mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
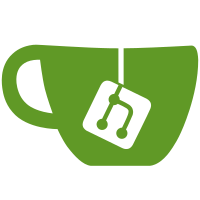
* feat(crypto): use passwap for machine and app secrets * fix command package tests * add hash generator command test * naming convention, fix query tests * rename PasswordHasher and cleanup start commands * add reducer tests * fix intergration tests, cleanup old config * add app secret unit tests * solve setup panics * fix push of updated events * add missing event translations * update documentation * solve linter errors * remove nolint:SA1019 as it doesn't seem to help anyway * add nolint to deprecated filter usage * update users migration version * remove unused ClientSecret from APIConfigChangedEvent --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
62 lines
1.2 KiB
Go
62 lines
1.2 KiB
Go
package domain
|
|
|
|
import (
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/eventstore/v1/models"
|
|
)
|
|
|
|
type APIApp struct {
|
|
models.ObjectRoot
|
|
|
|
AppID string
|
|
AppName string
|
|
ClientID string
|
|
EncodedHash string
|
|
ClientSecretString string
|
|
AuthMethodType APIAuthMethodType
|
|
|
|
State AppState
|
|
}
|
|
|
|
func (a *APIApp) GetApplicationName() string {
|
|
return a.AppName
|
|
}
|
|
|
|
func (a *APIApp) GetState() AppState {
|
|
return a.State
|
|
}
|
|
|
|
type APIAuthMethodType int32
|
|
|
|
const (
|
|
APIAuthMethodTypeBasic APIAuthMethodType = iota
|
|
APIAuthMethodTypePrivateKeyJWT
|
|
)
|
|
|
|
func (a *APIApp) IsValid() bool {
|
|
return a.AppName != ""
|
|
}
|
|
|
|
func (a *APIApp) setClientID(clientID string) {
|
|
a.ClientID = clientID
|
|
}
|
|
|
|
func (a *APIApp) setClientSecret(encodedHash string) {
|
|
a.EncodedHash = encodedHash
|
|
}
|
|
|
|
func (a *APIApp) requiresClientSecret() bool {
|
|
return a.AuthMethodType == APIAuthMethodTypeBasic
|
|
}
|
|
|
|
func (a *APIApp) GenerateClientSecretIfNeeded(generator *crypto.HashGenerator) (plain string, err error) {
|
|
if a.AuthMethodType == APIAuthMethodTypePrivateKeyJWT {
|
|
return "", nil
|
|
}
|
|
a.EncodedHash, plain, err = generator.NewCode()
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
return plain, nil
|
|
}
|