mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
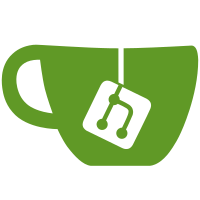
* feat: enable iframe use * cleanup * fix mocks * fix linting * docs: add iframe usage to solution scenarios configurations * improve api * feat(console): security policy * description * remove unnecessary line * disable input button and urls when not enabled * add image to docs Co-authored-by: Max Peintner <max@caos.ch> Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com>
106 lines
1.8 KiB
Go
106 lines
1.8 KiB
Go
package authz
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"golang.org/x/text/language"
|
|
)
|
|
|
|
func Test_Instance(t *testing.T) {
|
|
type args struct {
|
|
ctx context.Context
|
|
}
|
|
type res struct {
|
|
instanceID string
|
|
projectID string
|
|
consoleID string
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
res res
|
|
}{
|
|
{
|
|
"empty context",
|
|
args{
|
|
context.Background(),
|
|
},
|
|
res{
|
|
instanceID: "",
|
|
projectID: "",
|
|
consoleID: "",
|
|
},
|
|
},
|
|
{
|
|
"WithInstanceID",
|
|
args{
|
|
WithInstanceID(context.Background(), "id"),
|
|
},
|
|
res{
|
|
instanceID: "id",
|
|
projectID: "",
|
|
consoleID: "",
|
|
},
|
|
},
|
|
{
|
|
"WithInstance",
|
|
args{
|
|
WithInstance(context.Background(), &mockInstance{}),
|
|
},
|
|
res{
|
|
instanceID: "instanceID",
|
|
projectID: "projectID",
|
|
consoleID: "consoleID",
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got := GetInstance(tt.args.ctx)
|
|
assert.Equal(t, tt.res.instanceID, got.InstanceID())
|
|
assert.Equal(t, tt.res.projectID, got.ProjectID())
|
|
assert.Equal(t, tt.res.consoleID, got.ConsoleClientID())
|
|
})
|
|
}
|
|
}
|
|
|
|
type mockInstance struct{}
|
|
|
|
func (m *mockInstance) InstanceID() string {
|
|
return "instanceID"
|
|
}
|
|
|
|
func (m *mockInstance) ProjectID() string {
|
|
return "projectID"
|
|
}
|
|
|
|
func (m *mockInstance) ConsoleClientID() string {
|
|
return "consoleID"
|
|
}
|
|
|
|
func (m *mockInstance) ConsoleApplicationID() string {
|
|
return "appID"
|
|
}
|
|
|
|
func (m *mockInstance) DefaultLanguage() language.Tag {
|
|
return language.English
|
|
}
|
|
|
|
func (m *mockInstance) DefaultOrganisationID() string {
|
|
return "orgID"
|
|
}
|
|
|
|
func (m *mockInstance) RequestedDomain() string {
|
|
return "zitadel.cloud"
|
|
}
|
|
|
|
func (m *mockInstance) RequestedHost() string {
|
|
return "zitadel.cloud:443"
|
|
}
|
|
|
|
func (m *mockInstance) SecurityPolicyAllowedOrigins() []string {
|
|
return nil
|
|
}
|