mirror of
https://github.com/zitadel/zitadel.git
synced 2025-05-02 23:10:49 +00:00
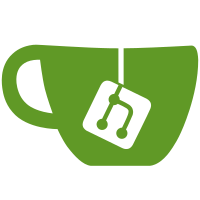
* feat(api): add google provider template * refactor reduce functions * handle removed event * linting * fix projection * feat(api): add generic oauth provider template * feat(api): add github provider templates * feat(api): add github provider templates * fixes * proto comment * fix filtering * requested changes * feat(api): add generic oauth provider template * remove wrongly committed message * increase budget for angular build * fix linting * fixes * fix merge * fix merge * fix projection * fix merge * updates from previous PRs * enable github providers in login * fix merge * fix test and add github styling in login * cleanup * feat(api): add gitlab provider templates * fix: merge * fix display of providers in login * implement gitlab in login and make prompt `select_account` optional since gitlab can't handle it * fix merge * fix merge and add tests for command side * requested changes * requested changes * Update internal/query/idp_template.go Co-authored-by: Silvan <silvan.reusser@gmail.com> * fix merge * requested changes --------- Co-authored-by: Silvan <silvan.reusser@gmail.com>
119 lines
2.5 KiB
Go
119 lines
2.5 KiB
Go
package domain
|
|
|
|
import (
|
|
"net/url"
|
|
"time"
|
|
|
|
"github.com/zitadel/logging"
|
|
|
|
"github.com/zitadel/zitadel/internal/eventstore/v1/models"
|
|
)
|
|
|
|
type LoginPolicy struct {
|
|
models.ObjectRoot
|
|
|
|
Default bool
|
|
AllowUsernamePassword bool
|
|
AllowRegister bool
|
|
AllowExternalIDP bool
|
|
IDPProviders []*IDPProvider
|
|
ForceMFA bool
|
|
SecondFactors []SecondFactorType
|
|
MultiFactors []MultiFactorType
|
|
PasswordlessType PasswordlessType
|
|
HidePasswordReset bool
|
|
IgnoreUnknownUsernames bool
|
|
AllowDomainDiscovery bool
|
|
DefaultRedirectURI string
|
|
PasswordCheckLifetime time.Duration
|
|
ExternalLoginCheckLifetime time.Duration
|
|
MFAInitSkipLifetime time.Duration
|
|
SecondFactorCheckLifetime time.Duration
|
|
MultiFactorCheckLifetime time.Duration
|
|
DisableLoginWithEmail bool
|
|
DisableLoginWithPhone bool
|
|
}
|
|
|
|
func ValidateDefaultRedirectURI(rawURL string) bool {
|
|
if rawURL == "" {
|
|
return true
|
|
}
|
|
parsedURL, err := url.Parse(rawURL)
|
|
if err != nil {
|
|
return false
|
|
}
|
|
switch parsedURL.Scheme {
|
|
case "":
|
|
return false
|
|
case "http", "https":
|
|
return parsedURL.Host != ""
|
|
default:
|
|
return true
|
|
}
|
|
}
|
|
|
|
type IDPProvider struct {
|
|
models.ObjectRoot
|
|
Type IdentityProviderType
|
|
IDPConfigID string
|
|
|
|
Name string
|
|
StylingType IDPConfigStylingType // deprecated
|
|
IDPType IDPType
|
|
IDPState IDPConfigState
|
|
}
|
|
|
|
func (p IDPProvider) IsValid() bool {
|
|
return p.IDPConfigID != ""
|
|
}
|
|
|
|
// DisplayName returns the name or a default
|
|
// to be used when always a name must be displayed (e.g. login)
|
|
func (p IDPProvider) DisplayName() string {
|
|
if p.Name != "" {
|
|
return p.Name
|
|
}
|
|
switch p.IDPType {
|
|
case IDPTypeGitHub:
|
|
return "GitHub"
|
|
case IDPTypeGitLab:
|
|
return "GitLab"
|
|
case IDPTypeGoogle:
|
|
return "Google"
|
|
case IDPTypeUnspecified,
|
|
IDPTypeOIDC,
|
|
IDPTypeJWT,
|
|
IDPTypeOAuth,
|
|
IDPTypeLDAP,
|
|
IDPTypeAzureAD,
|
|
IDPTypeGitHubEnterprise,
|
|
IDPTypeGitLabSelfHosted:
|
|
fallthrough
|
|
default:
|
|
// we should never get here, so log it
|
|
logging.Errorf("name of provider (type %d) is empty - id: %s", p.IDPType, p.IDPConfigID)
|
|
return ""
|
|
}
|
|
}
|
|
|
|
type PasswordlessType int32
|
|
|
|
const (
|
|
PasswordlessTypeNotAllowed PasswordlessType = iota
|
|
PasswordlessTypeAllowed
|
|
|
|
passwordlessCount
|
|
)
|
|
|
|
func (f PasswordlessType) Valid() bool {
|
|
return f >= 0 && f < passwordlessCount
|
|
}
|
|
|
|
func (p *LoginPolicy) HasSecondFactors() bool {
|
|
return len(p.SecondFactors) > 0
|
|
}
|
|
|
|
func (p *LoginPolicy) HasMultiFactors() bool {
|
|
return len(p.MultiFactors) > 0
|
|
}
|