mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
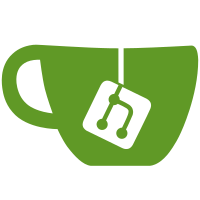
* start sub * start implement subsciptions * start subscription * implementation for member done * admin done * fix: tests * extend handlers * prepary notification * no errors in adminapi * changed current sequence in all packages * ignore mocks * works * subscriptions as singleton * tests * refactor: rename function scope var * fix: process ALL previous sequences * fix: spooler and pubsub * handler check * fix: process events until all done * fix break on query err * fix: handler * fix: process sequence or return error * check aggregate id * fix: log only in error case * fix tests * fix: handlers * fix: spooler * fix: spooler * fix: tests * fix: continue * fix: locker duration * fix: variable lock duration * fix: test * fix: test * fix: test min max time Co-authored-by: Livio Amstutz <livio.a@gmail.com>
105 lines
3.2 KiB
Go
105 lines
3.2 KiB
Go
package handler
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/caos/zitadel/internal/admin/repository/eventsourcing/view"
|
|
"github.com/caos/zitadel/internal/config/systemdefaults"
|
|
"github.com/caos/zitadel/internal/config/types"
|
|
"github.com/caos/zitadel/internal/eventstore"
|
|
"github.com/caos/zitadel/internal/eventstore/query"
|
|
iam_event "github.com/caos/zitadel/internal/iam/repository/eventsourcing"
|
|
org_event "github.com/caos/zitadel/internal/org/repository/eventsourcing"
|
|
usr_event "github.com/caos/zitadel/internal/user/repository/eventsourcing"
|
|
)
|
|
|
|
type Configs map[string]*Config
|
|
|
|
type Config struct {
|
|
MinimumCycleDuration types.Duration
|
|
}
|
|
|
|
type handler struct {
|
|
view *view.View
|
|
bulkLimit uint64
|
|
cycleDuration time.Duration
|
|
errorCountUntilSkip uint64
|
|
|
|
es eventstore.Eventstore
|
|
}
|
|
|
|
func (h *handler) Eventstore() eventstore.Eventstore {
|
|
return h.es
|
|
}
|
|
|
|
type EventstoreRepos struct {
|
|
UserEvents *usr_event.UserEventstore
|
|
IamEvents *iam_event.IAMEventstore
|
|
OrgEvents *org_event.OrgEventstore
|
|
}
|
|
|
|
func Register(configs Configs, bulkLimit, errorCount uint64, view *view.View, es eventstore.Eventstore, repos EventstoreRepos, defaults systemdefaults.SystemDefaults) []query.Handler {
|
|
return []query.Handler{
|
|
newOrg(
|
|
handler{view, bulkLimit, configs.cycleDuration("Org"), errorCount, es}),
|
|
newIAMMember(
|
|
handler{view, bulkLimit, configs.cycleDuration("IamMember"), errorCount, es},
|
|
repos.UserEvents),
|
|
newIDPConfig(
|
|
handler{view, bulkLimit, configs.cycleDuration("IDPConfig"), errorCount, es}),
|
|
newLabelPolicy(
|
|
handler{view, bulkLimit, configs.cycleDuration("LabelPolicy"), errorCount, es}),
|
|
newLoginPolicy(
|
|
handler{view, bulkLimit, configs.cycleDuration("LoginPolicy"), errorCount, es}),
|
|
newIDPProvider(
|
|
handler{view, bulkLimit, configs.cycleDuration("IDPProvider"), errorCount, es},
|
|
defaults,
|
|
repos.IamEvents,
|
|
repos.OrgEvents),
|
|
newUser(
|
|
handler{view, bulkLimit, configs.cycleDuration("User"), errorCount, es},
|
|
repos.OrgEvents,
|
|
repos.IamEvents,
|
|
defaults),
|
|
newPasswordComplexityPolicy(
|
|
handler{view, bulkLimit, configs.cycleDuration("PasswordComplexityPolicy"), errorCount, es}),
|
|
newPasswordAgePolicy(
|
|
handler{view, bulkLimit, configs.cycleDuration("PasswordAgePolicy"), errorCount, es}),
|
|
newPasswordLockoutPolicy(
|
|
handler{view, bulkLimit, configs.cycleDuration("PasswordLockoutPolicy"), errorCount, es}),
|
|
newOrgIAMPolicy(
|
|
handler{view, bulkLimit, configs.cycleDuration("OrgIAMPolicy"), errorCount, es}),
|
|
newExternalIDP(
|
|
handler{view, bulkLimit, configs.cycleDuration("ExternalIDP"), errorCount, es},
|
|
defaults,
|
|
repos.IamEvents,
|
|
repos.OrgEvents),
|
|
}
|
|
}
|
|
|
|
func subscribe(es eventstore.Eventstore, handlers []query.Handler) {
|
|
for _, handler := range handlers {
|
|
es.Subscribe(handler.AggregateTypes()...)
|
|
}
|
|
}
|
|
|
|
func (configs Configs) cycleDuration(viewModel string) time.Duration {
|
|
c, ok := configs[viewModel]
|
|
if !ok {
|
|
return 3 * time.Minute
|
|
}
|
|
return c.MinimumCycleDuration.Duration
|
|
}
|
|
|
|
func (h *handler) MinimumCycleDuration() time.Duration {
|
|
return h.cycleDuration
|
|
}
|
|
|
|
func (h *handler) LockDuration() time.Duration {
|
|
return h.cycleDuration / 3
|
|
}
|
|
|
|
func (h *handler) QueryLimit() uint64 {
|
|
return h.bulkLimit
|
|
}
|