mirror of
https://github.com/zitadel/zitadel.git
synced 2025-01-11 20:43:39 +00:00
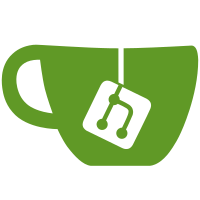
# Which Problems Are Solved - Adds infrastructure code (basic implementation, error handling, middlewares, ...) to implement the SCIM v2 interface - Adds support for the user create SCIM v2 endpoint # How the Problems Are Solved - Adds support for the user create SCIM v2 endpoint under `POST /scim/v2/{orgID}/Users` # Additional Context Part of #8140
61 lines
1.8 KiB
Go
61 lines
1.8 KiB
Go
package metadata
|
|
|
|
import (
|
|
"context"
|
|
"strings"
|
|
)
|
|
|
|
type Key string
|
|
type ScopedKey string
|
|
|
|
const (
|
|
externalIdProvisioningDomainPlaceholder = "{provisioningDomain}"
|
|
|
|
KeyPrefix = "urn:zitadel:scim:"
|
|
KeyProvisioningDomain Key = KeyPrefix + "provisioning_domain"
|
|
|
|
KeyExternalId Key = KeyPrefix + "externalId"
|
|
keyScopedExternalIdTemplate = KeyPrefix + externalIdProvisioningDomainPlaceholder + ":externalId"
|
|
KeyMiddleName Key = KeyPrefix + "name.middleName"
|
|
KeyHonorificPrefix Key = KeyPrefix + "name.honorificPrefix"
|
|
KeyHonorificSuffix Key = KeyPrefix + "name.honorificSuffix"
|
|
KeyProfileUrl Key = KeyPrefix + "profileURL"
|
|
KeyTitle Key = KeyPrefix + "title"
|
|
KeyLocale Key = KeyPrefix + "locale"
|
|
KeyTimezone Key = KeyPrefix + "timezone"
|
|
KeyIms Key = KeyPrefix + "ims"
|
|
KeyPhotos Key = KeyPrefix + "photos"
|
|
KeyAddresses Key = KeyPrefix + "addresses"
|
|
KeyEntitlements Key = KeyPrefix + "entitlements"
|
|
KeyRoles Key = KeyPrefix + "roles"
|
|
)
|
|
|
|
var ScimUserRelevantMetadataKeys = []Key{
|
|
KeyExternalId,
|
|
KeyMiddleName,
|
|
KeyHonorificPrefix,
|
|
KeyHonorificSuffix,
|
|
KeyProfileUrl,
|
|
KeyTitle,
|
|
KeyLocale,
|
|
KeyTimezone,
|
|
KeyIms,
|
|
KeyPhotos,
|
|
KeyAddresses,
|
|
KeyEntitlements,
|
|
KeyRoles,
|
|
}
|
|
|
|
func ScopeExternalIdKey(provisioningDomain string) ScopedKey {
|
|
return ScopedKey(strings.Replace(keyScopedExternalIdTemplate, externalIdProvisioningDomainPlaceholder, provisioningDomain, 1))
|
|
}
|
|
|
|
func ScopeKey(ctx context.Context, key Key) ScopedKey {
|
|
// only the externalID is scoped
|
|
if key == KeyExternalId {
|
|
return GetScimContextData(ctx).ExternalIDScopedMetadataKey
|
|
}
|
|
|
|
return ScopedKey(key)
|
|
}
|