mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
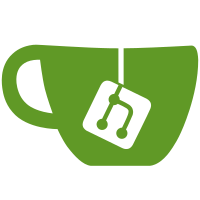
* feat(import): add functionality to import data into an instance * feat(import): move import to admin api and additional checks for nil pointer * fix(export): export implementation with filtered members and grants * fix: export and import implementation * fix: add possibility to export hashed passwords with the user * fix(import): import with structure of v1 and v2 * docs: add v1 proto * fix(import): check im imported user is already existing * fix(import): add otp import function * fix(import): add external idps, domains, custom text and messages * fix(import): correct usage of default values from login policy * fix(export): fix renaming of add project function * fix(import): move checks for unit tests * expect filter * fix(import): move checks for unit tests * fix(import): move checks for unit tests * fix(import): produce prerelease from branch * fix(import): correctly use provided user id for machine user imports * fix(import): corrected otp import and added guide for export and import * fix: import verified and primary domains * fix(import): add reading from gcs, s3 and localfile with tracing * fix(import): gcs and s3, file size correction and error logging * Delete docker-compose.yml * fix(import): progress logging and count of resources * fix(import): progress logging and count of resources * log subscription * fix(import): incorporate review * fix(import): incorporate review * docs: add suggestion for import Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com> * fix(import): add verification otp event and handling of deleted but existing users Co-authored-by: Livio Amstutz <livio.a@gmail.com> Co-authored-by: Fabienne <fabienne.gerschwiler@gmail.com> Co-authored-by: Silvan <silvan.reusser@gmail.com> Co-authored-by: Fabi <38692350+hifabienne@users.noreply.github.com>
113 lines
3.8 KiB
Go
113 lines
3.8 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
caos_errs "github.com/zitadel/zitadel/internal/errors"
|
|
"github.com/zitadel/zitadel/internal/repository/user"
|
|
"github.com/zitadel/zitadel/internal/telemetry/tracing"
|
|
)
|
|
|
|
func (c *Commands) AddMachine(ctx context.Context, orgID string, machine *domain.Machine) (*domain.Machine, error) {
|
|
if !machine.IsValid() {
|
|
return nil, caos_errs.ThrowInvalidArgument(nil, "COMMAND-bm9Ds", "Errors.User.Invalid")
|
|
}
|
|
domainPolicy, err := c.getOrgDomainPolicy(ctx, orgID)
|
|
if err != nil {
|
|
return nil, caos_errs.ThrowPreconditionFailed(err, "COMMAND-3M9fs", "Errors.Org.DomainPolicy.NotFound")
|
|
}
|
|
if !domainPolicy.UserLoginMustBeDomain {
|
|
return nil, caos_errs.ThrowPreconditionFailed(nil, "COMMAND-6M0ds", "Errors.User.Invalid")
|
|
}
|
|
userID, err := c.idGenerator.Next()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return c.addMachineWithID(ctx, orgID, userID, machine, domainPolicy)
|
|
}
|
|
|
|
func (c *Commands) AddMachineWithID(ctx context.Context, orgID string, userID string, machine *domain.Machine) (*domain.Machine, error) {
|
|
existingMachine, err := c.machineWriteModelByID(ctx, userID, orgID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if isUserStateExists(existingMachine.UserState) {
|
|
return nil, caos_errs.ThrowPreconditionFailed(nil, "COMMAND-k2una", "Errors.User.AlreadyExisting")
|
|
}
|
|
domainPolicy, err := c.getOrgDomainPolicy(ctx, orgID)
|
|
if err != nil {
|
|
return nil, caos_errs.ThrowPreconditionFailed(err, "COMMAND-3M9fs", "Errors.Org.DomainPolicy.NotFound")
|
|
}
|
|
if !domainPolicy.UserLoginMustBeDomain {
|
|
return nil, caos_errs.ThrowPreconditionFailed(nil, "COMMAND-6M0dd", "Errors.User.Invalid")
|
|
}
|
|
return c.addMachineWithID(ctx, orgID, userID, machine, domainPolicy)
|
|
}
|
|
|
|
func (c *Commands) addMachineWithID(ctx context.Context, orgID string, userID string, machine *domain.Machine, domainPolicy *domain.DomainPolicy) (*domain.Machine, error) {
|
|
|
|
machine.AggregateID = userID
|
|
addedMachine := NewMachineWriteModel(machine.AggregateID, orgID)
|
|
userAgg := UserAggregateFromWriteModel(&addedMachine.WriteModel)
|
|
events, err := c.eventstore.Push(ctx, user.NewMachineAddedEvent(
|
|
ctx,
|
|
userAgg,
|
|
machine.Username,
|
|
machine.Name,
|
|
machine.Description,
|
|
domainPolicy.UserLoginMustBeDomain,
|
|
))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
err = AppendAndReduce(addedMachine, events...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return writeModelToMachine(addedMachine), nil
|
|
}
|
|
|
|
func (c *Commands) ChangeMachine(ctx context.Context, machine *domain.Machine) (*domain.Machine, error) {
|
|
existingMachine, err := c.machineWriteModelByID(ctx, machine.AggregateID, machine.ResourceOwner)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if !isUserStateExists(existingMachine.UserState) {
|
|
return nil, caos_errs.ThrowNotFound(nil, "COMMAND-5M0od", "Errors.User.NotFound")
|
|
}
|
|
|
|
userAgg := UserAggregateFromWriteModel(&existingMachine.WriteModel)
|
|
changedEvent, hasChanged, err := existingMachine.NewChangedEvent(ctx, userAgg, machine.Name, machine.Description)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
if !hasChanged {
|
|
return nil, caos_errs.ThrowPreconditionFailed(nil, "COMMAND-2n8vs", "Errors.User.NotChanged")
|
|
}
|
|
|
|
events, err := c.eventstore.Push(ctx, changedEvent)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
err = AppendAndReduce(existingMachine, events...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return writeModelToMachine(existingMachine), nil
|
|
}
|
|
|
|
func (c *Commands) machineWriteModelByID(ctx context.Context, userID, resourceOwner string) (writeModel *MachineWriteModel, err error) {
|
|
if userID == "" {
|
|
return nil, caos_errs.ThrowInvalidArgument(nil, "COMMAND-0Plof", "Errors.User.UserIDMissing")
|
|
}
|
|
ctx, span := tracing.NewSpan(ctx)
|
|
defer func() { span.EndWithError(err) }()
|
|
|
|
writeModel = NewMachineWriteModel(userID, resourceOwner)
|
|
err = c.eventstore.FilterToQueryReducer(ctx, writeModel)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return writeModel, nil
|
|
}
|