mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
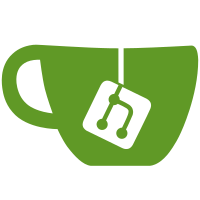
This implementation increases parallel write capabilities of the eventstore. Please have a look at the technical advisories: [05](https://zitadel.com/docs/support/advisory/a10005) and [06](https://zitadel.com/docs/support/advisory/a10006). The implementation of eventstore.push is rewritten and stored events are migrated to a new table `eventstore.events2`. If you are using cockroach: make sure that the database user of ZITADEL has `VIEWACTIVITY` grant. This is used to query events.
60 lines
1.5 KiB
Go
60 lines
1.5 KiB
Go
package handler
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/admin/repository/eventsourcing/view"
|
|
"github.com/zitadel/zitadel/internal/database"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
handler2 "github.com/zitadel/zitadel/internal/eventstore/handler/v2"
|
|
"github.com/zitadel/zitadel/internal/static"
|
|
)
|
|
|
|
type Config struct {
|
|
Client *database.DB
|
|
Eventstore *eventstore.Eventstore
|
|
|
|
BulkLimit uint64
|
|
FailureCountUntilSkip uint64
|
|
HandleActiveInstances time.Duration
|
|
TransactionDuration time.Duration
|
|
Handlers map[string]*ConfigOverwrites
|
|
}
|
|
|
|
type ConfigOverwrites struct {
|
|
MinimumCycleDuration time.Duration
|
|
}
|
|
|
|
func Register(ctx context.Context, config Config, view *view.View, static static.Storage) {
|
|
if static == nil {
|
|
return
|
|
}
|
|
|
|
newStyling(ctx,
|
|
config.overwrite("Styling"),
|
|
static,
|
|
view,
|
|
).Start(ctx)
|
|
}
|
|
|
|
func (config Config) overwrite(viewModel string) handler2.Config {
|
|
c := handler2.Config{
|
|
Client: config.Client,
|
|
Eventstore: config.Eventstore,
|
|
BulkLimit: uint16(config.BulkLimit),
|
|
RequeueEvery: 3 * time.Minute,
|
|
HandleActiveInstances: config.HandleActiveInstances,
|
|
MaxFailureCount: uint8(config.FailureCountUntilSkip),
|
|
TransactionDuration: config.TransactionDuration,
|
|
}
|
|
overwrite, ok := config.Handlers[viewModel]
|
|
if !ok {
|
|
return c
|
|
}
|
|
if overwrite.MinimumCycleDuration > 0 {
|
|
c.RequeueEvery = overwrite.MinimumCycleDuration
|
|
}
|
|
return c
|
|
}
|