mirror of
https://github.com/zitadel/zitadel.git
synced 2025-01-09 04:43:39 +00:00
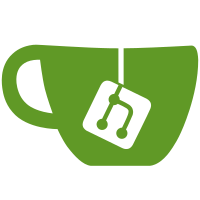
# Which Problems Are Solved Twilio supports a robust, multi-channel verification service that notably supports multi-region SMS sender numbers required for our use case. Currently, Zitadel does much of the work of the Twilio Verify (eg. localization, code generation, messaging) but doesn't support the pool of sender numbers that Twilio Verify does. # How the Problems Are Solved To support this API, we need to be able to store the Twilio Service ID and send that in a verification request where appropriate: phone number verification and SMS 2FA code paths. This PR does the following: - Adds the ability to use Twilio Verify of standard messaging through Twilio - Adds support for international numbers and more reliable verification messages sent from multiple numbers - Adds a new Twilio configuration option to support Twilio Verify in the admin console - Sends verification SMS messages through Twilio Verify - Implements Twilio Verification Checks for codes generated through the same # Additional Changes # Additional Context - base was implemented by @zhirschtritt in https://github.com/zitadel/zitadel/pull/8268 ❤️ - closes https://github.com/zitadel/zitadel/issues/8581 --------- Co-authored-by: Zachary Hirschtritt <zachary.hirschtritt@klaviyo.com> Co-authored-by: Joey Biscoglia <joey.biscoglia@klaviyo.com>
199 lines
5.0 KiB
Protocol Buffer
199 lines
5.0 KiB
Protocol Buffer
syntax = "proto3";
|
|
|
|
import "zitadel/object.proto";
|
|
import "validate/validate.proto";
|
|
import "google/protobuf/duration.proto";
|
|
import "protoc-gen-openapiv2/options/annotations.proto";
|
|
|
|
package zitadel.settings.v1;
|
|
|
|
option go_package = "github.com/zitadel/zitadel/pkg/grpc/settings";
|
|
|
|
message SecretGenerator {
|
|
SecretGeneratorType generator_type = 1;
|
|
zitadel.v1.ObjectDetails details = 2;
|
|
uint32 length = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "6";
|
|
}
|
|
];
|
|
google.protobuf.Duration expiry = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"3600s\"";
|
|
}
|
|
];
|
|
bool include_lower_letters = 5;
|
|
bool include_upper_letters = 6;
|
|
bool include_digits = 7;
|
|
bool include_symbols = 8;
|
|
}
|
|
|
|
message SecretGeneratorQuery {
|
|
oneof query {
|
|
option (validate.required) = true;
|
|
|
|
SecretGeneratorTypeQuery type_query = 1;
|
|
}
|
|
}
|
|
|
|
message SecretGeneratorTypeQuery {
|
|
SecretGeneratorType generator_type = 1;
|
|
}
|
|
|
|
enum SMTPConfigState {
|
|
SMTP_CONFIG_STATE_UNSPECIFIED = 0;
|
|
SMTP_CONFIG_ACTIVE = 1;
|
|
SMTP_CONFIG_INACTIVE = 2;
|
|
}
|
|
|
|
enum SecretGeneratorType {
|
|
SECRET_GENERATOR_TYPE_UNSPECIFIED = 0;
|
|
SECRET_GENERATOR_TYPE_INIT_CODE = 1;
|
|
SECRET_GENERATOR_TYPE_VERIFY_EMAIL_CODE = 2;
|
|
SECRET_GENERATOR_TYPE_VERIFY_PHONE_CODE = 3;
|
|
SECRET_GENERATOR_TYPE_PASSWORD_RESET_CODE = 4;
|
|
SECRET_GENERATOR_TYPE_PASSWORDLESS_INIT_CODE = 5;
|
|
SECRET_GENERATOR_TYPE_APP_SECRET = 6;
|
|
SECRET_GENERATOR_TYPE_OTP_SMS = 7;
|
|
SECRET_GENERATOR_TYPE_OTP_EMAIL = 8;
|
|
}
|
|
|
|
message SMTPConfig {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
string sender_address = 2 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"noreply@m.zitadel.cloud\"";
|
|
}
|
|
];
|
|
string sender_name = 3 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"ZITADEL\"";
|
|
}
|
|
];
|
|
bool tls = 4;
|
|
string host = 5 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"smtp.postmarkapp.com:587\"";
|
|
}
|
|
];
|
|
string user = 6 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"197f0117-529e-443d-bf6c-0292dd9a02b7\"";
|
|
}
|
|
];
|
|
string reply_to_address = 7 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"replyto@m.zitadel.cloud\"";
|
|
}
|
|
];
|
|
SMTPConfigState state = 8;
|
|
string description = 9 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"Mailjet\"";
|
|
}
|
|
];
|
|
string id = 10;
|
|
}
|
|
|
|
message EmailProvider {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
string id = 2;
|
|
EmailProviderState state = 3;
|
|
string description = 6;
|
|
|
|
oneof config {
|
|
EmailProviderSMTP smtp = 4;
|
|
EmailProviderHTTP http = 5;
|
|
}
|
|
}
|
|
|
|
enum EmailProviderState {
|
|
EMAIL_PROVIDER_STATE_UNSPECIFIED = 0;
|
|
EMAIL_PROVIDER_ACTIVE = 1;
|
|
EMAIL_PROVIDER_INACTIVE = 2;
|
|
}
|
|
|
|
message EmailProviderSMTP {
|
|
string sender_address = 1 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"noreply@m.zitadel.cloud\"";
|
|
}
|
|
];
|
|
string sender_name = 2 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"ZITADEL\"";
|
|
}
|
|
];
|
|
bool tls = 3;
|
|
string host = 4 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"smtp.postmarkapp.com:587\"";
|
|
}
|
|
];
|
|
string user = 5 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"197f0117-529e-443d-bf6c-0292dd9a02b7\"";
|
|
}
|
|
];
|
|
string reply_to_address = 6 [
|
|
(grpc.gateway.protoc_gen_openapiv2.options.openapiv2_field) = {
|
|
example: "\"replyto@m.zitadel.cloud\"";
|
|
}
|
|
];
|
|
}
|
|
|
|
message EmailProviderHTTP {
|
|
string endpoint = 1;
|
|
}
|
|
|
|
message SMSProvider {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
string id = 2;
|
|
SMSProviderConfigState state = 3;
|
|
string description = 6;
|
|
|
|
oneof config {
|
|
TwilioConfig twilio = 4;
|
|
HTTPConfig http = 5;
|
|
}
|
|
}
|
|
|
|
message TwilioConfig {
|
|
string sid = 1;
|
|
string sender_number = 2;
|
|
string verify_service_sid = 3;
|
|
}
|
|
|
|
message HTTPConfig {
|
|
string endpoint = 1;
|
|
}
|
|
|
|
enum SMSProviderConfigState {
|
|
SMS_PROVIDER_CONFIG_STATE_UNSPECIFIED = 0;
|
|
SMS_PROVIDER_CONFIG_ACTIVE = 1;
|
|
SMS_PROVIDER_CONFIG_INACTIVE = 2;
|
|
}
|
|
|
|
message DebugNotificationProvider {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
bool compact = 2;
|
|
}
|
|
|
|
message OIDCSettings {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
google.protobuf.Duration access_token_lifetime = 2;
|
|
google.protobuf.Duration id_token_lifetime = 3;
|
|
google.protobuf.Duration refresh_token_idle_expiration = 4;
|
|
google.protobuf.Duration refresh_token_expiration = 5;
|
|
}
|
|
|
|
message SecurityPolicy {
|
|
zitadel.v1.ObjectDetails details = 1;
|
|
// states if iframe embedding is enabled or disabled
|
|
bool enable_iframe_embedding = 2;
|
|
// origins allowed loading ZITADEL in an iframe if enable_iframe_embedding is true
|
|
repeated string allowed_origins = 3;
|
|
// allows users to impersonate other users. The impersonator needs the appropriate `*_IMPERSONATOR` roles assigned as well"
|
|
bool enable_impersonation = 4;
|
|
}
|