mirror of
https://github.com/zitadel/zitadel.git
synced 2025-04-07 07:54:32 +00:00
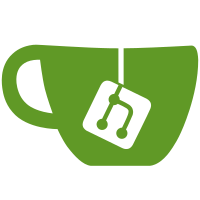
# Which Problems Are Solved When using a custom / new login UI and an OIDC application with registered BackChannelLogoutUI, no logout requests were sent to the URI when the user signed out. Additionally, as described in #9427, an error was logged: `level=error msg="event of type *session.TerminateEvent doesn't implement OriginEvent" caller="/home/runner/work/zitadel/zitadel/internal/notification/handlers/origin.go:24"` # How the Problems Are Solved - Properly pass `TriggerOrigin` information to session.TerminateEvent creation and implement `OriginEvent` interface. - Implemented `RegisterLogout` in `CreateOIDCSessionFromAuthRequest` and `CreateOIDCSessionFromDeviceAuth`, both used when interacting with the OIDC v2 API. - Both functions now receive the `BackChannelLogoutURI` of the client from the OIDC layer. # Additional Changes None # Additional Context - closes #9427 (cherry picked from commit ed697bbd69b7e9596e9cd53d8f37aad09403d87a)
51 lines
1.8 KiB
Go
51 lines
1.8 KiB
Go
package oidc
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
|
|
"github.com/zitadel/oidc/v3/pkg/oidc"
|
|
"github.com/zitadel/oidc/v3/pkg/op"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/command"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/telemetry/tracing"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
func (s *Server) DeviceToken(ctx context.Context, r *op.ClientRequest[oidc.DeviceAccessTokenRequest]) (_ *op.Response, err error) {
|
|
ctx, span := tracing.NewSpan(ctx)
|
|
defer func() {
|
|
span.EndWithError(err)
|
|
err = oidcError(err)
|
|
}()
|
|
|
|
client, ok := r.Client.(*Client)
|
|
if !ok {
|
|
return nil, zerrors.ThrowInternal(nil, "OIDC-Ae2ph", "Error.Internal")
|
|
}
|
|
session, err := s.command.CreateOIDCSessionFromDeviceAuth(ctx, r.Data.DeviceCode, client.client.BackChannelLogoutURI)
|
|
if err == nil {
|
|
return response(s.accessTokenResponseFromSession(ctx, client, session, "", client.client.ProjectID, client.client.ProjectRoleAssertion, client.client.AccessTokenRoleAssertion, client.client.IDTokenRoleAssertion, client.client.IDTokenUserinfoAssertion))
|
|
}
|
|
if errors.Is(err, context.DeadlineExceeded) {
|
|
return nil, oidc.ErrSlowDown().WithParent(err).WithReturnParentToClient(authz.GetFeatures(ctx).DebugOIDCParentError)
|
|
}
|
|
|
|
var target command.DeviceAuthStateError
|
|
if errors.As(err, &target) {
|
|
state := domain.DeviceAuthState(target)
|
|
if state == domain.DeviceAuthStateInitiated {
|
|
return nil, oidc.ErrAuthorizationPending()
|
|
}
|
|
if state == domain.DeviceAuthStateExpired {
|
|
return nil, oidc.ErrExpiredDeviceCode()
|
|
}
|
|
if state == domain.DeviceAuthStateDenied {
|
|
return nil, oidc.ErrAccessDenied()
|
|
}
|
|
}
|
|
return nil, oidc.ErrInvalidGrant().WithParent(err).WithReturnParentToClient(authz.GetFeatures(ctx).DebugOIDCParentError)
|
|
}
|