mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
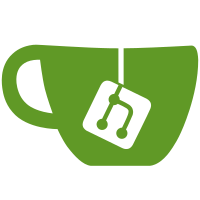
* fix: make user creation errors helpful * fix linting and unit testing errors * fix linting * make zitadel config reusable * fix human validations * translate ssr errors * make zitadel config reusable * cover more translations for ssr * handle email validation message centrally * fix unit tests * fix linting * align signatures * use more precise wording * handle phone validation message centrally * fix: return specific profile errors * docs: edit comments * fix unit tests --------- Co-authored-by: Silvan <silvan.reusser@gmail.com>
47 lines
1.5 KiB
Go
47 lines
1.5 KiB
Go
package admin
|
|
|
|
import (
|
|
"github.com/zitadel/logging"
|
|
"golang.org/x/text/language"
|
|
|
|
user_grpc "github.com/zitadel/zitadel/internal/api/grpc/user"
|
|
"github.com/zitadel/zitadel/internal/command"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
admin_grpc "github.com/zitadel/zitadel/pkg/grpc/admin"
|
|
)
|
|
|
|
func setUpOrgHumanToCommand(human *admin_grpc.SetUpOrgRequest_Human) *command.AddHuman {
|
|
var lang language.Tag
|
|
lang, err := language.Parse(human.Profile.PreferredLanguage)
|
|
logging.OnError(err).Debug("unable to parse language")
|
|
return &command.AddHuman{
|
|
Username: human.UserName,
|
|
FirstName: human.Profile.FirstName,
|
|
LastName: human.Profile.LastName,
|
|
NickName: human.Profile.NickName,
|
|
DisplayName: human.Profile.DisplayName,
|
|
PreferredLanguage: lang,
|
|
Gender: user_grpc.GenderToDomain(human.Profile.Gender),
|
|
Email: setUpOrgHumanEmailToDomain(human.Email),
|
|
Phone: setUpOrgHumanPhoneToDomain(human.Phone),
|
|
Password: human.Password,
|
|
}
|
|
}
|
|
|
|
func setUpOrgHumanEmailToDomain(email *admin_grpc.SetUpOrgRequest_Human_Email) command.Email {
|
|
return command.Email{
|
|
Address: domain.EmailAddress(email.Email),
|
|
Verified: email.IsEmailVerified,
|
|
}
|
|
}
|
|
|
|
func setUpOrgHumanPhoneToDomain(phone *admin_grpc.SetUpOrgRequest_Human_Phone) command.Phone {
|
|
if phone == nil {
|
|
return command.Phone{}
|
|
}
|
|
return command.Phone{
|
|
Number: domain.PhoneNumber(phone.Phone),
|
|
Verified: phone.IsPhoneVerified,
|
|
}
|
|
}
|