mirror of
https://github.com/zitadel/zitadel.git
synced 2025-04-21 19:51:33 +00:00
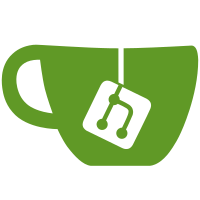
* begin init checks for projections * first projection checks * debug notification providers with query fixes * more projections and first index * more projections * more projections * finish projections * fix tests (remove db name) * create tables in setup * fix logging / error handling * add tenant to views * rename tenant to instance_id * add instance_id to all projections * add instance_id to all queries * correct instance_id on projections * add instance_id to failed_events * use separate context for instance * implement features projection * implement features projection * remove unique constraint from setup when migration failed * add error to failed setup event * add instance_id to primary keys * fix IAM projection * remove old migrations folder * fix keysFromYAML test
39 lines
1003 B
Go
39 lines
1003 B
Go
package login
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/caos/zitadel/internal/domain"
|
|
|
|
"github.com/caos/zitadel/internal/api/authz"
|
|
http_mw "github.com/caos/zitadel/internal/api/http/middleware"
|
|
)
|
|
|
|
const (
|
|
QueryAuthRequestID = "authRequestID"
|
|
queryUserAgentID = "userAgentID"
|
|
)
|
|
|
|
func (l *Login) getAuthRequest(r *http.Request) (*domain.AuthRequest, error) {
|
|
authRequestID := r.FormValue(QueryAuthRequestID)
|
|
if authRequestID == "" {
|
|
return nil, nil
|
|
}
|
|
userAgentID, _ := http_mw.UserAgentIDFromCtx(r.Context())
|
|
instanceID := authz.GetInstance(r.Context()).ID
|
|
return l.authRepo.AuthRequestByID(r.Context(), authRequestID, userAgentID, instanceID)
|
|
}
|
|
|
|
func (l *Login) getAuthRequestAndParseData(r *http.Request, data interface{}) (*domain.AuthRequest, error) {
|
|
authReq, err := l.getAuthRequest(r)
|
|
if err != nil {
|
|
return authReq, err
|
|
}
|
|
err = l.parser.Parse(r, data)
|
|
return authReq, err
|
|
}
|
|
|
|
func (l *Login) getParseData(r *http.Request, data interface{}) error {
|
|
return l.parser.Parse(r, data)
|
|
}
|