mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
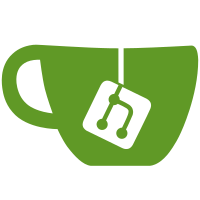
* docs: rewrite concept section * docs: add instance to guides * chore: error messages * fix: scenarios * docs: urls * docs: change images * docs: change images * docs: change images Co-authored-by: Livio Amstutz <livio.a@gmail.com>
59 lines
1.7 KiB
Go
59 lines
1.7 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
caos_errs "github.com/zitadel/zitadel/internal/errors"
|
|
)
|
|
|
|
func (c *Commands) ChangeIDPOIDCConfig(ctx context.Context, config *domain.OIDCIDPConfig, resourceOwner string) (*domain.OIDCIDPConfig, error) {
|
|
if resourceOwner == "" {
|
|
return nil, caos_errs.ThrowInvalidArgument(nil, "Org-4n8f2", "Errors.ResourceOwnerMissing")
|
|
}
|
|
if config.IDPConfigID == "" {
|
|
return nil, caos_errs.ThrowInvalidArgument(nil, "Org-66Qwj", "Errors.IDMissing")
|
|
}
|
|
existingConfig := NewOrgIDPOIDCConfigWriteModel(config.IDPConfigID, resourceOwner)
|
|
err := c.eventstore.FilterToQueryReducer(ctx, existingConfig)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if existingConfig.State == domain.IDPConfigStateRemoved || existingConfig.State == domain.IDPConfigStateUnspecified {
|
|
return nil, caos_errs.ThrowNotFound(nil, "Org-67J9d", "Errors.Org.IDPConfig.AlreadyExists")
|
|
}
|
|
|
|
orgAgg := OrgAggregateFromWriteModel(&existingConfig.WriteModel)
|
|
changedEvent, hasChanged, err := existingConfig.NewChangedEvent(
|
|
ctx,
|
|
orgAgg,
|
|
config.IDPConfigID,
|
|
config.ClientID,
|
|
config.Issuer,
|
|
config.AuthorizationEndpoint,
|
|
config.TokenEndpoint,
|
|
config.ClientSecretString,
|
|
c.idpConfigEncryption,
|
|
config.IDPDisplayNameMapping,
|
|
config.UsernameMapping,
|
|
config.Scopes...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if !hasChanged {
|
|
return nil, caos_errs.ThrowPreconditionFailed(nil, "Org-10ods", "Errors.Org.IDPConfig.NotChanged")
|
|
}
|
|
|
|
pushedEvents, err := c.eventstore.Push(ctx, changedEvent)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
err = AppendAndReduce(existingConfig, pushedEvents...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return writeModelToIDPOIDCConfig(&existingConfig.OIDCConfigWriteModel), nil
|
|
}
|