mirror of
https://github.com/zitadel/zitadel.git
synced 2025-01-07 16:47:41 +00:00
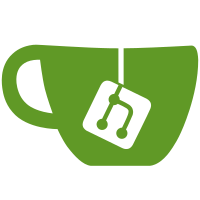
# Which Problems Are Solved To be able to migrate or test the new login UI, admins might want to (temporarily) switch individual apps. At a later point admin might want to make sure all applications use the new login UI. # How the Problems Are Solved - Added a feature flag `` on instance level to require all apps to use the new login and provide an optional base url. - if the flag is enabled, all (OIDC) applications will automatically use the v2 login. - if disabled, applications can decide based on their configuration - Added an option on OIDC apps to use the new login UI and an optional base url. - Removed the requirement to use `x-zitadel-login-client` to be redirected to the login V2 and retrieve created authrequest and link them to SSO sessions. - Added a new "IAM_LOGIN_CLIENT" role to allow management of users, sessions, grants and more without `x-zitadel-login-client`. # Additional Changes None # Additional Context closes https://github.com/zitadel/zitadel/issues/8702
76 lines
2.4 KiB
Go
76 lines
2.4 KiB
Go
package feature
|
|
|
|
import (
|
|
"net/url"
|
|
"slices"
|
|
)
|
|
|
|
//go:generate enumer -type Key -transform snake -trimprefix Key
|
|
type Key int
|
|
|
|
const (
|
|
KeyUnspecified Key = iota
|
|
KeyLoginDefaultOrg
|
|
KeyTriggerIntrospectionProjections
|
|
KeyLegacyIntrospection
|
|
KeyUserSchema
|
|
KeyTokenExchange
|
|
KeyActions
|
|
KeyImprovedPerformance
|
|
KeyWebKey
|
|
KeyDebugOIDCParentError
|
|
KeyOIDCSingleV1SessionTermination
|
|
KeyDisableUserTokenEvent
|
|
KeyEnableBackChannelLogout
|
|
KeyLoginV2
|
|
)
|
|
|
|
//go:generate enumer -type Level -transform snake -trimprefix Level
|
|
type Level int
|
|
|
|
const (
|
|
LevelUnspecified Level = iota
|
|
LevelSystem
|
|
LevelInstance
|
|
LevelOrg
|
|
LevelProject
|
|
LevelApp
|
|
LevelUser
|
|
)
|
|
|
|
type Features struct {
|
|
LoginDefaultOrg bool `json:"login_default_org,omitempty"`
|
|
TriggerIntrospectionProjections bool `json:"trigger_introspection_projections,omitempty"`
|
|
LegacyIntrospection bool `json:"legacy_introspection,omitempty"`
|
|
UserSchema bool `json:"user_schema,omitempty"`
|
|
TokenExchange bool `json:"token_exchange,omitempty"`
|
|
Actions bool `json:"actions,omitempty"`
|
|
ImprovedPerformance []ImprovedPerformanceType `json:"improved_performance,omitempty"`
|
|
WebKey bool `json:"web_key,omitempty"`
|
|
DebugOIDCParentError bool `json:"debug_oidc_parent_error,omitempty"`
|
|
OIDCSingleV1SessionTermination bool `json:"oidc_single_v1_session_termination,omitempty"`
|
|
DisableUserTokenEvent bool `json:"disable_user_token_event,omitempty"`
|
|
EnableBackChannelLogout bool `json:"enable_back_channel_logout,omitempty"`
|
|
LoginV2 LoginV2 `json:"login_v2,omitempty"`
|
|
}
|
|
|
|
type ImprovedPerformanceType int32
|
|
|
|
const (
|
|
ImprovedPerformanceTypeUnknown = iota
|
|
ImprovedPerformanceTypeOrgByID
|
|
ImprovedPerformanceTypeProjectGrant
|
|
ImprovedPerformanceTypeProject
|
|
ImprovedPerformanceTypeUserGrant
|
|
ImprovedPerformanceTypeOrgDomainVerified
|
|
)
|
|
|
|
func (f Features) ShouldUseImprovedPerformance(typ ImprovedPerformanceType) bool {
|
|
return slices.Contains(f.ImprovedPerformance, typ)
|
|
}
|
|
|
|
type LoginV2 struct {
|
|
Required bool `json:"required,omitempty"`
|
|
BaseURI *url.URL `json:"base_uri,omitempty"`
|
|
}
|