mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
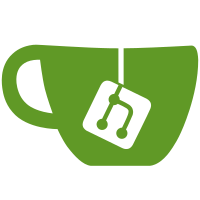
* commander * commander * selber! * move to packages * fix(errors): implement Is interface * test: command * test: commands * add init steps * setup tenant * add default step yaml * possibility to set password * merge v2 into v2-commander * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: rename iam command side to instance * fix: search query builder can filter events in memory * fix: filters for add member * fix(setup): add `ExternalSecure` to config * chore: name iam to instance * fix: matching * remove unsued func * base url * base url * test(command): filter funcs * test: commands * fix: rename orgiampolicy to domain policy * start from init * commands * config * fix indexes and add constraints * fixes * fix: merge conflicts * fix: protos * fix: md files * setup * add deprecated org iam policy again * typo * fix search query * fix filter * Apply suggestions from code review * remove custom org from org setup * add todos for verification * change apps creation * simplify package structure * fix error * move preparation helper for tests * fix unique constraints * fix config mapping in setup * fix error handling in encryption_keys.go * fix projection config * fix query from old views to projection * fix setup of mgmt api * set iam project and fix instance projection * fix tokens view * fix steps.yaml and defaults.yaml * fix projections * change instance context to interface * instance interceptors and additional events in setup * cleanup * tests for interceptors * fix label policy * add todo * single api endpoint in environment.json Co-authored-by: adlerhurst <silvan.reusser@gmail.com> Co-authored-by: fabi <fabienne.gerschwiler@gmail.com>
172 lines
5.8 KiB
Go
172 lines
5.8 KiB
Go
package projection
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/caos/zitadel/internal/errors"
|
|
"github.com/caos/zitadel/internal/eventstore"
|
|
"github.com/caos/zitadel/internal/eventstore/handler"
|
|
"github.com/caos/zitadel/internal/eventstore/handler/crdb"
|
|
"github.com/caos/zitadel/internal/repository/instance"
|
|
)
|
|
|
|
const (
|
|
InstanceProjectionTable = "projections.instances"
|
|
|
|
InstanceColumnID = "id"
|
|
InstanceColumnChangeDate = "change_date"
|
|
InstanceColumnGlobalOrgID = "global_org_id"
|
|
InstanceColumnProjectID = "iam_project_id"
|
|
InstanceColumnConsoleID = "console_client_id"
|
|
InstanceColumnSequence = "sequence"
|
|
InstanceColumnSetUpStarted = "setup_started"
|
|
InstanceColumnSetUpDone = "setup_done"
|
|
InstanceColumnDefaultLanguage = "default_language"
|
|
)
|
|
|
|
type InstanceProjection struct {
|
|
crdb.StatementHandler
|
|
}
|
|
|
|
func NewInstanceProjection(ctx context.Context, config crdb.StatementHandlerConfig) *InstanceProjection {
|
|
p := new(InstanceProjection)
|
|
config.ProjectionName = InstanceProjectionTable
|
|
config.Reducers = p.reducers()
|
|
config.InitCheck = crdb.NewTableCheck(
|
|
crdb.NewTable([]*crdb.Column{
|
|
crdb.NewColumn(InstanceColumnID, crdb.ColumnTypeText),
|
|
crdb.NewColumn(InstanceColumnChangeDate, crdb.ColumnTypeTimestamp),
|
|
crdb.NewColumn(InstanceColumnGlobalOrgID, crdb.ColumnTypeText, crdb.Default("")),
|
|
crdb.NewColumn(InstanceColumnProjectID, crdb.ColumnTypeText, crdb.Default("")),
|
|
crdb.NewColumn(InstanceColumnConsoleID, crdb.ColumnTypeText, crdb.Default("")),
|
|
crdb.NewColumn(InstanceColumnSequence, crdb.ColumnTypeInt64),
|
|
crdb.NewColumn(InstanceColumnSetUpStarted, crdb.ColumnTypeInt64, crdb.Default(0)),
|
|
crdb.NewColumn(InstanceColumnSetUpDone, crdb.ColumnTypeInt64, crdb.Default(0)),
|
|
crdb.NewColumn(InstanceColumnDefaultLanguage, crdb.ColumnTypeText, crdb.Default("")),
|
|
},
|
|
crdb.NewPrimaryKey(InstanceColumnID),
|
|
),
|
|
)
|
|
p.StatementHandler = crdb.NewStatementHandler(ctx, config)
|
|
return p
|
|
}
|
|
|
|
func (p *InstanceProjection) reducers() []handler.AggregateReducer {
|
|
return []handler.AggregateReducer{
|
|
{
|
|
Aggregate: instance.AggregateType,
|
|
EventRedusers: []handler.EventReducer{
|
|
{
|
|
Event: instance.GlobalOrgSetEventType,
|
|
Reduce: p.reduceGlobalOrgSet,
|
|
},
|
|
{
|
|
Event: instance.ProjectSetEventType,
|
|
Reduce: p.reduceIAMProjectSet,
|
|
},
|
|
{
|
|
Event: instance.ConsoleSetEventType,
|
|
Reduce: p.reduceConsoleSet,
|
|
},
|
|
{
|
|
Event: instance.DefaultLanguageSetEventType,
|
|
Reduce: p.reduceDefaultLanguageSet,
|
|
},
|
|
{
|
|
Event: instance.SetupStartedEventType,
|
|
Reduce: p.reduceSetupEvent,
|
|
},
|
|
{
|
|
Event: instance.SetupDoneEventType,
|
|
Reduce: p.reduceSetupEvent,
|
|
},
|
|
},
|
|
},
|
|
}
|
|
}
|
|
|
|
func (p *InstanceProjection) reduceGlobalOrgSet(event eventstore.Event) (*handler.Statement, error) {
|
|
e, ok := event.(*instance.GlobalOrgSetEvent)
|
|
if !ok {
|
|
return nil, errors.ThrowInvalidArgumentf(nil, "HANDL-2n9f2", "reduce.wrong.event.type %s", instance.GlobalOrgSetEventType)
|
|
}
|
|
return crdb.NewUpsertStatement(
|
|
e,
|
|
[]handler.Column{
|
|
handler.NewCol(InstanceColumnID, e.Aggregate().InstanceID),
|
|
handler.NewCol(InstanceColumnChangeDate, e.CreationDate()),
|
|
handler.NewCol(InstanceColumnSequence, e.Sequence()),
|
|
handler.NewCol(InstanceColumnGlobalOrgID, e.OrgID),
|
|
},
|
|
), nil
|
|
}
|
|
|
|
func (p *InstanceProjection) reduceIAMProjectSet(event eventstore.Event) (*handler.Statement, error) {
|
|
e, ok := event.(*instance.ProjectSetEvent)
|
|
if !ok {
|
|
return nil, errors.ThrowInvalidArgumentf(nil, "HANDL-30o0e", "reduce.wrong.event.type %s", instance.ProjectSetEventType)
|
|
}
|
|
return crdb.NewUpsertStatement(
|
|
e,
|
|
[]handler.Column{
|
|
handler.NewCol(InstanceColumnID, e.Aggregate().InstanceID),
|
|
handler.NewCol(InstanceColumnChangeDate, e.CreationDate()),
|
|
handler.NewCol(InstanceColumnSequence, e.Sequence()),
|
|
handler.NewCol(InstanceColumnProjectID, e.ProjectID),
|
|
},
|
|
), nil
|
|
}
|
|
|
|
func (p *InstanceProjection) reduceConsoleSet(event eventstore.Event) (*handler.Statement, error) {
|
|
e, ok := event.(*instance.ConsoleSetEvent)
|
|
if !ok {
|
|
return nil, errors.ThrowInvalidArgumentf(nil, "HANDL-Dgf11", "reduce.wrong.event.type %s", instance.ConsoleSetEventType)
|
|
}
|
|
return crdb.NewUpsertStatement(
|
|
e,
|
|
[]handler.Column{
|
|
handler.NewCol(InstanceColumnID, e.Aggregate().InstanceID),
|
|
handler.NewCol(InstanceColumnChangeDate, e.CreationDate()),
|
|
handler.NewCol(InstanceColumnSequence, e.Sequence()),
|
|
handler.NewCol(InstanceColumnConsoleID, e.ClientID),
|
|
},
|
|
), nil
|
|
}
|
|
|
|
func (p *InstanceProjection) reduceDefaultLanguageSet(event eventstore.Event) (*handler.Statement, error) {
|
|
e, ok := event.(*instance.DefaultLanguageSetEvent)
|
|
if !ok {
|
|
return nil, errors.ThrowInvalidArgumentf(nil, "HANDL-30o0e", "reduce.wrong.event.type %s", instance.DefaultLanguageSetEventType)
|
|
}
|
|
return crdb.NewUpsertStatement(
|
|
e,
|
|
[]handler.Column{
|
|
handler.NewCol(InstanceColumnID, e.Aggregate().InstanceID),
|
|
handler.NewCol(InstanceColumnChangeDate, e.CreationDate()),
|
|
handler.NewCol(InstanceColumnSequence, e.Sequence()),
|
|
handler.NewCol(InstanceColumnDefaultLanguage, e.Language.String()),
|
|
},
|
|
), nil
|
|
}
|
|
|
|
func (p *InstanceProjection) reduceSetupEvent(event eventstore.Event) (*handler.Statement, error) {
|
|
e, ok := event.(*instance.SetupStepEvent)
|
|
if !ok {
|
|
return nil, errors.ThrowInvalidArgumentf(nil, "HANDL-d9nfw", "reduce.wrong.event.type %v", []eventstore.EventType{instance.SetupDoneEventType, instance.SetupStartedEventType})
|
|
}
|
|
columns := []handler.Column{
|
|
handler.NewCol(InstanceColumnID, e.Aggregate().InstanceID),
|
|
handler.NewCol(InstanceColumnChangeDate, e.CreationDate()),
|
|
handler.NewCol(InstanceColumnSequence, e.Sequence()),
|
|
}
|
|
if e.EventType == instance.SetupStartedEventType {
|
|
columns = append(columns, handler.NewCol(InstanceColumnSetUpStarted, e.Step))
|
|
} else {
|
|
columns = append(columns, handler.NewCol(InstanceColumnSetUpDone, e.Step))
|
|
}
|
|
return crdb.NewUpsertStatement(
|
|
e,
|
|
columns,
|
|
), nil
|
|
}
|