mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
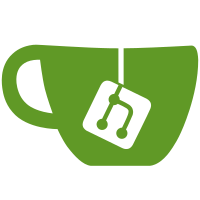
* commander * commander * selber! * move to packages * fix(errors): implement Is interface * test: command * test: commands * add init steps * setup tenant * add default step yaml * possibility to set password * merge v2 into v2-commander * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: search query builder can filter events in memory * fix: filters for add member * fix(setup): add `ExternalSecure` to config * chore: name iam to instance * fix: matching * remove unsued func * base url * base url * test(command): filter funcs * test: commands * fix: rename orgiampolicy to domain policy * start from init * commands * config * fix indexes and add constraints * fixes * fix: merge conflicts * fix: protos * fix: md files * setup * add deprecated org iam policy again * typo * fix search query * fix filter * Apply suggestions from code review * remove custom org from org setup * add todos for verification * change apps creation * simplify package structure * fix error * move preparation helper for tests * fix unique constraints * fix config mapping in setup * fix error handling in encryption_keys.go * fix projection config * fix query from old views to projection * fix setup of mgmt api * set iam project and fix instance projection * imports Co-authored-by: Livio Amstutz <livio.a@gmail.com> Co-authored-by: fabi <fabienne.gerschwiler@gmail.com>
85 lines
2.4 KiB
Go
85 lines
2.4 KiB
Go
package command
|
|
|
|
import (
|
|
"regexp"
|
|
|
|
"github.com/caos/zitadel/internal/domain"
|
|
"github.com/caos/zitadel/internal/errors"
|
|
"github.com/caos/zitadel/internal/eventstore"
|
|
"github.com/caos/zitadel/internal/repository/policy"
|
|
)
|
|
|
|
var (
|
|
hasStringLowerCase = regexp.MustCompile(`[a-z]`).MatchString
|
|
hasStringUpperCase = regexp.MustCompile(`[A-Z]`).MatchString
|
|
hasNumber = regexp.MustCompile(`[0-9]`).MatchString
|
|
hasSymbol = regexp.MustCompile(`[^A-Za-z0-9]`).MatchString
|
|
)
|
|
|
|
type PasswordComplexityPolicyWriteModel struct {
|
|
eventstore.WriteModel
|
|
|
|
MinLength uint64
|
|
HasLowercase bool
|
|
HasUppercase bool
|
|
HasNumber bool
|
|
HasSymbol bool
|
|
State domain.PolicyState
|
|
}
|
|
|
|
func (wm *PasswordComplexityPolicyWriteModel) Reduce() error {
|
|
for _, event := range wm.Events {
|
|
switch e := event.(type) {
|
|
case *policy.PasswordComplexityPolicyAddedEvent:
|
|
wm.MinLength = e.MinLength
|
|
wm.HasLowercase = e.HasLowercase
|
|
wm.HasUppercase = e.HasUppercase
|
|
wm.HasNumber = e.HasNumber
|
|
wm.HasSymbol = e.HasSymbol
|
|
wm.State = domain.PolicyStateActive
|
|
case *policy.PasswordComplexityPolicyChangedEvent:
|
|
if e.MinLength != nil {
|
|
wm.MinLength = *e.MinLength
|
|
}
|
|
if e.HasLowercase != nil {
|
|
wm.HasLowercase = *e.HasLowercase
|
|
}
|
|
if e.HasUppercase != nil {
|
|
wm.HasUppercase = *e.HasUppercase
|
|
}
|
|
if e.HasNumber != nil {
|
|
wm.HasNumber = *e.HasNumber
|
|
}
|
|
if e.HasSymbol != nil {
|
|
wm.HasSymbol = *e.HasSymbol
|
|
}
|
|
case *policy.PasswordComplexityPolicyRemovedEvent:
|
|
wm.State = domain.PolicyStateRemoved
|
|
}
|
|
}
|
|
return wm.WriteModel.Reduce()
|
|
}
|
|
|
|
func (wm *PasswordComplexityPolicyWriteModel) Validate(password string) error {
|
|
if wm.MinLength != 0 && uint64(len(password)) < wm.MinLength {
|
|
return errors.ThrowInvalidArgument(nil, "COMMA-HuJf6", "Errors.User.PasswordComplexityPolicy.MinLength")
|
|
}
|
|
|
|
if wm.HasLowercase && !hasStringLowerCase(password) {
|
|
return errors.ThrowInvalidArgument(nil, "COMMA-co3Xw", "Errors.User.PasswordComplexityPolicy.HasLower")
|
|
}
|
|
|
|
if wm.HasUppercase && !hasStringUpperCase(password) {
|
|
return errors.ThrowInvalidArgument(nil, "COMMA-VoaRj", "Errors.User.PasswordComplexityPolicy.HasUpper")
|
|
}
|
|
|
|
if wm.HasNumber && !hasNumber(password) {
|
|
return errors.ThrowInvalidArgument(nil, "COMMA-ZBv4H", "Errors.User.PasswordComplexityPolicy.HasNumber")
|
|
}
|
|
|
|
if wm.HasSymbol && !hasSymbol(password) {
|
|
return errors.ThrowInvalidArgument(nil, "COMMA-ZDLwA", "Errors.User.PasswordComplexityPolicy.HasSymbol")
|
|
}
|
|
return nil
|
|
}
|