mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-16 12:58:00 +00:00
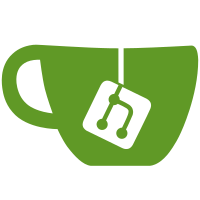
# Which Problems Are Solved UserSchema API is currently not completely as defined for v3alpha. # How the Problems Are Solved Update the protos and integration tests. # Additional Changes None # Additional Context None
318 lines
8.9 KiB
Go
318 lines
8.9 KiB
Go
//go:build integration
|
|
|
|
package userschema_test
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/brianvoe/gofakeit/v6"
|
|
"github.com/muhlemmer/gu"
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
"google.golang.org/protobuf/types/known/structpb"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/grpc"
|
|
"github.com/zitadel/zitadel/internal/integration"
|
|
object "github.com/zitadel/zitadel/pkg/grpc/resources/object/v3alpha"
|
|
schema "github.com/zitadel/zitadel/pkg/grpc/resources/userschema/v3alpha"
|
|
)
|
|
|
|
func TestServer_ListUserSchemas(t *testing.T) {
|
|
ensureFeatureEnabled(t, IAMOwnerCTX)
|
|
|
|
userSchema := new(structpb.Struct)
|
|
err := userSchema.UnmarshalJSON([]byte(`{
|
|
"$schema": "urn:zitadel:schema:v1",
|
|
"type": "object",
|
|
"properties": {}
|
|
}`))
|
|
require.NoError(t, err)
|
|
type args struct {
|
|
ctx context.Context
|
|
req *schema.SearchUserSchemasRequest
|
|
prepare func(request *schema.SearchUserSchemasRequest, resp *schema.SearchUserSchemasResponse) error
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want *schema.SearchUserSchemasResponse
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "missing permission",
|
|
args: args{
|
|
ctx: Tester.WithAuthorization(context.Background(), integration.OrgOwner),
|
|
req: &schema.SearchUserSchemasRequest{},
|
|
},
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "not found, error",
|
|
args: args{
|
|
ctx: IAMOwnerCTX,
|
|
req: &schema.SearchUserSchemasRequest{
|
|
Filters: []*schema.SearchFilter{
|
|
{
|
|
Filter: &schema.SearchFilter_IdFilter{
|
|
IdFilter: &schema.IDFilter{
|
|
Id: "notexisting",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
want: &schema.SearchUserSchemasResponse{
|
|
Details: &object.ListDetails{
|
|
TotalResult: 0,
|
|
AppliedLimit: 100,
|
|
},
|
|
Result: []*schema.GetUserSchema{},
|
|
},
|
|
},
|
|
{
|
|
name: "single (id), ok",
|
|
args: args{
|
|
ctx: IAMOwnerCTX,
|
|
req: &schema.SearchUserSchemasRequest{},
|
|
prepare: func(request *schema.SearchUserSchemasRequest, resp *schema.SearchUserSchemasResponse) error {
|
|
schemaType := gofakeit.Name()
|
|
createResp := Tester.CreateUserSchemaEmptyWithType(IAMOwnerCTX, schemaType)
|
|
request.Filters = []*schema.SearchFilter{
|
|
{
|
|
Filter: &schema.SearchFilter_IdFilter{
|
|
IdFilter: &schema.IDFilter{
|
|
Id: createResp.GetDetails().GetId(),
|
|
Method: object.TextFilterMethod_TEXT_FILTER_METHOD_EQUALS,
|
|
},
|
|
},
|
|
},
|
|
}
|
|
resp.Result[0].Config.Type = schemaType
|
|
resp.Result[0].Details = createResp.GetDetails()
|
|
// as schema is freshly created, the changed date is the created date
|
|
resp.Result[0].Details.Created = resp.Result[0].Details.GetChanged()
|
|
resp.Details.Timestamp = resp.Result[0].Details.GetChanged()
|
|
return nil
|
|
},
|
|
},
|
|
want: &schema.SearchUserSchemasResponse{
|
|
Details: &object.ListDetails{
|
|
TotalResult: 1,
|
|
AppliedLimit: 100,
|
|
},
|
|
Result: []*schema.GetUserSchema{
|
|
{
|
|
State: schema.State_STATE_ACTIVE,
|
|
Revision: 1,
|
|
Config: &schema.UserSchema{
|
|
Type: "",
|
|
DataType: &schema.UserSchema_Schema{
|
|
Schema: userSchema,
|
|
},
|
|
PossibleAuthenticators: nil,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "multiple (type), ok",
|
|
args: args{
|
|
ctx: IAMOwnerCTX,
|
|
req: &schema.SearchUserSchemasRequest{},
|
|
prepare: func(request *schema.SearchUserSchemasRequest, resp *schema.SearchUserSchemasResponse) error {
|
|
schemaType := gofakeit.Name()
|
|
schemaType1 := schemaType + "_1"
|
|
schemaType2 := schemaType + "_2"
|
|
createResp := Tester.CreateUserSchemaEmptyWithType(IAMOwnerCTX, schemaType1)
|
|
createResp2 := Tester.CreateUserSchemaEmptyWithType(IAMOwnerCTX, schemaType2)
|
|
|
|
request.SortingColumn = gu.Ptr(schema.FieldName_FIELD_NAME_TYPE)
|
|
request.Query = &object.SearchQuery{Desc: false}
|
|
request.Filters = []*schema.SearchFilter{
|
|
{
|
|
Filter: &schema.SearchFilter_TypeFilter{
|
|
TypeFilter: &schema.TypeFilter{
|
|
Type: schemaType,
|
|
Method: object.TextFilterMethod_TEXT_FILTER_METHOD_STARTS_WITH,
|
|
},
|
|
},
|
|
},
|
|
}
|
|
|
|
resp.Result[0].Config.Type = schemaType1
|
|
resp.Result[0].Details = createResp.GetDetails()
|
|
resp.Result[1].Config.Type = schemaType2
|
|
resp.Result[1].Details = createResp2.GetDetails()
|
|
return nil
|
|
},
|
|
},
|
|
want: &schema.SearchUserSchemasResponse{
|
|
Details: &object.ListDetails{
|
|
TotalResult: 2,
|
|
AppliedLimit: 100,
|
|
},
|
|
Result: []*schema.GetUserSchema{
|
|
{
|
|
State: schema.State_STATE_ACTIVE,
|
|
Revision: 1,
|
|
Config: &schema.UserSchema{
|
|
DataType: &schema.UserSchema_Schema{
|
|
Schema: userSchema,
|
|
},
|
|
},
|
|
},
|
|
{
|
|
State: schema.State_STATE_ACTIVE,
|
|
Revision: 1,
|
|
Config: &schema.UserSchema{
|
|
DataType: &schema.UserSchema_Schema{
|
|
Schema: userSchema,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
if tt.args.prepare != nil {
|
|
err := tt.args.prepare(tt.args.req, tt.want)
|
|
require.NoError(t, err)
|
|
}
|
|
|
|
retryDuration := 20 * time.Second
|
|
if ctxDeadline, ok := IAMOwnerCTX.Deadline(); ok {
|
|
retryDuration = time.Until(ctxDeadline)
|
|
}
|
|
|
|
require.EventuallyWithT(t, func(ttt *assert.CollectT) {
|
|
got, err := Client.SearchUserSchemas(tt.args.ctx, tt.args.req)
|
|
if tt.wantErr {
|
|
require.Error(ttt, err)
|
|
return
|
|
}
|
|
assert.NoError(ttt, err)
|
|
|
|
// always first check length, otherwise its failed anyway
|
|
assert.Len(ttt, got.Result, len(tt.want.Result))
|
|
for i := range tt.want.Result {
|
|
want := tt.want.Result[i]
|
|
got := got.Result[i]
|
|
|
|
integration.AssertResourceDetails(t, want.GetDetails(), got.GetDetails())
|
|
want.Details = got.Details
|
|
grpc.AllFieldsEqual(t, want.ProtoReflect(), got.ProtoReflect(), grpc.CustomMappers)
|
|
}
|
|
integration.AssertListDetails(t, tt.want, got)
|
|
}, retryDuration, time.Millisecond*100, "timeout waiting for expected user schema result")
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestServer_GetUserSchema(t *testing.T) {
|
|
ensureFeatureEnabled(t, IAMOwnerCTX)
|
|
|
|
userSchema := new(structpb.Struct)
|
|
err := userSchema.UnmarshalJSON([]byte(`{
|
|
"$schema": "urn:zitadel:schema:v1",
|
|
"type": "object",
|
|
"properties": {}
|
|
}`))
|
|
require.NoError(t, err)
|
|
type args struct {
|
|
ctx context.Context
|
|
req *schema.GetUserSchemaRequest
|
|
prepare func(request *schema.GetUserSchemaRequest, resp *schema.GetUserSchemaResponse) error
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want *schema.GetUserSchemaResponse
|
|
wantErr bool
|
|
}{
|
|
{
|
|
name: "missing permission",
|
|
args: args{
|
|
ctx: Tester.WithAuthorization(context.Background(), integration.OrgOwner),
|
|
req: &schema.GetUserSchemaRequest{},
|
|
prepare: func(request *schema.GetUserSchemaRequest, resp *schema.GetUserSchemaResponse) error {
|
|
schemaType := gofakeit.Name()
|
|
createResp := Tester.CreateUserSchemaEmptyWithType(IAMOwnerCTX, schemaType)
|
|
request.Id = createResp.GetDetails().GetId()
|
|
return nil
|
|
},
|
|
},
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "not existing, error",
|
|
args: args{
|
|
ctx: IAMOwnerCTX,
|
|
req: &schema.GetUserSchemaRequest{
|
|
Id: "notexisting",
|
|
},
|
|
},
|
|
wantErr: true,
|
|
},
|
|
{
|
|
name: "get, ok",
|
|
args: args{
|
|
ctx: IAMOwnerCTX,
|
|
req: &schema.GetUserSchemaRequest{},
|
|
prepare: func(request *schema.GetUserSchemaRequest, resp *schema.GetUserSchemaResponse) error {
|
|
schemaType := gofakeit.Name()
|
|
createResp := Tester.CreateUserSchemaEmptyWithType(IAMOwnerCTX, schemaType)
|
|
request.Id = createResp.GetDetails().GetId()
|
|
|
|
resp.UserSchema.Config.Type = schemaType
|
|
resp.UserSchema.Details = createResp.GetDetails()
|
|
return nil
|
|
},
|
|
},
|
|
want: &schema.GetUserSchemaResponse{
|
|
UserSchema: &schema.GetUserSchema{
|
|
State: schema.State_STATE_ACTIVE,
|
|
Revision: 1,
|
|
Config: &schema.UserSchema{
|
|
DataType: &schema.UserSchema_Schema{
|
|
Schema: userSchema,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
if tt.args.prepare != nil {
|
|
err := tt.args.prepare(tt.args.req, tt.want)
|
|
require.NoError(t, err)
|
|
}
|
|
|
|
retryDuration := 5 * time.Second
|
|
if ctxDeadline, ok := IAMOwnerCTX.Deadline(); ok {
|
|
retryDuration = time.Until(ctxDeadline)
|
|
}
|
|
|
|
require.EventuallyWithT(t, func(ttt *assert.CollectT) {
|
|
got, err := Client.GetUserSchema(tt.args.ctx, tt.args.req)
|
|
if tt.wantErr {
|
|
assert.Error(t, err, "Error: "+err.Error())
|
|
} else {
|
|
assert.NoError(t, err)
|
|
wantSchema := tt.want.GetUserSchema()
|
|
gotSchema := got.GetUserSchema()
|
|
integration.AssertResourceDetails(t, wantSchema.GetDetails(), gotSchema.GetDetails())
|
|
tt.want.UserSchema.Details = got.GetUserSchema().GetDetails()
|
|
grpc.AllFieldsEqual(t, tt.want.ProtoReflect(), got.ProtoReflect(), grpc.CustomMappers)
|
|
}
|
|
}, retryDuration, time.Millisecond*100, "timeout waiting for expected user schema result")
|
|
})
|
|
}
|
|
}
|