mirror of
https://github.com/zitadel/zitadel.git
synced 2025-07-15 12:28:36 +00:00
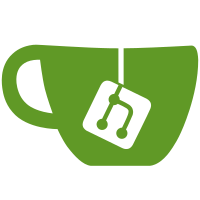
* add setup steps * refactoring * omitempty * cleanup * begin org * create org * setup org * setup org * merge * fixes * fixes * fixes * add project * add oidc application * fix app creation * add resourceOwner to writemodels * resource owner * cleanup * global org, iam project and iam member in setup * logs * logs * logs * cleanup * Update internal/v2/command/project.go Co-authored-by: Fabi <38692350+fgerschwiler@users.noreply.github.com> * check project state * add org domain commands * add org status changes and member commands * fixes * policies * login policy * fix iam project event * mapper * label policy * change to command * fix * fix * handle change event differently and lot of fixes * idps * fixes * fixes * fixes * changedEvent handling * fix change events * remove creation date Co-authored-by: Fabi <38692350+fgerschwiler@users.noreply.github.com>
71 lines
1.9 KiB
Go
71 lines
1.9 KiB
Go
package command
|
|
|
|
import (
|
|
"github.com/caos/zitadel/internal/crypto"
|
|
"github.com/caos/zitadel/internal/eventstore/v2"
|
|
"github.com/caos/zitadel/internal/v2/domain"
|
|
"github.com/caos/zitadel/internal/v2/repository/idpconfig"
|
|
)
|
|
|
|
type OIDCConfigWriteModel struct {
|
|
eventstore.WriteModel
|
|
|
|
IDPConfigID string
|
|
ClientID string
|
|
ClientSecret *crypto.CryptoValue
|
|
Issuer string
|
|
Scopes []string
|
|
|
|
IDPDisplayNameMapping domain.OIDCMappingField
|
|
UserNameMapping domain.OIDCMappingField
|
|
State domain.IDPConfigState
|
|
}
|
|
|
|
func (wm *OIDCConfigWriteModel) Reduce() error {
|
|
for _, event := range wm.Events {
|
|
switch e := event.(type) {
|
|
case *idpconfig.OIDCConfigAddedEvent:
|
|
wm.reduceConfigAddedEvent(e)
|
|
case *idpconfig.OIDCConfigChangedEvent:
|
|
wm.reduceConfigChangedEvent(e)
|
|
case *idpconfig.IDPConfigDeactivatedEvent:
|
|
wm.State = domain.IDPConfigStateInactive
|
|
case *idpconfig.IDPConfigReactivatedEvent:
|
|
wm.State = domain.IDPConfigStateActive
|
|
case *idpconfig.IDPConfigRemovedEvent:
|
|
wm.State = domain.IDPConfigStateRemoved
|
|
}
|
|
}
|
|
|
|
return wm.WriteModel.Reduce()
|
|
}
|
|
|
|
func (wm *OIDCConfigWriteModel) reduceConfigAddedEvent(e *idpconfig.OIDCConfigAddedEvent) {
|
|
wm.IDPConfigID = e.IDPConfigID
|
|
wm.ClientID = e.ClientID
|
|
wm.ClientSecret = e.ClientSecret
|
|
wm.Issuer = e.Issuer
|
|
wm.Scopes = e.Scopes
|
|
wm.IDPDisplayNameMapping = e.IDPDisplayNameMapping
|
|
wm.UserNameMapping = e.UserNameMapping
|
|
wm.State = domain.IDPConfigStateActive
|
|
}
|
|
|
|
func (wm *OIDCConfigWriteModel) reduceConfigChangedEvent(e *idpconfig.OIDCConfigChangedEvent) {
|
|
if e.ClientID != nil {
|
|
wm.ClientID = *e.ClientID
|
|
}
|
|
if e.Issuer != nil {
|
|
wm.Issuer = *e.Issuer
|
|
}
|
|
if len(e.Scopes) > 0 {
|
|
wm.Scopes = e.Scopes
|
|
}
|
|
if e.IDPDisplayNameMapping != nil && e.IDPDisplayNameMapping.Valid() {
|
|
wm.IDPDisplayNameMapping = *e.IDPDisplayNameMapping
|
|
}
|
|
if e.UserNameMapping != nil && e.UserNameMapping.Valid() {
|
|
wm.UserNameMapping = *e.UserNameMapping
|
|
}
|
|
}
|