mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
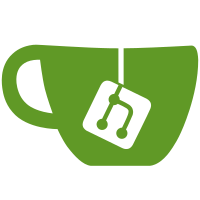
* reproduce #5808 Add an integration test that imports and gets N amount of human users. - With N set to 1-10 the operation seems to succeed always - With N set to 100 the operation seems to fail between 1 and 7 times. * fix merge issue * fix: reset the call timestamp after a bulk trigger With the use of `AS OF SYSTEM TIME` in queries, there was a change for the query package not finding the latest projection verson after a bulk trigger. If events where processed in the bulk trigger, the resulting row timestamp would be after the call start timestamp. This sometimes resulted in consistency issues when Set and Get API methods are called in short succession. For example a Import and Get user could sometimes result in a Not Found error. Although the issue was reported for the Management API user import, it is likely this bug contributed to the flaky integration and e2e tests. Fixes #5808 * trigger bulk action in GetSession * don't use the new context in handler schedule * disable reproduction test --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
292 lines
8.1 KiB
Go
292 lines
8.1 KiB
Go
package query
|
|
|
|
import (
|
|
"context"
|
|
"database/sql"
|
|
errs "errors"
|
|
"time"
|
|
|
|
sq "github.com/Masterminds/squirrel"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/api/call"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/query/projection"
|
|
"github.com/zitadel/zitadel/internal/telemetry/tracing"
|
|
)
|
|
|
|
var (
|
|
projectsTable = table{
|
|
name: projection.ProjectProjectionTable,
|
|
instanceIDCol: projection.ProjectColumnInstanceID,
|
|
}
|
|
ProjectColumnID = Column{
|
|
name: projection.ProjectColumnID,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnName = Column{
|
|
name: projection.ProjectColumnName,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnProjectRoleAssertion = Column{
|
|
name: projection.ProjectColumnProjectRoleAssertion,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnProjectRoleCheck = Column{
|
|
name: projection.ProjectColumnProjectRoleCheck,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnHasProjectCheck = Column{
|
|
name: projection.ProjectColumnHasProjectCheck,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnPrivateLabelingSetting = Column{
|
|
name: projection.ProjectColumnPrivateLabelingSetting,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnCreationDate = Column{
|
|
name: projection.ProjectColumnCreationDate,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnChangeDate = Column{
|
|
name: projection.ProjectColumnChangeDate,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnResourceOwner = Column{
|
|
name: projection.ProjectColumnResourceOwner,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnInstanceID = Column{
|
|
name: projection.ProjectColumnInstanceID,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnSequence = Column{
|
|
name: projection.ProjectColumnSequence,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnState = Column{
|
|
name: projection.ProjectColumnState,
|
|
table: projectsTable,
|
|
}
|
|
ProjectColumnOwnerRemoved = Column{
|
|
name: projection.ProjectColumnOwnerRemoved,
|
|
table: projectsTable,
|
|
}
|
|
)
|
|
|
|
type Projects struct {
|
|
SearchResponse
|
|
Projects []*Project
|
|
}
|
|
|
|
type Project struct {
|
|
ID string
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
ResourceOwner string
|
|
State domain.ProjectState
|
|
Sequence uint64
|
|
|
|
Name string
|
|
ProjectRoleAssertion bool
|
|
ProjectRoleCheck bool
|
|
HasProjectCheck bool
|
|
PrivateLabelingSetting domain.PrivateLabelingSetting
|
|
}
|
|
|
|
type ProjectSearchQueries struct {
|
|
SearchRequest
|
|
Queries []SearchQuery
|
|
}
|
|
|
|
func (q *Queries) ProjectByID(ctx context.Context, shouldTriggerBulk bool, id string, withOwnerRemoved bool) (_ *Project, err error) {
|
|
ctx, span := tracing.NewSpan(ctx)
|
|
defer func() { span.EndWithError(err) }()
|
|
|
|
if shouldTriggerBulk {
|
|
ctx = projection.ProjectProjection.Trigger(ctx)
|
|
}
|
|
|
|
stmt, scan := prepareProjectQuery(ctx, q.client)
|
|
eq := sq.Eq{
|
|
ProjectColumnID.identifier(): id,
|
|
ProjectColumnInstanceID.identifier(): authz.GetInstance(ctx).InstanceID(),
|
|
}
|
|
if !withOwnerRemoved {
|
|
eq[ProjectColumnOwnerRemoved.identifier()] = false
|
|
}
|
|
query, args, err := stmt.Where(eq).ToSql()
|
|
if err != nil {
|
|
return nil, errors.ThrowInternal(err, "QUERY-2m00Q", "Errors.Query.SQLStatment")
|
|
}
|
|
|
|
row := q.client.QueryRowContext(ctx, query, args...)
|
|
return scan(row)
|
|
}
|
|
|
|
func (q *Queries) SearchProjects(ctx context.Context, queries *ProjectSearchQueries, withOwnerRemoved bool) (projects *Projects, err error) {
|
|
ctx, span := tracing.NewSpan(ctx)
|
|
defer func() { span.EndWithError(err) }()
|
|
|
|
query, scan := prepareProjectsQuery(ctx, q.client)
|
|
eq := sq.Eq{ProjectColumnInstanceID.identifier(): authz.GetInstance(ctx).InstanceID()}
|
|
if !withOwnerRemoved {
|
|
eq[ProjectColumnOwnerRemoved.identifier()] = false
|
|
}
|
|
stmt, args, err := queries.toQuery(query).Where(eq).ToSql()
|
|
if err != nil {
|
|
return nil, errors.ThrowInvalidArgument(err, "QUERY-fn9ew", "Errors.Query.InvalidRequest")
|
|
}
|
|
|
|
rows, err := q.client.QueryContext(ctx, stmt, args...)
|
|
if err != nil {
|
|
return nil, errors.ThrowInternal(err, "QUERY-2j00f", "Errors.Internal")
|
|
}
|
|
projects, err = scan(rows)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
projects.LatestSequence, err = q.latestSequence(ctx, projectsTable)
|
|
return projects, err
|
|
}
|
|
|
|
func NewProjectNameSearchQuery(method TextComparison, value string) (SearchQuery, error) {
|
|
return NewTextQuery(ProjectColumnName, value, method)
|
|
}
|
|
|
|
func NewProjectIDSearchQuery(ids []string) (SearchQuery, error) {
|
|
list := make([]interface{}, len(ids))
|
|
for i, value := range ids {
|
|
list[i] = value
|
|
}
|
|
return NewListQuery(ProjectColumnID, list, ListIn)
|
|
}
|
|
|
|
func NewProjectResourceOwnerSearchQuery(value string) (SearchQuery, error) {
|
|
return NewTextQuery(ProjectColumnResourceOwner, value, TextEquals)
|
|
}
|
|
|
|
func (r *ProjectSearchQueries) AppendMyResourceOwnerQuery(orgID string) error {
|
|
query, err := NewProjectResourceOwnerSearchQuery(orgID)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
r.Queries = append(r.Queries, query)
|
|
return nil
|
|
}
|
|
|
|
func (r *ProjectSearchQueries) AppendPermissionQueries(permissions []string) error {
|
|
if !authz.HasGlobalPermission(permissions) {
|
|
ids := authz.GetAllPermissionCtxIDs(permissions)
|
|
query, err := NewProjectIDSearchQuery(ids)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
r.Queries = append(r.Queries, query)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (q *ProjectSearchQueries) toQuery(query sq.SelectBuilder) sq.SelectBuilder {
|
|
query = q.SearchRequest.toQuery(query)
|
|
for _, q := range q.Queries {
|
|
query = q.toQuery(query)
|
|
}
|
|
return query
|
|
}
|
|
|
|
func prepareProjectQuery(ctx context.Context, db prepareDatabase) (sq.SelectBuilder, func(*sql.Row) (*Project, error)) {
|
|
return sq.Select(
|
|
ProjectColumnID.identifier(),
|
|
ProjectColumnCreationDate.identifier(),
|
|
ProjectColumnChangeDate.identifier(),
|
|
ProjectColumnResourceOwner.identifier(),
|
|
ProjectColumnState.identifier(),
|
|
ProjectColumnSequence.identifier(),
|
|
ProjectColumnName.identifier(),
|
|
ProjectColumnProjectRoleAssertion.identifier(),
|
|
ProjectColumnProjectRoleCheck.identifier(),
|
|
ProjectColumnHasProjectCheck.identifier(),
|
|
ProjectColumnPrivateLabelingSetting.identifier()).
|
|
From(projectsTable.identifier() + db.Timetravel(call.Took(ctx))).
|
|
PlaceholderFormat(sq.Dollar),
|
|
func(row *sql.Row) (*Project, error) {
|
|
p := new(Project)
|
|
err := row.Scan(
|
|
&p.ID,
|
|
&p.CreationDate,
|
|
&p.ChangeDate,
|
|
&p.ResourceOwner,
|
|
&p.State,
|
|
&p.Sequence,
|
|
&p.Name,
|
|
&p.ProjectRoleAssertion,
|
|
&p.ProjectRoleCheck,
|
|
&p.HasProjectCheck,
|
|
&p.PrivateLabelingSetting,
|
|
)
|
|
if err != nil {
|
|
if errs.Is(err, sql.ErrNoRows) {
|
|
return nil, errors.ThrowNotFound(err, "QUERY-fk2fs", "Errors.Project.NotFound")
|
|
}
|
|
return nil, errors.ThrowInternal(err, "QUERY-dj2FF", "Errors.Internal")
|
|
}
|
|
return p, nil
|
|
}
|
|
}
|
|
|
|
func prepareProjectsQuery(ctx context.Context, db prepareDatabase) (sq.SelectBuilder, func(*sql.Rows) (*Projects, error)) {
|
|
return sq.Select(
|
|
ProjectColumnID.identifier(),
|
|
ProjectColumnCreationDate.identifier(),
|
|
ProjectColumnChangeDate.identifier(),
|
|
ProjectColumnResourceOwner.identifier(),
|
|
ProjectColumnState.identifier(),
|
|
ProjectColumnSequence.identifier(),
|
|
ProjectColumnName.identifier(),
|
|
ProjectColumnProjectRoleAssertion.identifier(),
|
|
ProjectColumnProjectRoleCheck.identifier(),
|
|
ProjectColumnHasProjectCheck.identifier(),
|
|
ProjectColumnPrivateLabelingSetting.identifier(),
|
|
countColumn.identifier()).
|
|
From(projectsTable.identifier() + db.Timetravel(call.Took(ctx))).
|
|
PlaceholderFormat(sq.Dollar),
|
|
func(rows *sql.Rows) (*Projects, error) {
|
|
projects := make([]*Project, 0)
|
|
var count uint64
|
|
for rows.Next() {
|
|
project := new(Project)
|
|
err := rows.Scan(
|
|
&project.ID,
|
|
&project.CreationDate,
|
|
&project.ChangeDate,
|
|
&project.ResourceOwner,
|
|
&project.State,
|
|
&project.Sequence,
|
|
&project.Name,
|
|
&project.ProjectRoleAssertion,
|
|
&project.ProjectRoleCheck,
|
|
&project.HasProjectCheck,
|
|
&project.PrivateLabelingSetting,
|
|
&count,
|
|
)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
projects = append(projects, project)
|
|
}
|
|
|
|
if err := rows.Close(); err != nil {
|
|
return nil, errors.ThrowInternal(err, "QUERY-QMXJv", "Errors.Query.CloseRows")
|
|
}
|
|
|
|
return &Projects{
|
|
Projects: projects,
|
|
SearchResponse: SearchResponse{
|
|
Count: count,
|
|
},
|
|
}, nil
|
|
}
|
|
}
|