mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
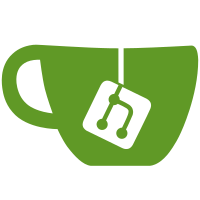
* chore: rename package errors to zerrors * rename package errors to gerrors * fix error related linting issues * fix zitadel error assertion * fix gosimple linting issues * fix deprecated linting issues * resolve gci linting issues * fix import structure --------- Co-authored-by: Elio Bischof <elio@zitadel.com>
74 lines
1.8 KiB
Go
74 lines
1.8 KiB
Go
package model
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
type UserSessionView struct {
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
State domain.UserSessionState
|
|
ResourceOwner string
|
|
UserAgentID string
|
|
UserID string
|
|
UserName string
|
|
LoginName string
|
|
DisplayName string
|
|
AvatarKey string
|
|
SelectedIDPConfigID string
|
|
PasswordVerification time.Time
|
|
PasswordlessVerification time.Time
|
|
ExternalLoginVerification time.Time
|
|
SecondFactorVerification time.Time
|
|
SecondFactorVerificationType domain.MFAType
|
|
MultiFactorVerification time.Time
|
|
MultiFactorVerificationType domain.MFAType
|
|
Sequence uint64
|
|
}
|
|
|
|
type UserSessionSearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
SortingColumn UserSessionSearchKey
|
|
Asc bool
|
|
Queries []*UserSessionSearchQuery
|
|
}
|
|
|
|
type UserSessionSearchKey int32
|
|
|
|
const (
|
|
UserSessionSearchKeyUnspecified UserSessionSearchKey = iota
|
|
UserSessionSearchKeyUserAgentID
|
|
UserSessionSearchKeyUserID
|
|
UserSessionSearchKeyState
|
|
UserSessionSearchKeyResourceOwner
|
|
UserSessionSearchKeyInstanceID
|
|
UserSessionSearchKeyOwnerRemoved
|
|
)
|
|
|
|
type UserSessionSearchQuery struct {
|
|
Key UserSessionSearchKey
|
|
Method domain.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type UserSessionSearchResponse struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
TotalResult uint64
|
|
Result []*UserSessionView
|
|
}
|
|
|
|
func (r *UserSessionSearchRequest) EnsureLimit(limit uint64) error {
|
|
if r.Limit > limit {
|
|
return zerrors.ThrowInvalidArgument(nil, "SEARCH-27ifs", "Errors.Limit.ExceedsDefault")
|
|
}
|
|
if r.Limit == 0 {
|
|
r.Limit = limit
|
|
}
|
|
return nil
|
|
}
|