mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
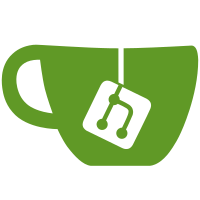
* feat: set quotas
* fix: start new period on younger anchor
* cleanup e2e config
* fix set notifications
* lint
* test: fix quota projection tests
* fix add quota tests
* make quota fields nullable
* enable amount 0
* fix initial setup
* create a prerelease
* avoid success comments
* fix quota projection primary key
* Revert "fix quota projection primary key"
This reverts commit e72f4d7fa1
.
* simplify write model
* fix aggregate id
* avoid push without changes
* test set quota lifecycle
* test set quota mutations
* fix quota unit test
* fix: quotas
* test quota.set event projection
* use SetQuota in integration tests
* fix: release quotas 3
* reset releaserc
* fix comment
* test notification order doesn't matter
* test notification order doesn't matter
* test with unmarshalled events
* test with unmarshalled events
54 lines
1.6 KiB
Go
54 lines
1.6 KiB
Go
package system
|
|
|
|
import (
|
|
"github.com/zitadel/zitadel/internal/command"
|
|
"github.com/zitadel/zitadel/pkg/grpc/quota"
|
|
"google.golang.org/protobuf/types/known/durationpb"
|
|
"google.golang.org/protobuf/types/known/timestamppb"
|
|
)
|
|
|
|
type setQuotaRequest interface {
|
|
GetUnit() quota.Unit
|
|
GetFrom() *timestamppb.Timestamp
|
|
GetResetInterval() *durationpb.Duration
|
|
GetAmount() uint64
|
|
GetLimit() bool
|
|
GetNotifications() []*quota.Notification
|
|
}
|
|
|
|
func instanceQuotaPbToCommand(req setQuotaRequest) *command.SetQuota {
|
|
return &command.SetQuota{
|
|
Unit: instanceQuotaUnitPbToCommand(req.GetUnit()),
|
|
From: req.GetFrom().AsTime(),
|
|
ResetInterval: req.GetResetInterval().AsDuration(),
|
|
Amount: req.GetAmount(),
|
|
Limit: req.GetLimit(),
|
|
Notifications: instanceQuotaNotificationsPbToCommand(req.GetNotifications()),
|
|
}
|
|
}
|
|
|
|
func instanceQuotaUnitPbToCommand(unit quota.Unit) command.QuotaUnit {
|
|
switch unit {
|
|
case quota.Unit_UNIT_REQUESTS_ALL_AUTHENTICATED:
|
|
return command.QuotaRequestsAllAuthenticated
|
|
case quota.Unit_UNIT_ACTIONS_ALL_RUN_SECONDS:
|
|
return command.QuotaActionsAllRunsSeconds
|
|
case quota.Unit_UNIT_UNIMPLEMENTED:
|
|
fallthrough
|
|
default:
|
|
return command.QuotaUnit(unit.String())
|
|
}
|
|
}
|
|
|
|
func instanceQuotaNotificationsPbToCommand(req []*quota.Notification) command.QuotaNotifications {
|
|
notifications := make([]*command.QuotaNotification, len(req))
|
|
for idx, item := range req {
|
|
notifications[idx] = &command.QuotaNotification{
|
|
Percent: uint16(item.Percent),
|
|
Repeat: item.Repeat,
|
|
CallURL: item.CallUrl,
|
|
}
|
|
}
|
|
return notifications
|
|
}
|