mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
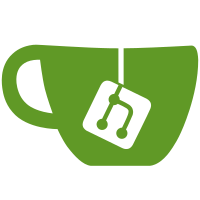
* feat: first implementation for saml sp * fix: add command side instance and org for saml provider * fix: add query side instance and org for saml provider * fix: request handling in event and retrieval of finished intent * fix: add review changes and integration tests * fix: add integration tests for saml idp * fix: correct unit tests with review changes * fix: add saml session unit test * fix: add saml session unit test * fix: add saml session unit test * fix: changes from review * fix: changes from review * fix: proto build error * fix: proto build error * fix: proto build error * fix: proto require metadata oneof * fix: login with saml provider * fix: integration test for saml assertion * lint client.go * fix json tag * fix: linting * fix import * fix: linting * fix saml idp query * fix: linting * lint: try all issues * revert linting config * fix: add regenerate endpoints * fix: translations * fix mk.yaml * ignore acs path for user agent cookie * fix: add AuthFromProvider test for saml * fix: integration test for saml retrieve information --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
227 lines
5.3 KiB
Go
227 lines
5.3 KiB
Go
package jwt
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"testing"
|
|
|
|
"github.com/golang/mock/gomock"
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/idp"
|
|
)
|
|
|
|
func TestProvider_BeginAuth(t *testing.T) {
|
|
type fields struct {
|
|
name string
|
|
issuer string
|
|
jwtEndpoint string
|
|
keysEndpoint string
|
|
headerName string
|
|
encryptionAlg func(t *testing.T) crypto.EncryptionAlgorithm
|
|
}
|
|
type args struct {
|
|
params []any
|
|
}
|
|
type want struct {
|
|
session idp.Session
|
|
err func(error) bool
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
args args
|
|
want want
|
|
}{
|
|
{
|
|
name: "missing userAgentID error",
|
|
fields: fields{
|
|
issuer: "https://jwt.com",
|
|
jwtEndpoint: "https://auth.com/jwt",
|
|
keysEndpoint: "https://jwt.com/keys",
|
|
headerName: "jwt-header",
|
|
encryptionAlg: func(t *testing.T) crypto.EncryptionAlgorithm {
|
|
return crypto.CreateMockEncryptionAlg(gomock.NewController(t))
|
|
},
|
|
},
|
|
args: args{
|
|
params: nil,
|
|
},
|
|
want: want{
|
|
err: func(err error) bool {
|
|
return errors.Is(err, ErrMissingUserAgentID)
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "invalid userAgentID error",
|
|
fields: fields{
|
|
issuer: "https://jwt.com",
|
|
jwtEndpoint: "https://auth.com/jwt",
|
|
keysEndpoint: "https://jwt.com/keys",
|
|
headerName: "jwt-header",
|
|
encryptionAlg: func(t *testing.T) crypto.EncryptionAlgorithm {
|
|
return crypto.CreateMockEncryptionAlg(gomock.NewController(t))
|
|
},
|
|
},
|
|
args: args{
|
|
params: []any{
|
|
0,
|
|
},
|
|
},
|
|
want: want{
|
|
err: func(err error) bool {
|
|
return errors.Is(err, ErrMissingUserAgentID)
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "successful auth",
|
|
fields: fields{
|
|
issuer: "https://jwt.com",
|
|
jwtEndpoint: "https://auth.com/jwt",
|
|
keysEndpoint: "https://jwt.com/keys",
|
|
headerName: "jwt-header",
|
|
encryptionAlg: func(t *testing.T) crypto.EncryptionAlgorithm {
|
|
return crypto.CreateMockEncryptionAlg(gomock.NewController(t))
|
|
},
|
|
},
|
|
args: args{
|
|
params: []any{
|
|
"agent",
|
|
},
|
|
},
|
|
want: want{
|
|
session: &Session{AuthURL: "https://auth.com/jwt?authRequestID=testState&userAgentID=YWdlbnQ"},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
a := assert.New(t)
|
|
|
|
provider, err := New(
|
|
tt.fields.name,
|
|
tt.fields.issuer,
|
|
tt.fields.jwtEndpoint,
|
|
tt.fields.keysEndpoint,
|
|
tt.fields.headerName,
|
|
tt.fields.encryptionAlg(t),
|
|
)
|
|
require.NoError(t, err)
|
|
|
|
ctx := context.Background()
|
|
session, err := provider.BeginAuth(ctx, "testState", tt.args.params...)
|
|
if tt.want.err != nil && !tt.want.err(err) {
|
|
a.Fail("invalid error", err)
|
|
}
|
|
if tt.want.err == nil {
|
|
a.NoError(err)
|
|
wantHeaders, wantContent := tt.want.session.GetAuth(ctx)
|
|
gotHeaders, gotContent := session.GetAuth(ctx)
|
|
a.Equal(wantHeaders, gotHeaders)
|
|
a.Equal(wantContent, gotContent)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestProvider_Options(t *testing.T) {
|
|
type fields struct {
|
|
name string
|
|
issuer string
|
|
jwtEndpoint string
|
|
keysEndpoint string
|
|
headerName string
|
|
encryptionAlg func(t *testing.T) crypto.EncryptionAlgorithm
|
|
opts []ProviderOpts
|
|
}
|
|
type want struct {
|
|
name string
|
|
linkingAllowed bool
|
|
creationAllowed bool
|
|
autoCreation bool
|
|
autoUpdate bool
|
|
pkce bool
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
want want
|
|
}{
|
|
{
|
|
name: "default",
|
|
fields: fields{
|
|
name: "jwt",
|
|
issuer: "https://jwt.com",
|
|
jwtEndpoint: "https://auth.com/jwt",
|
|
keysEndpoint: "https://jwt.com/keys",
|
|
headerName: "jwt-header",
|
|
encryptionAlg: func(t *testing.T) crypto.EncryptionAlgorithm {
|
|
return crypto.CreateMockEncryptionAlg(gomock.NewController(t))
|
|
},
|
|
opts: nil,
|
|
},
|
|
want: want{
|
|
name: "jwt",
|
|
linkingAllowed: false,
|
|
creationAllowed: false,
|
|
autoCreation: false,
|
|
autoUpdate: false,
|
|
pkce: false,
|
|
},
|
|
},
|
|
{
|
|
name: "all true",
|
|
fields: fields{
|
|
name: "jwt",
|
|
issuer: "https://jwt.com",
|
|
jwtEndpoint: "https://auth.com/jwt",
|
|
keysEndpoint: "https://jwt.com/keys",
|
|
headerName: "jwt-header",
|
|
encryptionAlg: func(t *testing.T) crypto.EncryptionAlgorithm {
|
|
return crypto.CreateMockEncryptionAlg(gomock.NewController(t))
|
|
},
|
|
opts: []ProviderOpts{
|
|
WithLinkingAllowed(),
|
|
WithCreationAllowed(),
|
|
WithAutoCreation(),
|
|
WithAutoUpdate(),
|
|
},
|
|
},
|
|
want: want{
|
|
name: "jwt",
|
|
linkingAllowed: true,
|
|
creationAllowed: true,
|
|
autoCreation: true,
|
|
autoUpdate: true,
|
|
pkce: true,
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
a := assert.New(t)
|
|
|
|
provider, err := New(
|
|
tt.fields.name,
|
|
tt.fields.issuer,
|
|
tt.fields.jwtEndpoint,
|
|
tt.fields.keysEndpoint,
|
|
tt.fields.headerName,
|
|
tt.fields.encryptionAlg(t),
|
|
tt.fields.opts...,
|
|
)
|
|
require.NoError(t, err)
|
|
|
|
a.Equal(tt.want.name, provider.Name())
|
|
a.Equal(tt.want.linkingAllowed, provider.IsLinkingAllowed())
|
|
a.Equal(tt.want.creationAllowed, provider.IsCreationAllowed())
|
|
a.Equal(tt.want.autoCreation, provider.IsAutoCreation())
|
|
a.Equal(tt.want.autoUpdate, provider.IsAutoUpdate())
|
|
})
|
|
}
|
|
}
|