mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
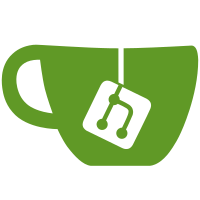
* fix(oidc): return bad request for base64 errors We've recently noticed an increased amount of 500: internal server error status returns on zitadel cloud. The source of these errors appear to be erroneous input in fields that are supposed to be bas64 formatted. ``` time=2024-04-08T14:05:47.600Z level=ERROR msg="request error" oidc_error.parent="ID=OIDC-AhX2u Message=Errors.Internal Parent=(illegal base64 data at input byte 8)" oidc_error.description=Errors.Internal oidc_error.type=server_error status_code=500 ``` Within the possible code paths of the token endpoint there are a couple of uses of base64.Encoding.DecodeString of which a returned error was not properly wrapped, but returned as-is. This causes the oidc error handler to return a 500 with the `OIDC-AhX2u` ID. We were not able to pinpoint the exact errors that are happening to any one call of `DecodeString`. This fix wraps all errors from `DecodeString` so that proper 400: bad request is returned with information about the error. Each wrapper now has an unique error ID, so that logs will contain the source of the error as well. This bug was reported internally by the ops team. * catch op.ErrInvalidRefreshToken
38 lines
1.2 KiB
Go
38 lines
1.2 KiB
Go
package domain
|
|
|
|
import (
|
|
"encoding/base64"
|
|
"strings"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
func NewRefreshToken(userID, tokenID string, algorithm crypto.EncryptionAlgorithm) (string, error) {
|
|
return RefreshToken(userID, tokenID, tokenID, algorithm)
|
|
}
|
|
|
|
func RefreshToken(userID, tokenID, token string, algorithm crypto.EncryptionAlgorithm) (string, error) {
|
|
encrypted, err := algorithm.Encrypt([]byte(userID + ":" + tokenID + ":" + token))
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
return base64.RawURLEncoding.EncodeToString(encrypted), nil
|
|
}
|
|
|
|
func FromRefreshToken(refreshToken string, algorithm crypto.EncryptionAlgorithm) (userID, tokenID, token string, err error) {
|
|
decoded, err := base64.RawURLEncoding.DecodeString(refreshToken)
|
|
if err != nil {
|
|
return "", "", "", zerrors.ThrowInvalidArgument(err, "DOMAIN-BGDhn", "Errors.User.RefreshToken.Invalid")
|
|
}
|
|
decrypted, err := algorithm.Decrypt(decoded, algorithm.EncryptionKeyID())
|
|
if err != nil {
|
|
return "", "", "", err
|
|
}
|
|
split := strings.Split(string(decrypted), ":")
|
|
if len(split) != 3 {
|
|
return "", "", "", zerrors.ThrowInvalidArgument(nil, "DOMAIN-BGDhn", "Errors.User.RefreshToken.Invalid")
|
|
}
|
|
return split[0], split[1], split[2], nil
|
|
}
|