mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
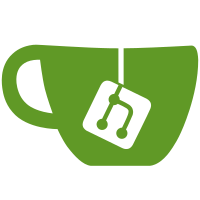
* fix: add events for domain * fix: add/remove domain command side * fix: add/remove domain command side * fix: add/remove domain query side * fix: create instance * fix: merge v2 * fix: instance domain * fix: instance domain * fix: instance domain * fix: instance domain * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from writemodels * fix: remove domain.IAMID from api * fix: remove domain.IAMID * fix: remove domain.IAMID * fix: add instance domain queries * fix: fix after merge * Update auth_request.go * fix keypair * remove unused code * feat: read instance id from context * feat: remove unused code * feat: use instance id from context * some fixes Co-authored-by: Livio Amstutz <livio.a@gmail.com>
155 lines
4.0 KiB
Go
155 lines
4.0 KiB
Go
package query
|
|
|
|
import (
|
|
"context"
|
|
"database/sql"
|
|
errs "errors"
|
|
"time"
|
|
|
|
sq "github.com/Masterminds/squirrel"
|
|
|
|
"github.com/caos/zitadel/internal/api/authz"
|
|
|
|
"github.com/caos/zitadel/internal/domain"
|
|
"github.com/caos/zitadel/internal/errors"
|
|
"github.com/caos/zitadel/internal/query/projection"
|
|
)
|
|
|
|
type LockoutPolicy struct {
|
|
ID string
|
|
Sequence uint64
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
ResourceOwner string
|
|
State domain.PolicyState
|
|
|
|
MaxPasswordAttempts uint64
|
|
ShowFailures bool
|
|
|
|
IsDefault bool
|
|
}
|
|
|
|
var (
|
|
lockoutTable = table{
|
|
name: projection.LockoutPolicyTable,
|
|
}
|
|
LockoutColID = Column{
|
|
name: projection.LockoutPolicyIDCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColInstanceID = Column{
|
|
name: projection.LockoutPolicyInstanceIDCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColSequence = Column{
|
|
name: projection.LockoutPolicySequenceCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColCreationDate = Column{
|
|
name: projection.LockoutPolicyCreationDateCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColChangeDate = Column{
|
|
name: projection.LockoutPolicyChangeDateCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColResourceOwner = Column{
|
|
name: projection.LockoutPolicyResourceOwnerCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColShowFailures = Column{
|
|
name: projection.LockoutPolicyShowLockOutFailuresCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColMaxPasswordAttempts = Column{
|
|
name: projection.LockoutPolicyMaxPasswordAttemptsCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColIsDefault = Column{
|
|
name: projection.LockoutPolicyIsDefaultCol,
|
|
table: lockoutTable,
|
|
}
|
|
LockoutColState = Column{
|
|
name: projection.LockoutPolicyStateCol,
|
|
table: lockoutTable,
|
|
}
|
|
)
|
|
|
|
func (q *Queries) LockoutPolicyByOrg(ctx context.Context, orgID string) (*LockoutPolicy, error) {
|
|
stmt, scan := prepareLockoutPolicyQuery()
|
|
query, args, err := stmt.Where(
|
|
sq.And{
|
|
sq.Eq{
|
|
LockoutColInstanceID.identifier(): authz.GetInstance(ctx).InstanceID(),
|
|
},
|
|
sq.Or{
|
|
sq.Eq{
|
|
LockoutColID.identifier(): orgID,
|
|
},
|
|
sq.Eq{
|
|
LockoutColID.identifier(): authz.GetInstance(ctx).InstanceID(),
|
|
},
|
|
},
|
|
}).
|
|
OrderBy(LockoutColIsDefault.identifier()).
|
|
Limit(1).ToSql()
|
|
if err != nil {
|
|
return nil, errors.ThrowInternal(err, "QUERY-SKR6X", "Errors.Query.SQLStatement")
|
|
}
|
|
|
|
row := q.client.QueryRowContext(ctx, query, args...)
|
|
return scan(row)
|
|
}
|
|
|
|
func (q *Queries) DefaultLockoutPolicy(ctx context.Context) (*LockoutPolicy, error) {
|
|
stmt, scan := prepareLockoutPolicyQuery()
|
|
query, args, err := stmt.Where(sq.Eq{
|
|
LockoutColID.identifier(): authz.GetInstance(ctx).InstanceID(),
|
|
LockoutColInstanceID.identifier(): authz.GetInstance(ctx).InstanceID(),
|
|
}).
|
|
OrderBy(LockoutColIsDefault.identifier()).
|
|
Limit(1).ToSql()
|
|
if err != nil {
|
|
return nil, errors.ThrowInternal(err, "QUERY-mN0Ci", "Errors.Query.SQLStatement")
|
|
}
|
|
|
|
row := q.client.QueryRowContext(ctx, query, args...)
|
|
return scan(row)
|
|
}
|
|
|
|
func prepareLockoutPolicyQuery() (sq.SelectBuilder, func(*sql.Row) (*LockoutPolicy, error)) {
|
|
return sq.Select(
|
|
LockoutColID.identifier(),
|
|
LockoutColSequence.identifier(),
|
|
LockoutColCreationDate.identifier(),
|
|
LockoutColChangeDate.identifier(),
|
|
LockoutColResourceOwner.identifier(),
|
|
LockoutColShowFailures.identifier(),
|
|
LockoutColMaxPasswordAttempts.identifier(),
|
|
LockoutColIsDefault.identifier(),
|
|
LockoutColState.identifier(),
|
|
).
|
|
From(lockoutTable.identifier()).PlaceholderFormat(sq.Dollar),
|
|
func(row *sql.Row) (*LockoutPolicy, error) {
|
|
policy := new(LockoutPolicy)
|
|
err := row.Scan(
|
|
&policy.ID,
|
|
&policy.Sequence,
|
|
&policy.CreationDate,
|
|
&policy.ChangeDate,
|
|
&policy.ResourceOwner,
|
|
&policy.ShowFailures,
|
|
&policy.MaxPasswordAttempts,
|
|
&policy.IsDefault,
|
|
&policy.State,
|
|
)
|
|
if err != nil {
|
|
if errs.Is(err, sql.ErrNoRows) {
|
|
return nil, errors.ThrowNotFound(err, "QUERY-63mtI", "Errors.PasswordComplexityPolicy.NotFound")
|
|
}
|
|
return nil, errors.ThrowInternal(err, "QUERY-uulCZ", "Errors.Internal")
|
|
}
|
|
return policy, nil
|
|
}
|
|
}
|