mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
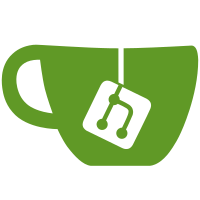
* begin init checks for projections * first projection checks * debug notification providers with query fixes * more projections and first index * more projections * more projections * finish projections * fix tests (remove db name) * create tables in setup * fix logging / error handling * add tenant to views * rename tenant to instance_id * add instance_id to all projections * add instance_id to all queries * correct instance_id on projections * add instance_id to failed_events * use separate context for instance * implement features projection * implement features projection * remove unique constraint from setup when migration failed * add error to failed setup event * add instance_id to primary keys * fix IAM projection * remove old migrations folder * fix keysFromYAML test
69 lines
2.3 KiB
Go
69 lines
2.3 KiB
Go
package repository
|
|
|
|
import (
|
|
"database/sql"
|
|
"time"
|
|
)
|
|
|
|
//Event represents all information about a manipulation of an aggregate
|
|
type Event struct {
|
|
//ID is a generated uuid for this event
|
|
ID string
|
|
|
|
//Sequence is the sequence of the event
|
|
Sequence uint64
|
|
|
|
//PreviousAggregateSequence is the sequence of the previous sequence of the aggregate (e.g. org.250989)
|
|
// if it's 0 then it's the first event of this aggregate
|
|
PreviousAggregateSequence uint64
|
|
|
|
//PreviousAggregateTypeSequence is the sequence of the previous sequence of the aggregate root (e.g. org)
|
|
// the first event of the aggregate has previous aggregate root sequence 0
|
|
PreviousAggregateTypeSequence uint64
|
|
|
|
//CreationDate is the time the event is created
|
|
// it's used for human readability.
|
|
// Don't use it for event ordering,
|
|
// time drifts in different services could cause integrity problems
|
|
CreationDate time.Time
|
|
|
|
//Type describes the cause of the event (e.g. user.added)
|
|
// it should always be in past-form
|
|
Type EventType
|
|
|
|
//Data describe the changed fields (e.g. userName = "hodor")
|
|
// data must always a pointer to a struct, a struct or a byte array containing json bytes
|
|
Data []byte
|
|
|
|
//EditorService should be a unique identifier for the service which created the event
|
|
// it's meant for maintainability
|
|
EditorService string
|
|
//EditorUser should be a unique identifier for the user which created the event
|
|
// it's meant for maintainability.
|
|
// It's recommend to use the aggregate id of the user
|
|
EditorUser string
|
|
|
|
//Version describes the definition of the aggregate at a certain point in time
|
|
// it's used in read models to reduce the events in the correct definition
|
|
Version Version
|
|
//AggregateID id is the unique identifier of the aggregate
|
|
// the client must generate it by it's own
|
|
AggregateID string
|
|
//AggregateType describes the meaning of the aggregate for this event
|
|
// it could an object like user
|
|
AggregateType AggregateType
|
|
//ResourceOwner is the organisation which owns this aggregate
|
|
// an aggregate can only be managed by one organisation
|
|
// use the ID of the org
|
|
ResourceOwner sql.NullString
|
|
//InstanceID is the instance where this event belongs to
|
|
// use the ID of the instance
|
|
InstanceID sql.NullString
|
|
}
|
|
|
|
//EventType is the description of the change
|
|
type EventType string
|
|
|
|
//AggregateType is the object name
|
|
type AggregateType string
|