mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
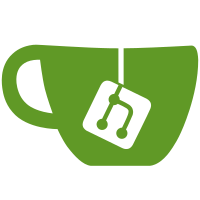
* feat: first implementation for saml sp * fix: add command side instance and org for saml provider * fix: add query side instance and org for saml provider * fix: request handling in event and retrieval of finished intent * fix: add review changes and integration tests * fix: add integration tests for saml idp * fix: correct unit tests with review changes * fix: add saml session unit test * fix: add saml session unit test * fix: add saml session unit test * fix: changes from review * fix: changes from review * fix: proto build error * fix: proto build error * fix: proto build error * fix: proto require metadata oneof * fix: login with saml provider * fix: integration test for saml assertion * lint client.go * fix json tag * fix: linting * fix import * fix: linting * fix saml idp query * fix: linting * lint: try all issues * revert linting config * fix: add regenerate endpoints * fix: translations * fix mk.yaml * ignore acs path for user agent cookie * fix: add AuthFromProvider test for saml * fix: integration test for saml retrieve information --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
196 lines
4.4 KiB
Go
196 lines
4.4 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/command/preparation"
|
|
"github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/repository/idp"
|
|
)
|
|
|
|
type GenericOAuthProvider struct {
|
|
Name string
|
|
ClientID string
|
|
ClientSecret string
|
|
AuthorizationEndpoint string
|
|
TokenEndpoint string
|
|
UserEndpoint string
|
|
Scopes []string
|
|
IDAttribute string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type GenericOIDCProvider struct {
|
|
Name string
|
|
Issuer string
|
|
ClientID string
|
|
ClientSecret string
|
|
Scopes []string
|
|
IsIDTokenMapping bool
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type JWTProvider struct {
|
|
Name string
|
|
Issuer string
|
|
JWTEndpoint string
|
|
KeyEndpoint string
|
|
HeaderName string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type AzureADProvider struct {
|
|
Name string
|
|
ClientID string
|
|
ClientSecret string
|
|
Scopes []string
|
|
Tenant string
|
|
EmailVerified bool
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type GitHubProvider struct {
|
|
Name string
|
|
ClientID string
|
|
ClientSecret string
|
|
Scopes []string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type GitHubEnterpriseProvider struct {
|
|
Name string
|
|
ClientID string
|
|
ClientSecret string
|
|
AuthorizationEndpoint string
|
|
TokenEndpoint string
|
|
UserEndpoint string
|
|
Scopes []string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type GitLabProvider struct {
|
|
Name string
|
|
ClientID string
|
|
ClientSecret string
|
|
Scopes []string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type GitLabSelfHostedProvider struct {
|
|
Name string
|
|
Issuer string
|
|
ClientID string
|
|
ClientSecret string
|
|
Scopes []string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type GoogleProvider struct {
|
|
Name string
|
|
ClientID string
|
|
ClientSecret string
|
|
Scopes []string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type LDAPProvider struct {
|
|
Name string
|
|
Servers []string
|
|
StartTLS bool
|
|
BaseDN string
|
|
BindDN string
|
|
BindPassword string
|
|
UserBase string
|
|
UserObjectClasses []string
|
|
UserFilters []string
|
|
Timeout time.Duration
|
|
LDAPAttributes idp.LDAPAttributes
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type SAMLProvider struct {
|
|
Name string
|
|
Metadata []byte
|
|
MetadataURL string
|
|
Binding string
|
|
WithSignedRequest bool
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
type AppleProvider struct {
|
|
Name string
|
|
ClientID string
|
|
TeamID string
|
|
KeyID string
|
|
PrivateKey []byte
|
|
Scopes []string
|
|
IDPOptions idp.Options
|
|
}
|
|
|
|
func ExistsIDP(ctx context.Context, filter preparation.FilterToQueryReducer, id, orgID string) (exists bool, err error) {
|
|
writeModel := NewOrgIDPRemoveWriteModel(orgID, id)
|
|
events, err := filter(ctx, writeModel.Query())
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
|
|
if len(events) > 0 {
|
|
writeModel.AppendEvents(events...)
|
|
if err := writeModel.Reduce(); err != nil {
|
|
return false, err
|
|
}
|
|
return writeModel.State.Exists(), nil
|
|
}
|
|
|
|
instanceWriteModel := NewInstanceIDPRemoveWriteModel(authz.GetInstance(ctx).InstanceID(), id)
|
|
events, err = filter(ctx, instanceWriteModel.Query())
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
|
|
if len(events) == 0 {
|
|
return false, nil
|
|
}
|
|
instanceWriteModel.AppendEvents(events...)
|
|
if err := instanceWriteModel.Reduce(); err != nil {
|
|
return false, err
|
|
}
|
|
return instanceWriteModel.State.Exists(), nil
|
|
}
|
|
|
|
func IDPProviderWriteModel(ctx context.Context, filter preparation.FilterToQueryReducer, id string) (_ *AllIDPWriteModel, err error) {
|
|
writeModel := NewIDPTypeWriteModel(id)
|
|
events, err := filter(ctx, writeModel.Query())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errors.ThrowPreconditionFailed(nil, "COMMAND-as02jin", "Errors.IDPConfig.NotExisting")
|
|
}
|
|
writeModel.AppendEvents(events...)
|
|
if err := writeModel.Reduce(); err != nil {
|
|
return nil, err
|
|
}
|
|
allWriteModel, err := NewAllIDPWriteModel(
|
|
writeModel.ResourceOwner,
|
|
writeModel.ResourceOwner == writeModel.InstanceID,
|
|
writeModel.ID,
|
|
writeModel.Type,
|
|
)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
events, err = filter(ctx, allWriteModel.Query())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
allWriteModel.AppendEvents(events...)
|
|
if err := allWriteModel.Reduce(); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return allWriteModel, err
|
|
}
|