mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
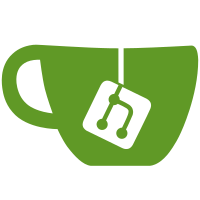
* fix: correct env var for tracing type * fix: local env tracing * fix: key in detail as string * fix: implement storage * fix: machine key by id fix: store public key as bytes instead of crypto value * update oidc pkg * dont check origins for service account tokens * fix: scopes * fix: dependencies * fix: dependencies * fix: remove unused code * fix: variable naming Co-authored-by: Livio Amstutz <livio.a@gmail.com>
55 lines
1012 B
Go
55 lines
1012 B
Go
package model
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/caos/zitadel/internal/model"
|
|
)
|
|
|
|
type MachineKeyView struct {
|
|
ID string
|
|
UserID string
|
|
Type MachineKeyType
|
|
Sequence uint64
|
|
CreationDate time.Time
|
|
ExpirationDate time.Time
|
|
PublicKey []byte
|
|
}
|
|
|
|
type MachineKeySearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
SortingColumn MachineKeySearchKey
|
|
Asc bool
|
|
Queries []*MachineKeySearchQuery
|
|
}
|
|
|
|
type MachineKeySearchKey int32
|
|
|
|
const (
|
|
MachineKeyKeyUnspecified MachineKeySearchKey = iota
|
|
MachineKeyKeyID
|
|
MachineKeyKeyUserID
|
|
)
|
|
|
|
type MachineKeySearchQuery struct {
|
|
Key MachineKeySearchKey
|
|
Method model.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type MachineKeySearchResponse struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
TotalResult uint64
|
|
Result []*MachineKeyView
|
|
Sequence uint64
|
|
Timestamp time.Time
|
|
}
|
|
|
|
func (r *MachineKeySearchRequest) EnsureLimit(limit uint64) {
|
|
if r.Limit == 0 || r.Limit > limit {
|
|
r.Limit = limit
|
|
}
|
|
}
|