mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
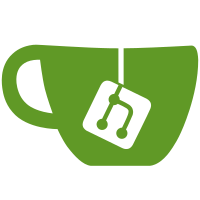
This implementation increases parallel write capabilities of the eventstore. Please have a look at the technical advisories: [05](https://zitadel.com/docs/support/advisory/a10005) and [06](https://zitadel.com/docs/support/advisory/a10006). The implementation of eventstore.push is rewritten and stored events are migrated to a new table `eventstore.events2`. If you are using cockroach: make sure that the database user of ZITADEL has `VIEWACTIVITY` grant. This is used to query events.
65 lines
1.4 KiB
Go
65 lines
1.4 KiB
Go
package eventstore
|
|
|
|
import (
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
)
|
|
|
|
var _ eventstore.Command = (*mockCommand)(nil)
|
|
|
|
type mockCommand struct {
|
|
aggregate *eventstore.Aggregate
|
|
payload any
|
|
constraints []*eventstore.UniqueConstraint
|
|
}
|
|
|
|
// Aggregate implements [eventstore.Command]
|
|
func (m *mockCommand) Aggregate() *eventstore.Aggregate {
|
|
return m.aggregate
|
|
}
|
|
|
|
// Creator implements [eventstore.Command]
|
|
func (m *mockCommand) Creator() string {
|
|
return "creator"
|
|
}
|
|
|
|
// Revision implements [eventstore.Command]
|
|
func (m *mockCommand) Revision() uint16 {
|
|
return 1
|
|
}
|
|
|
|
// Type implements [eventstore.Command]
|
|
func (m *mockCommand) Type() eventstore.EventType {
|
|
return "event.type"
|
|
}
|
|
|
|
// Payload implements [eventstore.Command]
|
|
func (m *mockCommand) Payload() any {
|
|
return m.payload
|
|
}
|
|
|
|
// UniqueConstraints implements [eventstore.Command]
|
|
func (m *mockCommand) UniqueConstraints() []*eventstore.UniqueConstraint {
|
|
return m.constraints
|
|
}
|
|
|
|
func mockEvent(aggregate *eventstore.Aggregate, sequence uint64, payload Payload) eventstore.Event {
|
|
return &event{
|
|
aggregate: aggregate,
|
|
creator: "creator",
|
|
revision: 1,
|
|
typ: "event.type",
|
|
sequence: sequence,
|
|
payload: payload,
|
|
}
|
|
}
|
|
|
|
func mockAggregate(id string) *eventstore.Aggregate {
|
|
return &eventstore.Aggregate{
|
|
ID: id,
|
|
Type: "type",
|
|
ResourceOwner: "ro",
|
|
InstanceID: "instance",
|
|
Version: "v1",
|
|
}
|
|
}
|