mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 03:25:35 +00:00
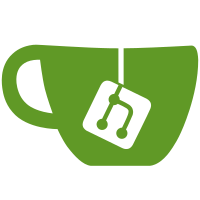
Add in UPnP portmapping, using goupnp library in order to get the UPnP client and run the portmapping functions. This rips out anywhere where UPnP used to be in portmapping, and has a flow separate from PMP and PCP. RELNOTE=portmapper now supports UPnP mappings Fixes #682 Updates #2109 Signed-off-by: julianknodt <julianknodt@gmail.com>
58 lines
1.4 KiB
Go
58 lines
1.4 KiB
Go
// Copyright (c) 2021 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package portmapper
|
|
|
|
import (
|
|
"context"
|
|
"os"
|
|
"strconv"
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func TestCreateOrGetMapping(t *testing.T) {
|
|
if v, _ := strconv.ParseBool(os.Getenv("HIT_NETWORK")); !v {
|
|
t.Skip("skipping test without HIT_NETWORK=1")
|
|
}
|
|
c := NewClient(t.Logf, nil)
|
|
defer c.Close()
|
|
c.SetLocalPort(1234)
|
|
for i := 0; i < 2; i++ {
|
|
if i > 0 {
|
|
time.Sleep(100 * time.Millisecond)
|
|
}
|
|
ext, err := c.createOrGetMapping(context.Background())
|
|
t.Logf("Got: %v, %v", ext, err)
|
|
}
|
|
}
|
|
|
|
func TestClientProbe(t *testing.T) {
|
|
if v, _ := strconv.ParseBool(os.Getenv("HIT_NETWORK")); !v {
|
|
t.Skip("skipping test without HIT_NETWORK=1")
|
|
}
|
|
c := NewClient(t.Logf, nil)
|
|
defer c.Close()
|
|
for i := 0; i < 3; i++ {
|
|
if i > 0 {
|
|
time.Sleep(100 * time.Millisecond)
|
|
}
|
|
res, err := c.Probe(context.Background())
|
|
t.Logf("Got(t=%dms): %+v, %v", i*100, res, err)
|
|
}
|
|
}
|
|
|
|
func TestClientProbeThenMap(t *testing.T) {
|
|
if v, _ := strconv.ParseBool(os.Getenv("HIT_NETWORK")); !v {
|
|
t.Skip("skipping test without HIT_NETWORK=1")
|
|
}
|
|
c := NewClient(t.Logf, nil)
|
|
defer c.Close()
|
|
c.SetLocalPort(1234)
|
|
res, err := c.Probe(context.Background())
|
|
t.Logf("Probe: %+v, %v", res, err)
|
|
ext, err := c.createOrGetMapping(context.Background())
|
|
t.Logf("createOrGetMapping: %v, %v", ext, err)
|
|
}
|