mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
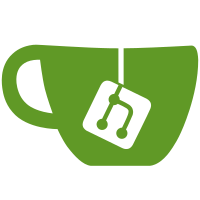
* add tracing interceptors to login and oidc * add some tracing spans * trace login calls * add some spans * add some spans (change password) * add some more tracing in oauth/oidc * revert org exists * Merge branch 'master' into http-tracing # Conflicts: # internal/api/oidc/auth_request.go # internal/api/oidc/client.go # internal/auth/repository/eventsourcing/eventstore/auth_request.go # internal/auth/repository/eventsourcing/eventstore/user.go # internal/authz/repository/eventsourcing/eventstore/token_verifier.go # internal/authz/repository/eventsourcing/view/token.go # internal/user/repository/eventsourcing/eventstore.go
82 lines
1.9 KiB
Go
82 lines
1.9 KiB
Go
package tracing
|
|
|
|
import (
|
|
"context"
|
|
"net/http"
|
|
|
|
"go.opencensus.io/trace"
|
|
)
|
|
|
|
type Tracer interface {
|
|
Start() error
|
|
NewSpan(ctx context.Context, caller string) (context.Context, *Span)
|
|
NewClientSpan(ctx context.Context, caller string) (context.Context, *Span)
|
|
NewServerSpan(ctx context.Context, caller string) (context.Context, *Span)
|
|
NewClientInterceptorSpan(ctx context.Context, name string) (context.Context, *Span)
|
|
NewServerInterceptorSpan(ctx context.Context, name string) (context.Context, *Span)
|
|
NewSpanHTTP(r *http.Request, caller string) (*http.Request, *Span)
|
|
Sampler() trace.Sampler
|
|
}
|
|
|
|
type Config interface {
|
|
NewTracer() error
|
|
}
|
|
|
|
var T Tracer
|
|
|
|
func Sampler() trace.Sampler {
|
|
if T == nil {
|
|
return trace.NeverSample()
|
|
}
|
|
return T.Sampler()
|
|
}
|
|
|
|
func NewSpan(ctx context.Context) (context.Context, *Span) {
|
|
if T == nil {
|
|
return ctx, CreateSpan(nil)
|
|
}
|
|
return T.NewSpan(ctx, GetCaller())
|
|
}
|
|
|
|
func NewNamedSpan(ctx context.Context, name string) (context.Context, *Span) {
|
|
if T == nil {
|
|
return ctx, CreateSpan(nil)
|
|
}
|
|
return T.NewSpan(ctx, name)
|
|
}
|
|
|
|
func NewClientSpan(ctx context.Context) (context.Context, *Span) {
|
|
if T == nil {
|
|
return ctx, CreateSpan(nil)
|
|
}
|
|
return T.NewClientSpan(ctx, GetCaller())
|
|
}
|
|
|
|
func NewServerSpan(ctx context.Context) (context.Context, *Span) {
|
|
if T == nil {
|
|
return ctx, CreateSpan(nil)
|
|
}
|
|
return T.NewServerSpan(ctx, GetCaller())
|
|
}
|
|
|
|
func NewClientInterceptorSpan(ctx context.Context) (context.Context, *Span) {
|
|
if T == nil {
|
|
return ctx, CreateSpan(nil)
|
|
}
|
|
return T.NewClientInterceptorSpan(ctx, GetCaller())
|
|
}
|
|
|
|
func NewServerInterceptorSpan(ctx context.Context) (context.Context, *Span) {
|
|
if T == nil {
|
|
return ctx, CreateSpan(nil)
|
|
}
|
|
return T.NewServerInterceptorSpan(ctx, GetCaller())
|
|
}
|
|
|
|
func NewSpanHTTP(r *http.Request) (*http.Request, *Span) {
|
|
if T == nil {
|
|
return r, CreateSpan(nil)
|
|
}
|
|
return T.NewSpanHTTP(r, GetCaller())
|
|
}
|