mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
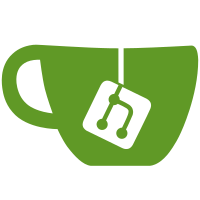
* fix: add prompt on oidc rp * fix: add prompt on oidc rp * fix: translation * fix: translation * fix: not existing login policy * fix: login policy * fix: identity provider detail * fix: idp update * fix: idps in login policy * fix: lint * fix: scss * fix: external idps on auth user detail * fix: idp create mapping fields * fix: remove idp provider * fix: angular lint * fix: login policy view * fix: translations
3845 lines
93 KiB
Go
3845 lines
93 KiB
Go
// Code generated by protoc-gen-validate. DO NOT EDIT.
|
|
// source: auth.proto
|
|
|
|
package auth
|
|
|
|
import (
|
|
"bytes"
|
|
"errors"
|
|
"fmt"
|
|
"net"
|
|
"net/mail"
|
|
"net/url"
|
|
"regexp"
|
|
"strings"
|
|
"time"
|
|
"unicode/utf8"
|
|
|
|
"github.com/golang/protobuf/ptypes"
|
|
)
|
|
|
|
// ensure the imports are used
|
|
var (
|
|
_ = bytes.MinRead
|
|
_ = errors.New("")
|
|
_ = fmt.Print
|
|
_ = utf8.UTFMax
|
|
_ = (*regexp.Regexp)(nil)
|
|
_ = (*strings.Reader)(nil)
|
|
_ = net.IPv4len
|
|
_ = time.Duration(0)
|
|
_ = (*url.URL)(nil)
|
|
_ = (*mail.Address)(nil)
|
|
_ = ptypes.DynamicAny{}
|
|
)
|
|
|
|
// define the regex for a UUID once up-front
|
|
var _auth_uuidPattern = regexp.MustCompile("^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$")
|
|
|
|
// Validate checks the field values on UserSessionViews with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserSessionViews) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetUserSessions() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserSessionViewsValidationError{
|
|
field: fmt.Sprintf("UserSessions[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserSessionViewsValidationError is the validation error returned by
|
|
// UserSessionViews.Validate if the designated constraints aren't met.
|
|
type UserSessionViewsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserSessionViewsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserSessionViewsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserSessionViewsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserSessionViewsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserSessionViewsValidationError) ErrorName() string { return "UserSessionViewsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserSessionViewsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserSessionViews.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserSessionViewsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserSessionViewsValidationError{}
|
|
|
|
// Validate checks the field values on UserSessionView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserSessionView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for AgentId
|
|
|
|
// no validation rules for AuthState
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for UserName
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for LoginName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserSessionViewValidationError is the validation error returned by
|
|
// UserSessionView.Validate if the designated constraints aren't met.
|
|
type UserSessionViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserSessionViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserSessionViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserSessionViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserSessionViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserSessionViewValidationError) ErrorName() string { return "UserSessionViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserSessionViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserSessionView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserSessionViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserSessionViewValidationError{}
|
|
|
|
// Validate checks the field values on UserView with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for PreferredLoginName
|
|
|
|
if v, ok := interface{}(m.GetLastLogin()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "LastLogin",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for ResourceOwner
|
|
|
|
// no validation rules for UserName
|
|
|
|
switch m.User.(type) {
|
|
|
|
case *UserView_Human:
|
|
|
|
if v, ok := interface{}(m.GetHuman()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "Human",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
case *UserView_Machine:
|
|
|
|
if v, ok := interface{}(m.GetMachine()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "Machine",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
default:
|
|
return UserViewValidationError{
|
|
field: "User",
|
|
reason: "value is required",
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserViewValidationError is the validation error returned by
|
|
// UserView.Validate if the designated constraints aren't met.
|
|
type UserViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserViewValidationError) ErrorName() string { return "UserViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserViewValidationError{}
|
|
|
|
// Validate checks the field values on MachineView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MachineView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetLastKeyAdded()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineViewValidationError{
|
|
field: "LastKeyAdded",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Description
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineViewValidationError is the validation error returned by
|
|
// MachineView.Validate if the designated constraints aren't met.
|
|
type MachineViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineViewValidationError) ErrorName() string { return "MachineViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineViewValidationError{}
|
|
|
|
// Validate checks the field values on MachineKeyView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MachineKeyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Type
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetExpirationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeyViewValidationError{
|
|
field: "ExpirationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineKeyViewValidationError is the validation error returned by
|
|
// MachineKeyView.Validate if the designated constraints aren't met.
|
|
type MachineKeyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineKeyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineKeyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineKeyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineKeyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineKeyViewValidationError) ErrorName() string { return "MachineKeyViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineKeyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineKeyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineKeyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineKeyViewValidationError{}
|
|
|
|
// Validate checks the field values on HumanView with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *HumanView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetPasswordChanged()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return HumanViewValidationError{
|
|
field: "PasswordChanged",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
return nil
|
|
}
|
|
|
|
// HumanViewValidationError is the validation error returned by
|
|
// HumanView.Validate if the designated constraints aren't met.
|
|
type HumanViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e HumanViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e HumanViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e HumanViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e HumanViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e HumanViewValidationError) ErrorName() string { return "HumanViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e HumanViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sHumanView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = HumanViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = HumanViewValidationError{}
|
|
|
|
// Validate checks the field values on UserProfile with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserProfile) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserProfileValidationError is the validation error returned by
|
|
// UserProfile.Validate if the designated constraints aren't met.
|
|
type UserProfileValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserProfileValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserProfileValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserProfileValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserProfileValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserProfileValidationError) ErrorName() string { return "UserProfileValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserProfileValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserProfile.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserProfileValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserProfileValidationError{}
|
|
|
|
// Validate checks the field values on UserProfileView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserProfileView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for PreferredLoginName
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserProfileViewValidationError is the validation error returned by
|
|
// UserProfileView.Validate if the designated constraints aren't met.
|
|
type UserProfileViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserProfileViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserProfileViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserProfileViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserProfileViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserProfileViewValidationError) ErrorName() string { return "UserProfileViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserProfileViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserProfileView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserProfileViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserProfileViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserProfileRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserProfileRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetFirstName()); l < 1 || l > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "FirstName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetLastName()); l < 1 || l > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "LastName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetNickName()) > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "NickName",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetPreferredLanguage()); l < 1 || l > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "PreferredLanguage",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Gender
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserProfileRequestValidationError is the validation error returned by
|
|
// UpdateUserProfileRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserProfileRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserProfileRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserProfileRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserProfileRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserProfileRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserProfileRequestValidationError) ErrorName() string {
|
|
return "UpdateUserProfileRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserProfileRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserProfileRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserProfileRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserProfileRequestValidationError{}
|
|
|
|
// Validate checks the field values on ChangeUserNameRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ChangeUserNameRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if !_ChangeUserNameRequest_UserName_Pattern.MatchString(m.GetUserName()) {
|
|
return ChangeUserNameRequestValidationError{
|
|
field: "UserName",
|
|
reason: "value does not match regex pattern \"^[^[:space:]]{1,200}$\"",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangeUserNameRequestValidationError is the validation error returned by
|
|
// ChangeUserNameRequest.Validate if the designated constraints aren't met.
|
|
type ChangeUserNameRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangeUserNameRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangeUserNameRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangeUserNameRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangeUserNameRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangeUserNameRequestValidationError) ErrorName() string {
|
|
return "ChangeUserNameRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangeUserNameRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChangeUserNameRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangeUserNameRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangeUserNameRequestValidationError{}
|
|
|
|
var _ChangeUserNameRequest_UserName_Pattern = regexp.MustCompile("^[^[:space:]]{1,200}$")
|
|
|
|
// Validate checks the field values on UserEmail with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserEmail) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserEmailValidationError is the validation error returned by
|
|
// UserEmail.Validate if the designated constraints aren't met.
|
|
type UserEmailValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserEmailValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserEmailValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserEmailValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserEmailValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserEmailValidationError) ErrorName() string { return "UserEmailValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserEmailValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserEmail.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserEmailValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserEmailValidationError{}
|
|
|
|
// Validate checks the field values on UserEmailView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserEmailView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserEmailViewValidationError is the validation error returned by
|
|
// UserEmailView.Validate if the designated constraints aren't met.
|
|
type UserEmailViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserEmailViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserEmailViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserEmailViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserEmailViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserEmailViewValidationError) ErrorName() string { return "UserEmailViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserEmailViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserEmailView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserEmailViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserEmailViewValidationError{}
|
|
|
|
// Validate checks the field values on VerifyMyUserEmailRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *VerifyMyUserEmailRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetCode()); l < 1 || l > 200 {
|
|
return VerifyMyUserEmailRequestValidationError{
|
|
field: "Code",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// VerifyMyUserEmailRequestValidationError is the validation error returned by
|
|
// VerifyMyUserEmailRequest.Validate if the designated constraints aren't met.
|
|
type VerifyMyUserEmailRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e VerifyMyUserEmailRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e VerifyMyUserEmailRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e VerifyMyUserEmailRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e VerifyMyUserEmailRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e VerifyMyUserEmailRequestValidationError) ErrorName() string {
|
|
return "VerifyMyUserEmailRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e VerifyMyUserEmailRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sVerifyMyUserEmailRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = VerifyMyUserEmailRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = VerifyMyUserEmailRequestValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserEmailRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserEmailRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetEmail()); l < 1 || l > 200 {
|
|
return UpdateUserEmailRequestValidationError{
|
|
field: "Email",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserEmailRequestValidationError is the validation error returned by
|
|
// UpdateUserEmailRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserEmailRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserEmailRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserEmailRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserEmailRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserEmailRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserEmailRequestValidationError) ErrorName() string {
|
|
return "UpdateUserEmailRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserEmailRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserEmailRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserEmailRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserEmailRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserPhone with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserPhone) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserPhoneValidationError is the validation error returned by
|
|
// UserPhone.Validate if the designated constraints aren't met.
|
|
type UserPhoneValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserPhoneValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserPhoneValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserPhoneValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserPhoneValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserPhoneValidationError) ErrorName() string { return "UserPhoneValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserPhoneValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserPhone.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserPhoneValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserPhoneValidationError{}
|
|
|
|
// Validate checks the field values on UserPhoneView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserPhoneView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserPhoneViewValidationError is the validation error returned by
|
|
// UserPhoneView.Validate if the designated constraints aren't met.
|
|
type UserPhoneViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserPhoneViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserPhoneViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserPhoneViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserPhoneViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserPhoneViewValidationError) ErrorName() string { return "UserPhoneViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserPhoneViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserPhoneView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserPhoneViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserPhoneViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserPhoneRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserPhoneRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetPhone()); l < 1 || l > 20 {
|
|
return UpdateUserPhoneRequestValidationError{
|
|
field: "Phone",
|
|
reason: "value length must be between 1 and 20 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserPhoneRequestValidationError is the validation error returned by
|
|
// UpdateUserPhoneRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserPhoneRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserPhoneRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserPhoneRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserPhoneRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserPhoneRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserPhoneRequestValidationError) ErrorName() string {
|
|
return "UpdateUserPhoneRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserPhoneRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserPhoneRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserPhoneRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserPhoneRequestValidationError{}
|
|
|
|
// Validate checks the field values on VerifyUserPhoneRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *VerifyUserPhoneRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetCode()); l < 1 || l > 200 {
|
|
return VerifyUserPhoneRequestValidationError{
|
|
field: "Code",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// VerifyUserPhoneRequestValidationError is the validation error returned by
|
|
// VerifyUserPhoneRequest.Validate if the designated constraints aren't met.
|
|
type VerifyUserPhoneRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e VerifyUserPhoneRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e VerifyUserPhoneRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e VerifyUserPhoneRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e VerifyUserPhoneRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e VerifyUserPhoneRequestValidationError) ErrorName() string {
|
|
return "VerifyUserPhoneRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e VerifyUserPhoneRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sVerifyUserPhoneRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = VerifyUserPhoneRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = VerifyUserPhoneRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserAddress with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserAddress) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserAddressValidationError is the validation error returned by
|
|
// UserAddress.Validate if the designated constraints aren't met.
|
|
type UserAddressValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserAddressValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserAddressValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserAddressValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserAddressValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserAddressValidationError) ErrorName() string { return "UserAddressValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserAddressValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserAddress.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserAddressValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserAddressValidationError{}
|
|
|
|
// Validate checks the field values on UserAddressView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserAddressView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserAddressViewValidationError is the validation error returned by
|
|
// UserAddressView.Validate if the designated constraints aren't met.
|
|
type UserAddressViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserAddressViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserAddressViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserAddressViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserAddressViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserAddressViewValidationError) ErrorName() string { return "UserAddressViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserAddressViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserAddressView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserAddressViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserAddressViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserAddressRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserAddressRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetCountry()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "Country",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetLocality()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "Locality",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPostalCode()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "PostalCode",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetRegion()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "Region",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetStreetAddress()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "StreetAddress",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserAddressRequestValidationError is the validation error returned by
|
|
// UpdateUserAddressRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserAddressRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserAddressRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserAddressRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserAddressRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserAddressRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserAddressRequestValidationError) ErrorName() string {
|
|
return "UpdateUserAddressRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserAddressRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserAddressRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserAddressRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserAddressRequestValidationError{}
|
|
|
|
// Validate checks the field values on PasswordChange with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *PasswordChange) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetOldPassword()); l < 1 || l > 72 {
|
|
return PasswordChangeValidationError{
|
|
field: "OldPassword",
|
|
reason: "value length must be between 1 and 72 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetNewPassword()); l < 1 || l > 72 {
|
|
return PasswordChangeValidationError{
|
|
field: "NewPassword",
|
|
reason: "value length must be between 1 and 72 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordChangeValidationError is the validation error returned by
|
|
// PasswordChange.Validate if the designated constraints aren't met.
|
|
type PasswordChangeValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordChangeValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordChangeValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordChangeValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordChangeValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordChangeValidationError) ErrorName() string { return "PasswordChangeValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordChangeValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordChange.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordChangeValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordChangeValidationError{}
|
|
|
|
// Validate checks the field values on VerifyMfaOtp with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *VerifyMfaOtp) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetCode()) < 1 {
|
|
return VerifyMfaOtpValidationError{
|
|
field: "Code",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// VerifyMfaOtpValidationError is the validation error returned by
|
|
// VerifyMfaOtp.Validate if the designated constraints aren't met.
|
|
type VerifyMfaOtpValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e VerifyMfaOtpValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e VerifyMfaOtpValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e VerifyMfaOtpValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e VerifyMfaOtpValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e VerifyMfaOtpValidationError) ErrorName() string { return "VerifyMfaOtpValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e VerifyMfaOtpValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sVerifyMfaOtp.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = VerifyMfaOtpValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = VerifyMfaOtpValidationError{}
|
|
|
|
// Validate checks the field values on MultiFactors with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MultiFactors) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetMfas() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MultiFactorsValidationError{
|
|
field: fmt.Sprintf("Mfas[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MultiFactorsValidationError is the validation error returned by
|
|
// MultiFactors.Validate if the designated constraints aren't met.
|
|
type MultiFactorsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MultiFactorsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MultiFactorsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MultiFactorsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MultiFactorsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MultiFactorsValidationError) ErrorName() string { return "MultiFactorsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MultiFactorsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMultiFactors.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MultiFactorsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MultiFactorsValidationError{}
|
|
|
|
// Validate checks the field values on MultiFactor with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MultiFactor) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Type
|
|
|
|
// no validation rules for State
|
|
|
|
return nil
|
|
}
|
|
|
|
// MultiFactorValidationError is the validation error returned by
|
|
// MultiFactor.Validate if the designated constraints aren't met.
|
|
type MultiFactorValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MultiFactorValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MultiFactorValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MultiFactorValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MultiFactorValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MultiFactorValidationError) ErrorName() string { return "MultiFactorValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MultiFactorValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMultiFactor.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MultiFactorValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MultiFactorValidationError{}
|
|
|
|
// Validate checks the field values on MfaOtpResponse with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MfaOtpResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for Url
|
|
|
|
// no validation rules for Secret
|
|
|
|
// no validation rules for State
|
|
|
|
return nil
|
|
}
|
|
|
|
// MfaOtpResponseValidationError is the validation error returned by
|
|
// MfaOtpResponse.Validate if the designated constraints aren't met.
|
|
type MfaOtpResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MfaOtpResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MfaOtpResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MfaOtpResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MfaOtpResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MfaOtpResponseValidationError) ErrorName() string { return "MfaOtpResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MfaOtpResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMfaOtpResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MfaOtpResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MfaOtpResponseValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserGrantSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
if _, ok := _UserGrantSearchRequest_SortingColumn_NotInLookup[m.GetSortingColumn()]; ok {
|
|
return UserGrantSearchRequestValidationError{
|
|
field: "SortingColumn",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Asc
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantSearchRequestValidationError is the validation error returned by
|
|
// UserGrantSearchRequest.Validate if the designated constraints aren't met.
|
|
type UserGrantSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantSearchRequestValidationError) ErrorName() string {
|
|
return "UserGrantSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantSearchRequestValidationError{}
|
|
|
|
var _UserGrantSearchRequest_SortingColumn_NotInLookup = map[UserGrantSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on UserGrantSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserGrantSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _UserGrantSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return UserGrantSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantSearchQueryValidationError is the validation error returned by
|
|
// UserGrantSearchQuery.Validate if the designated constraints aren't met.
|
|
type UserGrantSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantSearchQueryValidationError) ErrorName() string {
|
|
return "UserGrantSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantSearchQueryValidationError{}
|
|
|
|
var _UserGrantSearchQuery_Key_NotInLookup = map[UserGrantSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on UserGrantSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserGrantSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantSearchResponseValidationError is the validation error returned by
|
|
// UserGrantSearchResponse.Validate if the designated constraints aren't met.
|
|
type UserGrantSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantSearchResponseValidationError) ErrorName() string {
|
|
return "UserGrantSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserGrantView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for OrgId
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for OrgName
|
|
|
|
// no validation rules for GrantId
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantViewValidationError is the validation error returned by
|
|
// UserGrantView.Validate if the designated constraints aren't met.
|
|
type UserGrantViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantViewValidationError) ErrorName() string { return "UserGrantViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantViewValidationError{}
|
|
|
|
// Validate checks the field values on MyProjectOrgSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MyProjectOrgSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for Asc
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MyProjectOrgSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MyProjectOrgSearchRequestValidationError is the validation error returned by
|
|
// MyProjectOrgSearchRequest.Validate if the designated constraints aren't met.
|
|
type MyProjectOrgSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MyProjectOrgSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MyProjectOrgSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MyProjectOrgSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MyProjectOrgSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MyProjectOrgSearchRequestValidationError) ErrorName() string {
|
|
return "MyProjectOrgSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MyProjectOrgSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMyProjectOrgSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MyProjectOrgSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MyProjectOrgSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on MyProjectOrgSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MyProjectOrgSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _MyProjectOrgSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return MyProjectOrgSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// MyProjectOrgSearchQueryValidationError is the validation error returned by
|
|
// MyProjectOrgSearchQuery.Validate if the designated constraints aren't met.
|
|
type MyProjectOrgSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MyProjectOrgSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MyProjectOrgSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MyProjectOrgSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MyProjectOrgSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MyProjectOrgSearchQueryValidationError) ErrorName() string {
|
|
return "MyProjectOrgSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MyProjectOrgSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMyProjectOrgSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MyProjectOrgSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MyProjectOrgSearchQueryValidationError{}
|
|
|
|
var _MyProjectOrgSearchQuery_Key_NotInLookup = map[MyProjectOrgSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on MyProjectOrgSearchResponse with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MyProjectOrgSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MyProjectOrgSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MyProjectOrgSearchResponseValidationError is the validation error returned
|
|
// by MyProjectOrgSearchResponse.Validate if the designated constraints aren't met.
|
|
type MyProjectOrgSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MyProjectOrgSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MyProjectOrgSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MyProjectOrgSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MyProjectOrgSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MyProjectOrgSearchResponseValidationError) ErrorName() string {
|
|
return "MyProjectOrgSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MyProjectOrgSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMyProjectOrgSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MyProjectOrgSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MyProjectOrgSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on Org with the rules defined in the proto
|
|
// definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Org) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Name
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgValidationError is the validation error returned by Org.Validate if the
|
|
// designated constraints aren't met.
|
|
type OrgValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgValidationError) ErrorName() string { return "OrgValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrg.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgValidationError{}
|
|
|
|
// Validate checks the field values on MyPermissions with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MyPermissions) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MyPermissionsValidationError is the validation error returned by
|
|
// MyPermissions.Validate if the designated constraints aren't met.
|
|
type MyPermissionsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MyPermissionsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MyPermissionsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MyPermissionsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MyPermissionsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MyPermissionsValidationError) ErrorName() string { return "MyPermissionsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MyPermissionsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMyPermissions.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MyPermissionsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MyPermissionsValidationError{}
|
|
|
|
// Validate checks the field values on ChangesRequest with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ChangesRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for SequenceOffset
|
|
|
|
// no validation rules for Asc
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangesRequestValidationError is the validation error returned by
|
|
// ChangesRequest.Validate if the designated constraints aren't met.
|
|
type ChangesRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangesRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangesRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangesRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangesRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangesRequestValidationError) ErrorName() string { return "ChangesRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangesRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChangesRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangesRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangesRequestValidationError{}
|
|
|
|
// Validate checks the field values on Changes with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Changes) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetChanges() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangesValidationError{
|
|
field: fmt.Sprintf("Changes[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangesValidationError is the validation error returned by Changes.Validate
|
|
// if the designated constraints aren't met.
|
|
type ChangesValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangesValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangesValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangesValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangesValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangesValidationError) ErrorName() string { return "ChangesValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangesValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChanges.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangesValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangesValidationError{}
|
|
|
|
// Validate checks the field values on Change with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Change) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangeValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetEventType()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangeValidationError{
|
|
field: "EventType",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for EditorId
|
|
|
|
// no validation rules for Editor
|
|
|
|
if v, ok := interface{}(m.GetData()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangeValidationError{
|
|
field: "Data",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangeValidationError is the validation error returned by Change.Validate if
|
|
// the designated constraints aren't met.
|
|
type ChangeValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangeValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangeValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangeValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangeValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangeValidationError) ErrorName() string { return "ChangeValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangeValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChange.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangeValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangeValidationError{}
|
|
|
|
// Validate checks the field values on PasswordComplexityPolicy with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordComplexityPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Description
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordComplexityPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordComplexityPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for MinLength
|
|
|
|
// no validation rules for HasLowercase
|
|
|
|
// no validation rules for HasUppercase
|
|
|
|
// no validation rules for HasNumber
|
|
|
|
// no validation rules for HasSymbol
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for IsDefault
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordComplexityPolicyValidationError is the validation error returned by
|
|
// PasswordComplexityPolicy.Validate if the designated constraints aren't met.
|
|
type PasswordComplexityPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordComplexityPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordComplexityPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordComplexityPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordComplexityPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordComplexityPolicyValidationError) ErrorName() string {
|
|
return "PasswordComplexityPolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordComplexityPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordComplexityPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordComplexityPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordComplexityPolicyValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ExternalIDPResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPResponseValidationError is the validation error returned by
|
|
// ExternalIDPResponse.Validate if the designated constraints aren't met.
|
|
type ExternalIDPResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPResponseValidationError) ErrorName() string {
|
|
return "ExternalIDPResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPResponseValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPRemoveRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ExternalIDPRemoveRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for ExternalUserId
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPRemoveRequestValidationError is the validation error returned by
|
|
// ExternalIDPRemoveRequest.Validate if the designated constraints aren't met.
|
|
type ExternalIDPRemoveRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPRemoveRequestValidationError) ErrorName() string {
|
|
return "ExternalIDPRemoveRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPRemoveRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPRemoveRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPRemoveRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPRemoveRequestValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ExternalIDPSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPSearchRequestValidationError is the validation error returned by
|
|
// ExternalIDPSearchRequest.Validate if the designated constraints aren't met.
|
|
type ExternalIDPSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPSearchRequestValidationError) ErrorName() string {
|
|
return "ExternalIDPSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ExternalIDPSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPSearchResponseValidationError is the validation error returned by
|
|
// ExternalIDPSearchResponse.Validate if the designated constraints aren't met.
|
|
type ExternalIDPSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPSearchResponseValidationError) ErrorName() string {
|
|
return "ExternalIDPSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ExternalIDPView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for ExternalUserId
|
|
|
|
// no validation rules for IdpName
|
|
|
|
// no validation rules for ExternalUserDisplayName
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPViewValidationError is the validation error returned by
|
|
// ExternalIDPView.Validate if the designated constraints aren't met.
|
|
type ExternalIDPViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPViewValidationError) ErrorName() string { return "ExternalIDPViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPViewValidationError{}
|