mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
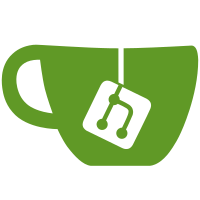
* chore: move to new org * logging * fix: org rename caos -> zitadel Co-authored-by: adlerhurst <silvan.reusser@gmail.com>
60 lines
1.4 KiB
Go
60 lines
1.4 KiB
Go
package sql
|
|
|
|
import (
|
|
"database/sql"
|
|
"os"
|
|
"testing"
|
|
|
|
"github.com/cockroachdb/cockroach-go/v2/testserver"
|
|
"github.com/zitadel/logging"
|
|
|
|
"github.com/zitadel/zitadel/cmd/admin/initialise"
|
|
)
|
|
|
|
var (
|
|
testCRDBClient *sql.DB
|
|
)
|
|
|
|
func TestMain(m *testing.M) {
|
|
ts, err := testserver.NewTestServer()
|
|
if err != nil {
|
|
logging.WithFields("error", err).Fatal("unable to start db")
|
|
}
|
|
|
|
testCRDBClient, err = sql.Open("postgres", ts.PGURL().String())
|
|
if err != nil {
|
|
logging.WithFields("error", err).Fatal("unable to connect to db")
|
|
}
|
|
if err = testCRDBClient.Ping(); err != nil {
|
|
logging.WithFields("error", err).Fatal("unable to ping db")
|
|
}
|
|
|
|
defer func() {
|
|
testCRDBClient.Close()
|
|
ts.Stop()
|
|
}()
|
|
|
|
if err = initDB(testCRDBClient); err != nil {
|
|
logging.WithFields("error", err).Fatal("migrations failed")
|
|
}
|
|
|
|
os.Exit(m.Run())
|
|
}
|
|
|
|
func initDB(db *sql.DB) error {
|
|
username := "zitadel"
|
|
database := "zitadel"
|
|
err := initialise.Initialise(db, initialise.VerifyUser(username, ""),
|
|
initialise.VerifyDatabase(database),
|
|
initialise.VerifyGrant(database, username))
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return initialise.VerifyZitadel(db)
|
|
}
|
|
|
|
func fillUniqueData(unique_type, field, instanceID string) error {
|
|
_, err := testCRDBClient.Exec("INSERT INTO eventstore.unique_constraints (unique_type, unique_field, instance_id) VALUES ($1, $2, $3)", unique_type, field, instanceID)
|
|
return err
|
|
}
|