mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
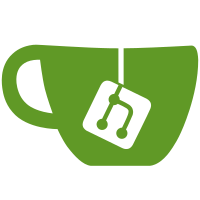
* feat: directly specify factors on addCustomLoginPolicy and return on LoginPolicy responses * fix proto * update login policy * feat: directly specify idp on addCustomLoginPolicy and return on LoginPolicy responses * fix: tests * fix(projection): trigger bulk * refactor: clean projection pkg * instance should bulk * fix(query): should trigger bulk on id calls * tests * build prerelease * fix: add shouldTriggerBulk * fix: test Co-authored-by: Livio Amstutz <livio.a@gmail.com> Co-authored-by: Max Peintner <max@caos.ch>
52 lines
1.8 KiB
Go
52 lines
1.8 KiB
Go
package eventstore
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
auth_view "github.com/zitadel/zitadel/internal/auth/repository/eventsourcing/view"
|
|
"github.com/zitadel/zitadel/internal/config/systemdefaults"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
eventstore "github.com/zitadel/zitadel/internal/eventstore/v1"
|
|
iam_model "github.com/zitadel/zitadel/internal/iam/model"
|
|
iam_view_model "github.com/zitadel/zitadel/internal/iam/repository/view/model"
|
|
"github.com/zitadel/zitadel/internal/query"
|
|
)
|
|
|
|
type OrgRepository struct {
|
|
SearchLimit uint64
|
|
|
|
Eventstore eventstore.Eventstore
|
|
View *auth_view.View
|
|
SystemDefaults systemdefaults.SystemDefaults
|
|
Query *query.Queries
|
|
}
|
|
|
|
func (repo *OrgRepository) GetIDPConfigByID(ctx context.Context, idpConfigID string) (*iam_model.IDPConfigView, error) {
|
|
idpConfig, err := repo.View.IDPConfigByID(idpConfigID, authz.GetInstance(ctx).InstanceID())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return iam_view_model.IDPConfigViewToModel(idpConfig), nil
|
|
}
|
|
|
|
func (repo *OrgRepository) GetMyPasswordComplexityPolicy(ctx context.Context) (*iam_model.PasswordComplexityPolicyView, error) {
|
|
policy, err := repo.Query.PasswordComplexityPolicyByOrg(ctx, true, authz.GetCtxData(ctx).OrgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return iam_view_model.PasswordComplexityViewToModel(policy), err
|
|
}
|
|
|
|
func (repo *OrgRepository) GetLoginText(ctx context.Context, orgID string) ([]*domain.CustomText, error) {
|
|
loginTexts, err := repo.Query.CustomTextListByTemplate(ctx, authz.GetInstance(ctx).InstanceID(), domain.LoginCustomText)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
orgLoginTexts, err := repo.Query.CustomTextListByTemplate(ctx, orgID, domain.LoginCustomText)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return append(query.CustomTextsToDomain(loginTexts), query.CustomTextsToDomain(orgLoginTexts)...), nil
|
|
}
|