mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
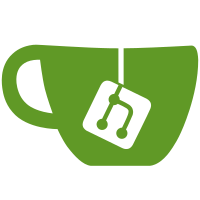
* feat(instance): implement create instance with direct machine user and credentials
* fix: deprecated add endpoint and variable declaration
* fix(instance): update logic for pats and machinekeys
* fix(instance): unit test corrections and additional unit test for pats and machinekeys
* fix(instance-create): include review changes
* fix(instance-create): linter fixes
* move iframe usage to solution scenarios configurations
* Revert "move iframe usage to solution scenarios configurations"
This reverts commit 9db31f3808
.
* fix merge
* fix: add review suggestions
Co-authored-by: Livio Spring <livio.a@gmail.com>
* fix: add review changes
* fix: add review changes for default definitions
* fix: add review changes for machinekey details
* fix: add machinekey output when setup with machineuser
* fix: add changes from review
* fix instance converter for machine and allow overwriting of further machine fields
Co-authored-by: Livio Spring <livio.a@gmail.com>
66 lines
1.6 KiB
Go
66 lines
1.6 KiB
Go
package domain
|
|
|
|
import (
|
|
"github.com/zitadel/logging"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
)
|
|
|
|
type authNKey interface {
|
|
SetPublicKey([]byte)
|
|
SetPrivateKey([]byte)
|
|
expiration
|
|
}
|
|
|
|
type AuthNKeyType int32
|
|
|
|
const (
|
|
AuthNKeyTypeNONE AuthNKeyType = iota
|
|
AuthNKeyTypeJSON
|
|
|
|
keyCount
|
|
)
|
|
|
|
func (k AuthNKeyType) Valid() bool {
|
|
return k >= 0 && k < keyCount
|
|
}
|
|
|
|
func (key *MachineKey) GenerateNewMachineKeyPair(keySize int) error {
|
|
privateKey, publicKey, err := crypto.GenerateKeyPair(keySize)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
key.PublicKey, err = crypto.PublicKeyToBytes(publicKey)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
key.PrivateKey = crypto.PrivateKeyToBytes(privateKey)
|
|
return nil
|
|
}
|
|
|
|
func SetNewAuthNKeyPair(key authNKey, keySize int) error {
|
|
privateKey, publicKey, err := NewAuthNKeyPair(keySize)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
key.SetPrivateKey(privateKey)
|
|
key.SetPublicKey(publicKey)
|
|
return nil
|
|
}
|
|
|
|
func NewAuthNKeyPair(keySize int) (privateKey, publicKey []byte, err error) {
|
|
private, public, err := crypto.GenerateKeyPair(keySize)
|
|
if err != nil {
|
|
logging.Log("AUTHN-Ud51I").WithError(err).Error("unable to create authn key pair")
|
|
return nil, nil, errors.ThrowInternal(err, "AUTHN-gdg2l", "Errors.Project.CouldNotGenerateClientSecret")
|
|
}
|
|
publicKey, err = crypto.PublicKeyToBytes(public)
|
|
if err != nil {
|
|
logging.Log("AUTHN-Dbb35").WithError(err).Error("unable to convert public key")
|
|
return nil, nil, errors.ThrowInternal(err, "AUTHN-Bne3f", "Errors.Project.CouldNotGenerateClientSecret")
|
|
}
|
|
privateKey = crypto.PrivateKeyToBytes(private)
|
|
return privateKey, publicKey, nil
|
|
}
|