mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
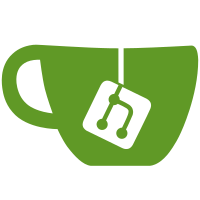
* document analytics config
* rework configuration and docs
* describe HandleActiveInstances better
* describe active instances on quotas better
* only projected events are considered
* cleanup
* describe changes at runtime
* push milestones
* stop tracking events
* calculate and push 4 in 6 milestones
* reduce milestone pushed
* remove docs
* fix scheduled pseudo event projection
* push 5 in 6 milestones
* push 6 in 6 milestones
* ignore client ids
* fix text array contains
* push human readable milestone type
* statement unit tests
* improve dev and db performance
* organize imports
* cleanup
* organize imports
* test projection
* check rows.Err()
* test search query
* pass linting
* review
* test 4 milestones
* simplify milestone by instance ids query
* use type NamespacedCondition
* cleanup
* lint
* lint
* dont overwrite original error
* no opt-in in examples
* cleanup
* prerelease
* enable request headers
* make limit configurable
* review fixes
* only requeue special handlers secondly
* include integration tests
* Revert "include integration tests"
This reverts commit 96db9504ec
.
* pass reducers
* test handlers
* fix unit test
* feat: increment version
* lint
* remove prerelease
* fix integration tests
100 lines
2.8 KiB
Go
100 lines
2.8 KiB
Go
package handlers
|
|
|
|
import (
|
|
"context"
|
|
"net/http"
|
|
|
|
"github.com/zitadel/zitadel/internal/command"
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/eventstore/handler"
|
|
"github.com/zitadel/zitadel/internal/eventstore/handler/crdb"
|
|
"github.com/zitadel/zitadel/internal/notification/channels/webhook"
|
|
_ "github.com/zitadel/zitadel/internal/notification/statik"
|
|
"github.com/zitadel/zitadel/internal/notification/types"
|
|
"github.com/zitadel/zitadel/internal/query/projection"
|
|
"github.com/zitadel/zitadel/internal/repository/quota"
|
|
)
|
|
|
|
const (
|
|
QuotaNotificationsProjectionTable = "projections.notifications_quota"
|
|
)
|
|
|
|
type quotaNotifier struct {
|
|
crdb.StatementHandler
|
|
commands *command.Commands
|
|
queries *NotificationQueries
|
|
metricSuccessfulDeliveriesJSON string
|
|
metricFailedDeliveriesJSON string
|
|
}
|
|
|
|
func NewQuotaNotifier(
|
|
ctx context.Context,
|
|
config crdb.StatementHandlerConfig,
|
|
commands *command.Commands,
|
|
queries *NotificationQueries,
|
|
metricSuccessfulDeliveriesJSON,
|
|
metricFailedDeliveriesJSON string,
|
|
) *quotaNotifier {
|
|
p := new(quotaNotifier)
|
|
config.ProjectionName = QuotaNotificationsProjectionTable
|
|
config.Reducers = p.reducers()
|
|
p.StatementHandler = crdb.NewStatementHandler(ctx, config)
|
|
p.commands = commands
|
|
p.queries = queries
|
|
p.metricSuccessfulDeliveriesJSON = metricSuccessfulDeliveriesJSON
|
|
p.metricFailedDeliveriesJSON = metricFailedDeliveriesJSON
|
|
projection.NotificationsQuotaProjection = p
|
|
return p
|
|
}
|
|
|
|
func (u *quotaNotifier) reducers() []handler.AggregateReducer {
|
|
return []handler.AggregateReducer{
|
|
{
|
|
Aggregate: quota.AggregateType,
|
|
EventRedusers: []handler.EventReducer{
|
|
{
|
|
Event: quota.NotificationDueEventType,
|
|
Reduce: u.reduceNotificationDue,
|
|
},
|
|
},
|
|
},
|
|
}
|
|
}
|
|
|
|
func (u *quotaNotifier) reduceNotificationDue(event eventstore.Event) (*handler.Statement, error) {
|
|
e, ok := event.(*quota.NotificationDueEvent)
|
|
if !ok {
|
|
return nil, errors.ThrowInvalidArgumentf(nil, "HANDL-DLxdE", "reduce.wrong.event.type %s", quota.NotificationDueEventType)
|
|
}
|
|
ctx := HandlerContext(event.Aggregate())
|
|
alreadyHandled, err := u.queries.IsAlreadyHandled(ctx, event, map[string]interface{}{"dueEventID": e.ID}, quota.AggregateType, quota.NotifiedEventType)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if alreadyHandled {
|
|
return crdb.NewNoOpStatement(e), nil
|
|
}
|
|
err = types.SendJSON(
|
|
ctx,
|
|
webhook.Config{
|
|
CallURL: e.CallURL,
|
|
Method: http.MethodPost,
|
|
},
|
|
u.queries.GetFileSystemProvider,
|
|
u.queries.GetLogProvider,
|
|
e,
|
|
e,
|
|
u.metricSuccessfulDeliveriesJSON,
|
|
u.metricFailedDeliveriesJSON,
|
|
).WithoutTemplate()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
err = u.commands.UsageNotificationSent(ctx, e)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return crdb.NewNoOpStatement(e), nil
|
|
}
|