mirror of
https://github.com/zitadel/zitadel.git
synced 2025-04-24 12:11:31 +00:00
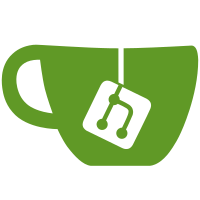
# Which Problems Are Solved The recently introduced notification queue have potential race conditions. # How the Problems Are Solved Current code is refactored to use the queue package, which is safe in regards of concurrency. # Additional Changes - the queue is included in startup - improved code quality of queue # Additional Context - closes https://github.com/zitadel/zitadel/issues/9278
39 lines
787 B
Go
39 lines
787 B
Go
package queue
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/jackc/pgx/v5"
|
|
"github.com/riverqueue/river/riverdriver"
|
|
"github.com/riverqueue/river/riverdriver/riverpgxv5"
|
|
"github.com/riverqueue/river/rivermigrate"
|
|
|
|
"github.com/zitadel/zitadel/internal/database"
|
|
)
|
|
|
|
type Migrator struct {
|
|
driver riverdriver.Driver[pgx.Tx]
|
|
}
|
|
|
|
func NewMigrator(client *database.DB) *Migrator {
|
|
return &Migrator{
|
|
driver: riverpgxv5.New(client.Pool),
|
|
}
|
|
}
|
|
|
|
func (m *Migrator) Execute(ctx context.Context) error {
|
|
_, err := m.driver.GetExecutor().Exec(ctx, "CREATE SCHEMA IF NOT EXISTS "+schema)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
migrator, err := rivermigrate.New(m.driver, nil)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
ctx = WithQueue(ctx)
|
|
_, err = migrator.Migrate(ctx, rivermigrate.DirectionUp, nil)
|
|
return err
|
|
|
|
}
|