mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
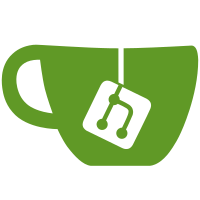
* chore: move to new org * logging * fix: org rename caos -> zitadel Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
package model
|
|
|
|
import (
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
)
|
|
|
|
type SecondFactorsSearchRequest struct {
|
|
Queries []*MFASearchQuery
|
|
}
|
|
|
|
type MultiFactorsSearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
Asc bool
|
|
Queries []*MFASearchQuery
|
|
}
|
|
|
|
type MFASearchQuery struct {
|
|
Key MFASearchKey
|
|
Method domain.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type MFASearchKey int32
|
|
|
|
const (
|
|
MFASearchKeyUnspecified MFASearchKey = iota
|
|
MFASearchKeyAggregateID
|
|
)
|
|
|
|
type SecondFactorsSearchResponse struct {
|
|
TotalResult uint64
|
|
Result []domain.SecondFactorType
|
|
}
|
|
|
|
type MultiFactorsSearchResponse struct {
|
|
TotalResult uint64
|
|
Result []domain.MultiFactorType
|
|
}
|
|
|
|
func (r *SecondFactorsSearchRequest) AppendAggregateIDQuery(aggregateID string) {
|
|
r.Queries = append(r.Queries, &MFASearchQuery{Key: MFASearchKeyAggregateID, Method: domain.SearchMethodEquals, Value: aggregateID})
|
|
}
|
|
|
|
func (r *MultiFactorsSearchRequest) AppendAggregateIDQuery(aggregateID string) {
|
|
r.Queries = append(r.Queries, &MFASearchQuery{Key: MFASearchKeyAggregateID, Method: domain.SearchMethodEquals, Value: aggregateID})
|
|
}
|