mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
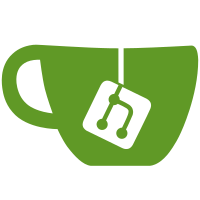
* feat: return 404 or 409 if org reg disallowed * fix: system limit permissions * feat: add iam limits api * feat: disallow public org registrations on default instance * add integration test * test: integration * fix test * docs: describe public org registrations * avoid updating docs deps * fix system limits integration test * silence integration tests * fix linting * ignore strange linter complaints * review * improve reset properties naming * redefine the api * use restrictions aggregate * test query * simplify and test projection * test commands * fix unit tests * move integration test * support restrictions on default instance * also test GetRestrictions * self review * lint * abstract away resource owner * fix tests * configure supported languages * fix allowed languages * fix tests * default lang must not be restricted * preferred language must be allowed * change preferred languages * check languages everywhere * lint * test command side * lint * add integration test * add integration test * restrict supported ui locales * lint * lint * cleanup * lint * allow undefined preferred language * fix integration tests * update main * fix env var * ignore linter * ignore linter * improve integration test config * reduce cognitive complexity * compile * check for duplicates * remove useless restriction checks * review * revert restriction renaming * fix language restrictions * lint * generate * allow custom texts for supported langs for now * fix tests * cleanup * cleanup * cleanup * lint * unsupported preferred lang is allowed * fix integration test * finish reverting to old property name * finish reverting to old property name * load languages * refactor(i18n): centralize translators and fs * lint * amplify no validations on preferred languages * fix integration test * lint * fix resetting allowed languages * test unchanged restrictions
121 lines
5.9 KiB
Go
121 lines
5.9 KiB
Go
package oidc
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/zitadel/oidc/v3/pkg/oidc"
|
|
"github.com/zitadel/oidc/v3/pkg/op"
|
|
"golang.org/x/text/language"
|
|
)
|
|
|
|
func TestServer_createDiscoveryConfig(t *testing.T) {
|
|
type fields struct {
|
|
LegacyServer *op.LegacyServer
|
|
signingKeyAlgorithm string
|
|
}
|
|
type args struct {
|
|
ctx context.Context
|
|
supportedUILocales []language.Tag
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
fields fields
|
|
args args
|
|
want *oidc.DiscoveryConfiguration
|
|
}{
|
|
{
|
|
"config",
|
|
fields{
|
|
LegacyServer: op.NewLegacyServer(
|
|
func() *op.Provider {
|
|
provider, _ := op.NewForwardedOpenIDProvider("path",
|
|
&op.Config{
|
|
CodeMethodS256: true,
|
|
AuthMethodPost: true,
|
|
AuthMethodPrivateKeyJWT: true,
|
|
GrantTypeRefreshToken: true,
|
|
RequestObjectSupported: true,
|
|
},
|
|
nil,
|
|
)
|
|
return provider
|
|
}(),
|
|
op.Endpoints{
|
|
Authorization: op.NewEndpoint("auth"),
|
|
Token: op.NewEndpoint("token"),
|
|
Introspection: op.NewEndpoint("introspect"),
|
|
Userinfo: op.NewEndpoint("userinfo"),
|
|
Revocation: op.NewEndpoint("revoke"),
|
|
EndSession: op.NewEndpoint("logout"),
|
|
JwksURI: op.NewEndpoint("keys"),
|
|
DeviceAuthorization: op.NewEndpoint("device"),
|
|
},
|
|
),
|
|
signingKeyAlgorithm: "RS256",
|
|
},
|
|
args{
|
|
ctx: op.ContextWithIssuer(context.Background(), "https://issuer.com"),
|
|
supportedUILocales: []language.Tag{language.English, language.German},
|
|
},
|
|
&oidc.DiscoveryConfiguration{
|
|
Issuer: "https://issuer.com",
|
|
AuthorizationEndpoint: "https://issuer.com/auth",
|
|
TokenEndpoint: "https://issuer.com/token",
|
|
IntrospectionEndpoint: "https://issuer.com/introspect",
|
|
UserinfoEndpoint: "https://issuer.com/userinfo",
|
|
RevocationEndpoint: "https://issuer.com/revoke",
|
|
EndSessionEndpoint: "https://issuer.com/logout",
|
|
DeviceAuthorizationEndpoint: "https://issuer.com/device",
|
|
CheckSessionIframe: "",
|
|
JwksURI: "https://issuer.com/keys",
|
|
RegistrationEndpoint: "",
|
|
ScopesSupported: []string{oidc.ScopeOpenID, oidc.ScopeProfile, oidc.ScopeEmail, oidc.ScopePhone, oidc.ScopeAddress, oidc.ScopeOfflineAccess},
|
|
ResponseTypesSupported: []string{string(oidc.ResponseTypeCode), string(oidc.ResponseTypeIDTokenOnly), string(oidc.ResponseTypeIDToken)},
|
|
ResponseModesSupported: nil,
|
|
GrantTypesSupported: []oidc.GrantType{oidc.GrantTypeCode, oidc.GrantTypeImplicit, oidc.GrantTypeRefreshToken, oidc.GrantTypeBearer},
|
|
ACRValuesSupported: nil,
|
|
SubjectTypesSupported: []string{"public"},
|
|
IDTokenSigningAlgValuesSupported: []string{"RS256"},
|
|
IDTokenEncryptionAlgValuesSupported: nil,
|
|
IDTokenEncryptionEncValuesSupported: nil,
|
|
UserinfoSigningAlgValuesSupported: nil,
|
|
UserinfoEncryptionAlgValuesSupported: nil,
|
|
UserinfoEncryptionEncValuesSupported: nil,
|
|
RequestObjectSigningAlgValuesSupported: []string{"RS256"},
|
|
RequestObjectEncryptionAlgValuesSupported: nil,
|
|
RequestObjectEncryptionEncValuesSupported: nil,
|
|
TokenEndpointAuthMethodsSupported: []oidc.AuthMethod{oidc.AuthMethodNone, oidc.AuthMethodBasic, oidc.AuthMethodPost, oidc.AuthMethodPrivateKeyJWT},
|
|
TokenEndpointAuthSigningAlgValuesSupported: []string{"RS256"},
|
|
RevocationEndpointAuthMethodsSupported: []oidc.AuthMethod{oidc.AuthMethodNone, oidc.AuthMethodBasic, oidc.AuthMethodPost, oidc.AuthMethodPrivateKeyJWT},
|
|
RevocationEndpointAuthSigningAlgValuesSupported: []string{"RS256"},
|
|
IntrospectionEndpointAuthMethodsSupported: []oidc.AuthMethod{oidc.AuthMethodBasic, oidc.AuthMethodPrivateKeyJWT},
|
|
IntrospectionEndpointAuthSigningAlgValuesSupported: []string{"RS256"},
|
|
DisplayValuesSupported: nil,
|
|
ClaimTypesSupported: nil,
|
|
ClaimsSupported: []string{"sub", "aud", "exp", "iat", "iss", "auth_time", "nonce", "acr", "amr", "c_hash", "at_hash", "act", "scopes", "client_id", "azp", "preferred_username", "name", "family_name", "given_name", "locale", "email", "email_verified", "phone_number", "phone_number_verified"},
|
|
ClaimsParameterSupported: false,
|
|
CodeChallengeMethodsSupported: []oidc.CodeChallengeMethod{"S256"},
|
|
ServiceDocumentation: "",
|
|
ClaimsLocalesSupported: nil,
|
|
UILocalesSupported: []language.Tag{language.English, language.German},
|
|
RequestParameterSupported: true,
|
|
RequestURIParameterSupported: false,
|
|
RequireRequestURIRegistration: false,
|
|
OPPolicyURI: "",
|
|
OPTermsOfServiceURI: "",
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
s := &Server{
|
|
LegacyServer: tt.fields.LegacyServer,
|
|
signingKeyAlgorithm: tt.fields.signingKeyAlgorithm,
|
|
}
|
|
assert.Equalf(t, tt.want, s.createDiscoveryConfig(tt.args.ctx, tt.args.supportedUILocales), "createDiscoveryConfig(%v)", tt.args.ctx)
|
|
})
|
|
}
|
|
}
|