internal/eventstore"
|
|
iam_model "github.com/caos/zitadel/internal/iam/model"
|
|
"github.com/caos/zitadel/internal/query/projection"
|
|
"github.com/caos/zitadel/internal/repository/action"
|
|
iam_repo "github.com/caos/zitadel/internal/repository/iam"
|
|
"github.com/caos/zitadel/internal/repository/keypair"
|
|
"github.com/caos/zitadel/internal/repository/org"
|
|
"github.com/caos/zitadel/internal/repository/project"
|
|
usr_repo "github.com/caos/zitadel/internal/repository/user"
|
|
"github.com/caos/zitadel/internal/repository/usergrant"
|
|
"github.com/caos/zitadel/internal/telemetry/tracing"
|
|
"github.com/rakyll/statik/fs"
|
|
"golang.org/x/text/language"
|
|
)
|
|
|
|
type Queries struct {
|
|
iamID string
|
|
eventstore *eventstore.Eventstore
|
|
client *sql.DB
|
|
|
|
DefaultLanguage language.Tag
|
|
LoginDir http.FileSystem
|
|
NotificationDir http.FileSystem
|
|
mutex sync.Mutex
|
|
LoginTranslationFileContents map[string][]byte
|
|
NotificationTranslationFileContents map[string][]byte
|
|
}
|
|
|
|
type Config struct {
|
|
Eventstore types.SQLUser
|
|
}
|
|
|
|
func StartQueries(ctx context.Context, es *eventstore.Eventstore, projections projection.Config, defaults sd.SystemDefaults, keyChan chan<- interface{}) (repo *Queries, err error) {
|
|
sqlClient, err := projections.CRDB.Start()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
statikLoginFS, err := fs.NewWithNamespace("login")
|
|
logging.Log("CONFI-7usEW").OnError(err).Panic("unable to start login statik dir")
|
|
|
|
statikNotificationFS, err := fs.NewWithNamespace("notification")
|
|
logging.Log("CONFI-7usEW").OnError(err).Panic("unable to start notification statik dir")
|
|
|
|
repo = &Queries{
|
|
iamID: defaults.IamID,
|
|
eventstore: es,
|
|
client: sqlClient,
|
|
DefaultLanguage: defaults.DefaultLanguage,
|
|
LoginDir: statikLoginFS,
|
|
NotificationDir: statikNotificationFS,
|
|
LoginTranslationFileContents: make(map[string][]byte),
|
|
NotificationTranslationFileContents: make(map[string][]byte),
|
|
}
|
|
iam_repo.RegisterEventMappers(repo.eventstore)
|
|
usr_repo.RegisterEventMappers(repo.eventstore)
|
|
org.RegisterEventMappers(repo.eventstore)
|
|
project.RegisterEventMappers(repo.eventstore)
|
|
action.RegisterEventMappers(repo.eventstore)
|
|
keypair.RegisterEventMappers(repo.eventstore)
|
|
usergrant.RegisterEventMappers(repo.eventstore)
|
|
|
|
err = projection.Start(ctx, sqlClient, es, projections, defaults, keyChan)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return repo, nil
|
|
}
|
|
|
|
func (r *Queries) IAMByID(ctx context.Context, id string) (_ *iam_model.IAM, err error) {
|
|
readModel, err := r.iamByID(ctx, id)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return readModelToIAM(readModel), nil
|
|
}
|
|
|
|
func (r *Queries) iamByID(ctx context.Context, id string) (_ *ReadModel, err error) {
|
|
ctx, span := tracing.NewSpan(ctx)
|
|
defer func() { span.EndWithError(err) }()
|
|
|
|
readModel := NewReadModel(id)
|
|
err = r.eventstore.FilterToQueryReducer(ctx, readModel)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return readModel, nil
|
|
}
|