mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
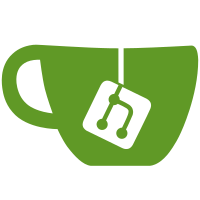
* feat(command): remove org * refactor: imports, unused code, error handling * reduce org removed in action * add org deletion to projections * add org removal to projections * add org removal to projections * org removed projection * lint import * projections * fix: table names in tests * fix: table names in tests * logging * add org state * fix(domain): add Owner removed to object details * feat(ListQuery): add with owner removed * fix(org-delete): add bool to functions to select with owner removed * fix(org-delete): add bools to user grants with events to determine if dependencies lost owner * fix(org-delete): add unit tests for owner removed and org removed events * fix(org-delete): add handling of org remove for grants and members * fix(org-delete): correction of unit tests for owner removed * fix(org-delete): update projections, unit tests and get functions * fix(org-delete): add change date to authnkeys and owner removed to org metadata * fix(org-delete): include owner removed for login names * fix(org-delete): some column fixes in projections and build for queries with owner removed * indexes * fix(org-delete): include review changes * fix(org-delete): change user projection name after merge * fix(org-delete): include review changes for project grant where no project owner is necessary * fix(org-delete): include auth and adminapi tables with owner removed information * fix(org-delete): cleanup username and orgdomain uniqueconstraints when org is removed * fix(org-delete): add permissions for org.remove * remove unnecessary unique constraints * fix column order in primary keys * fix(org-delete): include review changes * fix(org-delete): add owner removed indexes and chang setup step to create tables * fix(org-delete): move PK order of instance_id and change added user_grant from review * fix(org-delete): no params for prepareUserQuery * change to step 6 * merge main * fix(org-delete): OldUserName rename to private * fix linting * cleanup * fix: remove org test * create prerelease * chore: delete org-delete as prerelease Co-authored-by: Stefan Benz <stefan@caos.ch> Co-authored-by: Livio Spring <livio.a@gmail.com> Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com> Co-authored-by: Stefan Benz <46600784+stebenz@users.noreply.github.com>
74 lines
1.9 KiB
Go
74 lines
1.9 KiB
Go
package model
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
caos_errors "github.com/zitadel/zitadel/internal/errors"
|
|
)
|
|
|
|
type UserSessionView struct {
|
|
CreationDate time.Time
|
|
ChangeDate time.Time
|
|
State domain.UserSessionState
|
|
ResourceOwner string
|
|
UserAgentID string
|
|
UserID string
|
|
UserName string
|
|
LoginName string
|
|
DisplayName string
|
|
AvatarKey string
|
|
SelectedIDPConfigID string
|
|
PasswordVerification time.Time
|
|
PasswordlessVerification time.Time
|
|
ExternalLoginVerification time.Time
|
|
SecondFactorVerification time.Time
|
|
SecondFactorVerificationType domain.MFAType
|
|
MultiFactorVerification time.Time
|
|
MultiFactorVerificationType domain.MFAType
|
|
Sequence uint64
|
|
}
|
|
|
|
type UserSessionSearchRequest struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
SortingColumn UserSessionSearchKey
|
|
Asc bool
|
|
Queries []*UserSessionSearchQuery
|
|
}
|
|
|
|
type UserSessionSearchKey int32
|
|
|
|
const (
|
|
UserSessionSearchKeyUnspecified UserSessionSearchKey = iota
|
|
UserSessionSearchKeyUserAgentID
|
|
UserSessionSearchKeyUserID
|
|
UserSessionSearchKeyState
|
|
UserSessionSearchKeyResourceOwner
|
|
UserSessionSearchKeyInstanceID
|
|
UserSessionSearchKeyOwnerRemoved
|
|
)
|
|
|
|
type UserSessionSearchQuery struct {
|
|
Key UserSessionSearchKey
|
|
Method domain.SearchMethod
|
|
Value interface{}
|
|
}
|
|
|
|
type UserSessionSearchResponse struct {
|
|
Offset uint64
|
|
Limit uint64
|
|
TotalResult uint64
|
|
Result []*UserSessionView
|
|
}
|
|
|
|
func (r *UserSessionSearchRequest) EnsureLimit(limit uint64) error {
|
|
if r.Limit > limit {
|
|
return caos_errors.ThrowInvalidArgument(nil, "SEARCH-27ifs", "Errors.Limit.ExceedsDefault")
|
|
}
|
|
if r.Limit == 0 {
|
|
r.Limit = limit
|
|
}
|
|
return nil
|
|
}
|